The equals method in Java is a method used to compare whether objects are equal. It is a method of the Object class and is very commonly used in actual development. However, due to insufficient understanding of the equals method and its implementation, many developers will make some common misunderstandings when using the equals method. This article will introduce the precautions and common misunderstandings about the equals method in Java to help readers better understand and use the equals method.
First, let us understand the basic usage of the equals method. In Java, all classes inherit from the Object class, and the equals method in the Object class is defined as follows:
public boolean equals(Object obj) { return (this == obj); }
As you can see, the default implementation of the equals method in the Object class is to compare whether the references of the objects are the same. That is, determine whether two objects are the same object. But in actual development, we usually need to judge whether they are equal based on the contents of the object, so we need to override the equals method in the custom class. The following is an example of overriding the equals method:
public class Person { private String name; private int age; // 省略构造方法和其他代码 @Override public boolean equals(Object obj) { if (this == obj) { return true; } if (obj == null || getClass() != obj.getClass()) { return false; } Person person = (Person) obj; return age == person.age && Objects.equals(name, person.name); } }
In this example, we override the equals method of the Person class to determine whether two Person objects are equal based on name and age.
Next, we will introduce some precautions and common misunderstandings about the equals method that need to be paid attention to in actual development.
- When rewriting the equals method, you need to follow the specifications
When you rewrite the equals method, you need to follow certain specifications. According to the official Java documentation, the equals method needs to meet the following conditions:
- Reflexivity: x.equals(x) must return true.
- Symmetry: If x.equals(y) returns true, then y.equals(x) must also return true.
- Transitiveness: If x.equals(y) returns true, and y.equals(z) also returns true, then x.equals(z) must also return true.
- Consistency: If x and y do not change, then calling x.equals(y) multiple times should return the same result.
- For any non-null reference x, x.equals(null) must return false.
When rewriting the equals method, you need to ensure that the above conditions are met to ensure the correctness of the equals method.
- Don’t forget to rewrite the hashCode method
While rewriting the equals method, you also need to rewrite the hashCode method. According to the official Java documentation, if two objects are judged to be equal according to the equals method, then their hashCode values must be equal. Therefore, when rewriting the equals method, be sure to rewrite the hashCode method at the same time to ensure that the object can be operated correctly when placed in a data structure such as a hash table. - Pay attention to handling null pointer exceptions
When overriding the equals method, you need to pay attention to handling null pointer exceptions. When using the equals method to compare objects, you need to first determine whether it is null to avoid the occurrence of a null pointer exception. - Use the equals method of the Objects class
When overriding the equals method, you can use the equals method of the Objects class to compare whether the properties of the objects are equal to avoid the occurrence of null pointer exceptions and type conversion exceptions. The equals method of the Objects class can correctly handle null values and type conversions, so it is recommended to use the equals method of the Objects class when overriding the equals method. - Don’t confuse the equals method with ==
When using the equals method, be sure to distinguish the difference between the equals method and the == operator. The equals method is used to compare whether the contents of objects are equal, and the == operator is used to compare whether the references of objects are equal. Therefore, when using the equals method, be sure to clearly understand the difference between the two.
To summarize, the equals method in Java is a method used to compare whether objects are equal, and is very commonly used in actual development. However, when using the equals method, you need to pay attention to the specifications and precautions for rewriting the equals method to avoid common misunderstandings. Correctly rewriting the equals method can improve the maintainability and robustness of the program, so I hope this article can help readers better understand and use the equals method.
The above is the detailed content of Common misunderstandings and precautions: equals(Object) method in Java. For more information, please follow other related articles on the PHP Chinese website!
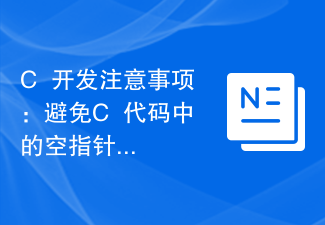
C++开发中,空指针异常是一种常见的错误,经常出现在指针没有被初始化或被释放后继续使用等情况下。空指针异常不仅会导致程序崩溃,还可能造成安全漏洞,因此需要特别注意。本文将介绍如何避免C++代码中的空指针异常。初始化指针变量C++中的指针必须在使用前进行初始化。如果没有初始化,指针将指向一个随机的内存地址,这可能导致空指针异常。要初始化指针,可以将其指向一个可
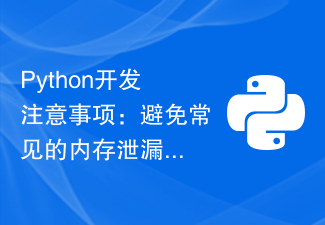
Python作为一种高级编程语言,具有易学易用和开发效率高等优点,在开发人员中越来越受欢迎。但是,由于其垃圾回收机制的实现方式,Python在处理大量内存时,容易出现内存泄漏问题。本文将从常见内存泄漏问题、引起问题的原因以及避免内存泄漏的方法三个方面来介绍Python开发过程中需要注意的事项。一、常见内存泄漏问题内存泄漏是指程序在运行中分配的内存空间无法释放
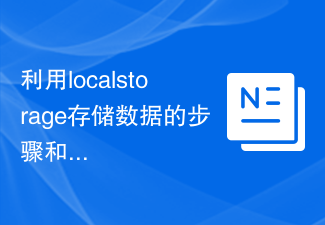
利用localStorage存储数据的步骤和注意事项本文主要介绍如何使用localStorage来存储数据,并提供相关的代码示例。LocalStorage是一种在浏览器中存储数据的方式,它可以将数据保存在用户的本地计算机上,而不需要通过服务器。下面是使用localStorage存储数据的步骤和需要注意的事项。步骤一:检测浏览器是否支持LocalStorage
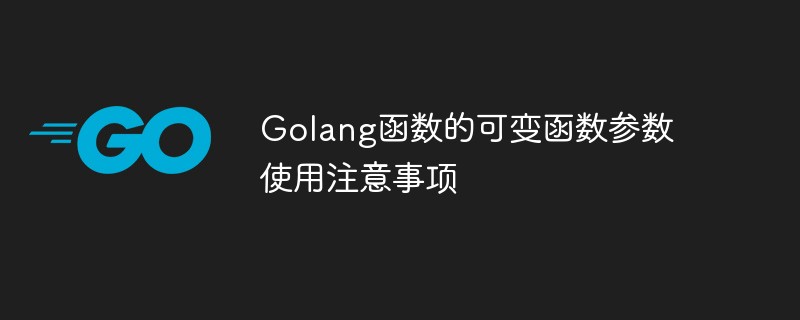
Golang是一种强类型、静态编程语言,其函数设计灵活,其中可变函数参数也是常见的实现方式之一,通常会用于函数参数个数不确定或者需要动态参数传递的场景。可变函数参数的使用虽然方便有效,但是也存在一些需要注意的问题,本文将详细介绍一下可变函数参数的使用注意事项。一、什么是可变函数参数?在Golang中,如果我们需要定义一个函数,但是无法确定该函数的参数个数,那
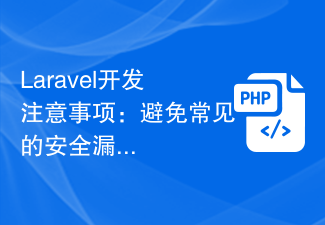
Laravel是一种广泛用于开发Web应用程序的PHP框架。它提供了许多方便易用的功能,以帮助开发者快速构建和维护应用程序。然而,与所有Web开发框架一样,Laravel也有一些可能导致安全漏洞的地方。在本文中,我们将重点介绍一些常见的安全漏洞,并提供一些注意事项,以帮助开发者避免这些问题。输入验证输入验证是防止用户提交恶意数据到应用程序的重要步骤。在Lar
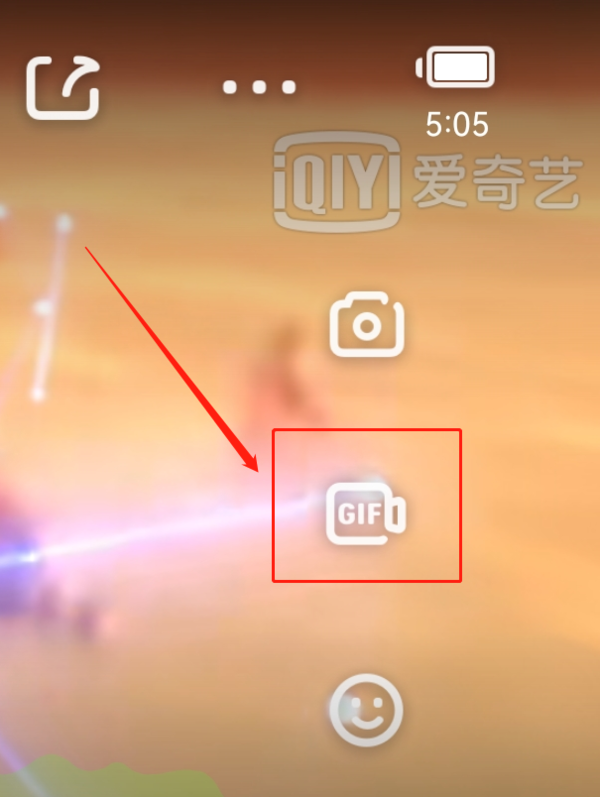
提到爱奇艺视频,大家都应该很熟悉。作为国内最受欢迎的视频播放软件之一,它是许多朋友观看剧集的必备工具。如果你在使用爱奇艺视频观看电影或电视剧时,看到一些有趣的片段,想要进行剪辑,该怎么办呢?接下来,我将为大家介绍一下在爱奇艺视频上如何剪辑视频,希望能对需要的朋友有所帮助在爱奇艺上如何进行视频剪辑?打开手机上的爱奇艺视频应用,并登录自己的账号。在登录后,找到要剪辑的视频并点击播放。进入视频播放界面后,点击屏幕,在左侧会出现选项图标。选择中间的视频截取图标,就会进入视频截取界面在视频截取界面,你可以
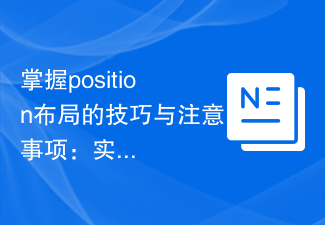
实现响应式布局:position布局的实践和注意事项概述:响应式布局是指根据用户的设备屏幕大小和分辨率自动调整网页内容的布局。在响应式布局中,position布局是常用的一种方法,它可以帮助我们实现不同屏幕尺寸下的元素定位和布局。一、position布局的基本原理position布局是基于CSS的定位属性,包括static、relative、absolute
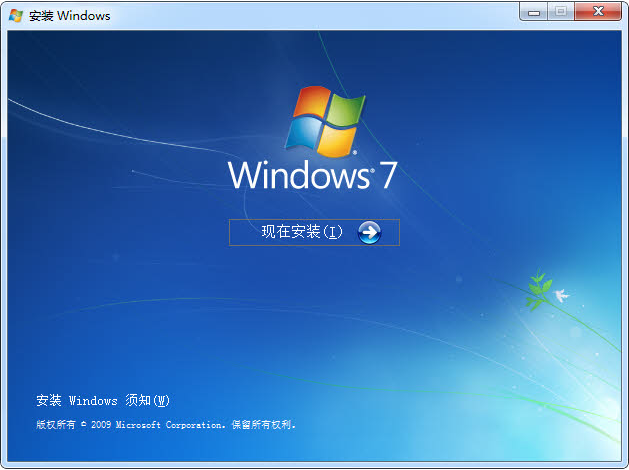
很多网友询问小编哪里可以下载到最安全的windows7iso镜像文件?网上搜索关于windows7iso镜像文件的资讯内容比较少,所以很多用户都不知道如何下载。今天小编给大家带来了win732以及win764位系统镜像文件的下载地址,大家快来看看吧。Windows7iso镜像系统硬件要求处理器:64位处理器;内存:最低1GB,是64位操作系统显卡:支持DirectX9128M显存:128MB硬盘空间:16G以上Windows7简体中文旗舰版x86ISO下载文件名:cn_windows_7_ult


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
