Master efficient data crawling technology: Build a powerful Java crawler
Building a powerful Java crawler: Mastering these technologies to achieve efficient data crawling requires specific code examples
1. Introduction
With the rapid development of the Internet With the abundance of data resources, more and more application scenarios require crawling data from web pages. As a powerful programming language, Java has its own web crawler development framework and rich third-party libraries, making it an ideal choice. In this article, we will explain how to build a powerful web crawler using Java and provide concrete code examples.
2. Basic knowledge of web crawlers
- What is a web crawler?
A web crawler is an automated program that simulates human behavior of browsing web pages on the Internet and grabs the required data from web pages. The crawler will extract data from the web page according to certain rules and save it locally or process it further. - The working principle of the crawler
The working principle of the crawler can be roughly divided into the following steps: - Send an HTTP request to obtain the web page content.
- Parse the page and extract the required data.
- For storage or other further processing.
3. Java crawler development framework
Java has many development frameworks that can be used for the development of web crawlers. Two commonly used frameworks are introduced below.
- Jsoup
Jsoup is a Java library for parsing, traversing and manipulating HTML. It provides a flexible API and convenient selectors that make extracting data from HTML very simple. The following is a sample code using Jsoup for data extraction:
// 导入Jsoup库 import org.jsoup.Jsoup; import org.jsoup.nodes.Document; import org.jsoup.nodes.Element; import org.jsoup.select.Elements; public class JsoupExample { public static void main(String[] args) throws Exception { // 发送HTTP请求获取网页内容 Document doc = Jsoup.connect("http://example.com").get(); // 解析页面,提取需要的数据 Elements elements = doc.select("h1"); // 使用选择器选择需要的元素 for (Element element : elements) { System.out.println(element.text()); } } }
- HttpClient
HttpClient is a Java HTTP request library, which can easily simulate the browser sending HTTP requests. and get the response from the server. The following is a sample code that uses HttpClient to send HTTP requests:
// 导入HttpClient库 import org.apache.http.HttpEntity; import org.apache.http.HttpResponse; import org.apache.http.client.HttpClient; import org.apache.http.client.methods.HttpGet; import org.apache.http.impl.client.DefaultHttpClient; import org.apache.http.util.EntityUtils; public class HttpClientExample { public static void main(String[] args) throws Exception { // 创建HttpClient实例 HttpClient httpClient = new DefaultHttpClient(); // 创建HttpGet请求 HttpGet httpGet = new HttpGet("http://example.com"); // 发送HTTP请求并获取服务器的响应 HttpResponse response = httpClient.execute(httpGet); // 解析响应,提取需要的数据 HttpEntity entity = response.getEntity(); String content = EntityUtils.toString(entity); System.out.println(content); } }
4. Advanced technology
- Multi-threading
In order to improve the efficiency of the crawler, we can use Multi-threading to crawl multiple web pages at the same time. The following is a sample code for a crawler implemented using Java multi-threading:
import org.jsoup.Jsoup; import org.jsoup.nodes.Document; import org.jsoup.nodes.Element; import org.jsoup.select.Elements; import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class MultiThreadSpider { private static final int THREAD_POOL_SIZE = 10; public static void main(String[] args) throws Exception { ExecutorService executorService = Executors.newFixedThreadPool(THREAD_POOL_SIZE); for (int i = 1; i <= 10; i++) { final int page = i; executorService.execute(() -> { try { // 发送HTTP请求获取网页内容 Document doc = Jsoup.connect("http://example.com/page=" + page).get(); // 解析页面,提取需要的数据 Elements elements = doc.select("h1"); // 使用选择器选择需要的元素 for (Element element : elements) { System.out.println(element.text()); } } catch (Exception e) { e.printStackTrace(); } }); } executorService.shutdown(); } }
- Agent IP
In order to solve the problem of IP being banned by the server due to high crawling frequency, we can use Proxy IP to hide real IP address. The following is a sample code for a crawler using proxy IP:
import org.jsoup.Jsoup; import org.jsoup.nodes.Document; import org.jsoup.nodes.Element; import org.jsoup.select.Elements; import java.net.InetSocketAddress; import java.net.Proxy; public class ProxyIPSpider { public static void main(String[] args) throws Exception { // 创建代理IP Proxy proxy = new Proxy(Proxy.Type.HTTP, new InetSocketAddress("127.0.0.1", 8080)); // 发送HTTP请求并使用代理IP Document doc = Jsoup.connect("http://example.com").proxy(proxy).get(); // 解析页面,提取需要的数据 Elements elements = doc.select("h1"); // 使用选择器选择需要的元素 for (Element element : elements) { System.out.println(element.text()); } } }
5. Summary
In this article, we introduced how to use Java to build a powerful web crawler and provided specific code examples. . By learning these techniques, we can crawl the required data from web pages more efficiently. Of course, the use of web crawlers also requires compliance with relevant laws and ethics, reasonable use of crawler tools, and protection of privacy and the rights of others. I hope this article will help you learn and use Java crawlers!
The above is the detailed content of Master efficient data crawling technology: Build a powerful Java crawler. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
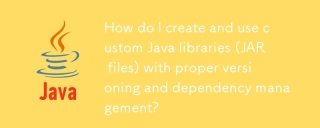
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
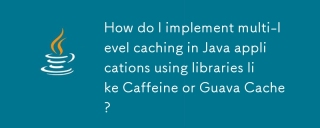
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
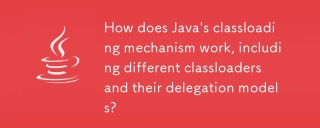
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
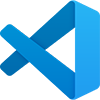
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
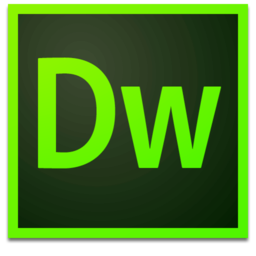
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor