


Explore the difference and usage between prototype and prototype chain
In JavaScript, object-oriented programming is a commonly used programming method. Prototype and prototype chain are two important concepts when doing object-oriented programming. This article explores the differences between prototypes and prototype chains and how to use them, providing concrete code examples.
-
Basic concepts of prototype and prototype chain:
- The prototype (Prototype) is an attribute of the constructor (Constructor), which is an object. Every object has a prototype, which can be accessed through the
__proto__
attribute. - The prototype chain (Prototype Chain) is a chain structure connected by a series of objects through the
__proto__
attribute. When accessing a property of an object, if the object itself does not have the property, it will be looked up along the prototype chain.
- The prototype (Prototype) is an attribute of the constructor (Constructor), which is an object. Every object has a prototype, which can be accessed through the
-
The difference between prototype and prototype chain:
- The prototype is unique to each object and is used to inherit properties and methods. The prototype of an object can be obtained through
Object.getPrototypeOf(obj)
. - The prototype chain is an association between objects, which consists of the prototype of each object. Through the prototype chain, objects can share the properties and methods of the prototype.
- The prototype is unique to each object and is used to inherit properties and methods. The prototype of an object can be obtained through
-
Methods using prototypes and prototype chains:
-
Creating constructors and instance objects:
function Person(name) { this.name = name; } Person.prototype.sayHello = function() { console.log('Hello, ' + this.name); }; var person1 = new Person('Alice'); person1.sayHello(); // 输出:Hello, Alice
-
Inherit properties and methods:
function Student(name, grade) { Person.call(this, name); // 调用父类构造函数 this.grade = grade; } Student.prototype = Object.create(Person.prototype); // 继承父类原型 Student.prototype.constructor = Student; // 修复构造函数 Student.prototype.study = function() { console.log(this.name + ' is studying in grade ' + this.grade); }; var student1 = new Student('Bob', 5); student1.sayHello(); // 输出:Hello, Bob student1.study(); // 输出:Bob is studying in grade 5
-
Find properties and methods:
console.log(student1.name); // 输出:Bob console.log(student1.__proto__ === Student.prototype); // 输出:true console.log(student1.__proto__.__proto__ === Person.prototype); // 输出:true console.log(student1.__proto__.__proto__.__proto__ === Object.prototype); // 输出:true console.log(student1.hasOwnProperty('name')); // 输出:true console.log(student1.hasOwnProperty('sayHello')); // 输出:false
-
Through the above code With the example, we can clearly understand the role and use of prototypes and prototype chains. The prototype provides the ability for objects to inherit properties and methods, and the prototype chain realizes the sharing of properties and methods between objects. Using prototypes and prototype chains can improve code reusability while reducing memory consumption. However, in actual development, it should be noted that too long a prototype chain may cause performance problems, so the inheritance relationship of objects needs to be designed reasonably.
Summary:
In JavaScript, prototypes and prototype chains are important concepts in object-oriented programming. The prototype provides the ability to inherit properties and methods, and the prototype chain realizes the sharing of properties and methods between objects. By properly using prototypes and prototype chains, code reusability and performance can be improved. I hope this article will be helpful to the understanding and use of prototypes and prototype chains.
The above is the detailed content of Analyze the similarities, differences and application methods of prototypes and prototype chains. For more information, please follow other related articles on the PHP Chinese website!
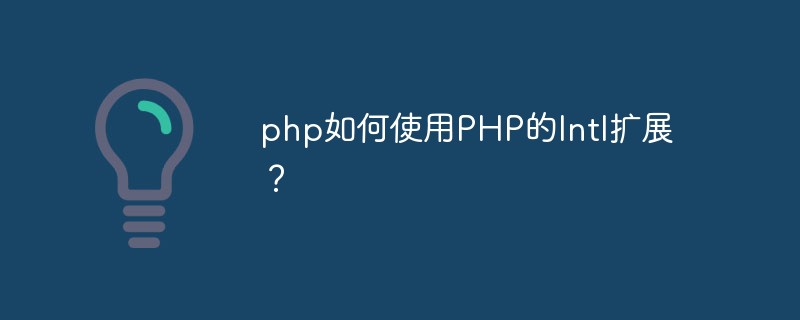
PHP的Intl扩展是一个非常实用的工具,它提供了一系列国际化和本地化的功能。本文将介绍如何使用PHP的Intl扩展。一、安装Intl扩展在开始使用Intl扩展之前,需要安装该扩展。在Windows下,可以在php.ini文件中打开该扩展。在Linux下,可以通过命令行安装:Ubuntu/Debian:sudoapt-getinstallphp7.4-
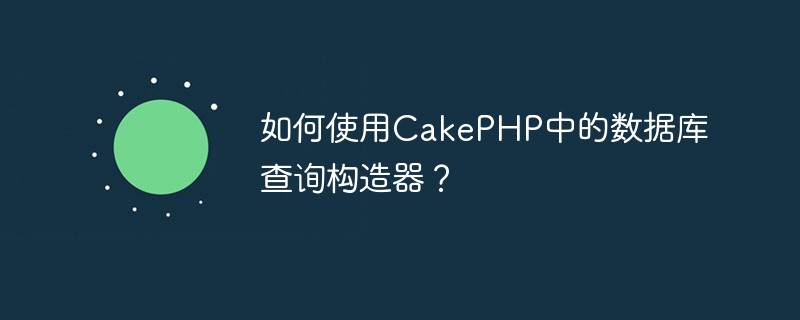
CakePHP是一个开源的PHPMVC框架,它广泛用于Web应用程序的开发。CakePHP具有许多功能和工具,其中包括一个强大的数据库查询构造器,用于交互性能数据库。该查询构造器允许您使用面向对象的语法执行SQL查询,而不必编写繁琐的SQL语句。本文将介绍如何使用CakePHP中的数据库查询构造器。建立数据库连接在使用数据库查询构造器之前,您首先需要在Ca

随着网络技术的发展,PHP已经成为了Web开发的重要工具之一。而其中一款流行的PHP框架——CodeIgniter(以下简称CI)也得到了越来越多的关注和使用。今天,我们就来看看如何使用CI框架。一、安装CI框架首先,我们需要下载CI框架并安装。在CI的官网(https://codeigniter.com/)上下载最新版本的CI框架压缩包。下载完成后,解压缩
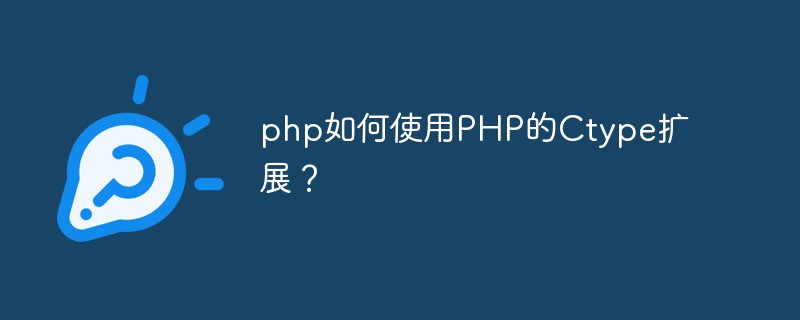
PHP是一种非常受欢迎的编程语言,它允许开发者创建各种各样的应用程序。但是,有时候在编写PHP代码时,我们需要处理和验证字符。这时候PHP的Ctype扩展就可以派上用场了。本文将就如何使用PHP的Ctype扩展展开介绍。什么是Ctype扩展?PHP的Ctype扩展是一个非常有用的工具,它提供了各种函数来验证字符串中的字符类型。这些函数包括isalnum、is
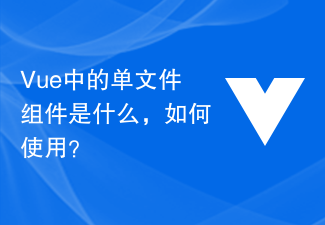
作为一种流行的前端框架,Vue能够提供开发者一个便捷高效的开发体验。其中,单文件组件是Vue的一个重要概念,使用它能够帮助开发者快速构建整洁、模块化的应用程序。在本文中,我们将介绍单文件组件是什么,以及如何在Vue中使用它们。一、单文件组件是什么?单文件组件(SingleFileComponent,简称SFC)是Vue中的一个重要概念,它
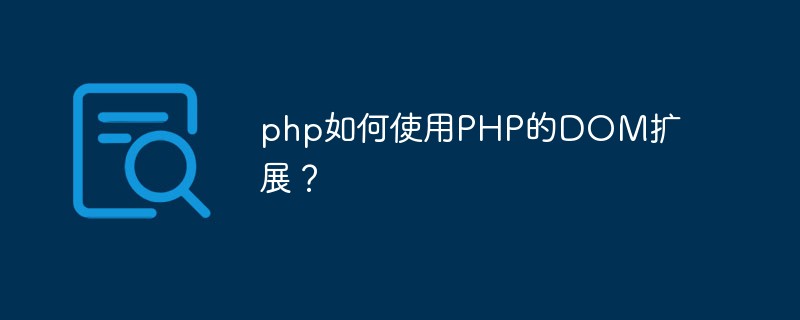
PHP的DOM扩展是一种基于文档对象模型(DOM)的PHP库,可以对XML文档进行创建、修改和查询操作。该扩展可以使PHP语言更加方便地处理XML文件,让开发者可以快速地实现对XML文件的数据分析和处理。本文将介绍如何使用PHP的DOM扩展。安装DOM扩展首先需要确保PHP已经安装了DOM扩展,如果没有安装需要先安装。在Linux系统中,可以使用以下命令来安
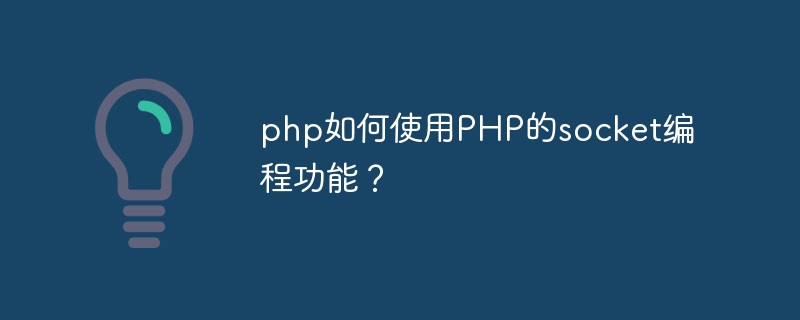
PHP是一门广泛应用于Web开发的编程语言,支持许多网络编程应用。其中,Socket编程是一种常用的实现网络通讯的方式,它能够让程序实现进程间的通讯,通过网络传输数据。本文将介绍如何在PHP中使用Socket编程功能。一、Socket编程简介Socket(套接字)是一种抽象的概念,在网络通信中代表了一个开放的端口,一个进程需要连接到该端口,才能与其它进程进行
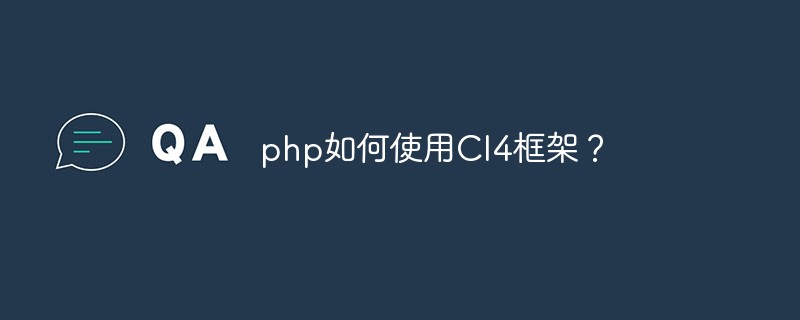
PHP是一种广泛使用的服务器端脚本语言,而CodeIgniter4(CI4)是一个流行的PHP框架,它提供了一种快速而优秀的方法来构建Web应用程序。在这篇文章中,我们将通过引导您了解如何使用CI4框架,来使您开始使用此框架来开发出众的Web应用程序。1.下载并安装CI4首先,您需要从官方网站(https://codeigniter.com/downloa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
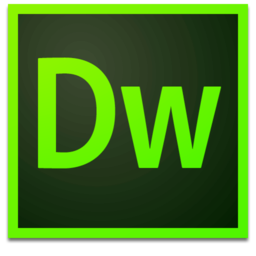
Dreamweaver Mac version
Visual web development tools
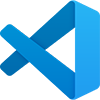
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
