


Detailed explanation of the differences and usage of variables and pointers in Go language
Detailed explanation of the difference between Go language variables and pointers and how to use them
Introduction:
Go language is a concise and efficient programming language with concurrency and garbage collection and other features, widely used in server-side development and system programming. In the process of learning and using the Go language, variables and pointers are very important concepts. This article will explain in detail the difference between variables and pointers in Go language and how to use them, and provide relevant code examples.
1. Variables:
A variable is a carrier for storing data, which can be of various types such as integer, floating point, Boolean, and string. In Go language, the syntax for declaring a variable is: var variable name type, for example:
var num int var name string
Variables can also be initialized by assignment:
var num int = 10 var name string = "Go"
Go language also provides a more concise method The variable declaration method:
num := 10 name := "Go"
The := composed of colon and equal sign is the short variable declaration syntax of Go language. The type of the variable can be automatically deduced according to the assignment situation, and the var keyword is omitted.
2. Pointer:
A pointer is a special variable that stores a memory address. In the Go language, the memory address of a variable can be obtained by using the & operator. For example:
var num int = 10 var p *int p = &num
In the above code, the variable p is a pointer to type int. The memory address of the num variable is obtained through the &p operator and assigned to p. Similarly, you can also use short variable declaration syntax to declare pointers:
num := 10 p := &num
Use pointers to indirectly access and modify the pointed variables. Use the * operator to obtain the value of the variable pointed to by the pointer, for example:
fmt.Println(*p) // 输出10
Using pointers can avoid copying a large amount of data, and is relatively efficient when passing parameters and return values. For example:
func modify(num *int) { *num = 20 } func main() { num := 10 modify(&num) fmt.Println(num) // 输出20 }
In the above code, the modify function uses a pointer parameter to modify the value of the variable pointed to by the incoming pointer to 20. Finally, the value of num is printed in the main function, and you can see that the value has been successfully modified.
3. The difference and usage of variables and pointers:
- Variables store real data values, while pointers store the memory address of the data value. Variables can directly access and manipulate data values, while pointers need to use the * operator to indirectly access and manipulate data values.
- Variables can be initialized directly when declared, but pointers need to be declared first and then assigned to the memory address of other variables.
- When variables are passed between functions, they are passed by value, and data will be copied. When pointers are passed between functions, they are passed by address, which can avoid copying a large amount of data.
- The scope of variables is local and exists inside the function. The scope of a pointer is global and can be shared between functions.
Summary:
This article explains in detail the difference and usage of variables and pointers in Go language, and uses sample code to help readers better understand and master these two concepts. When programming in the Go language, mastering the use of variables and pointers will help improve the efficiency and quality of the code.
Reference materials:
"Go Language Programming"
"Go Language Bible"
The above is the detailed content of Detailed explanation of the differences and usage of variables and pointers in Go language. For more information, please follow other related articles on the PHP Chinese website!
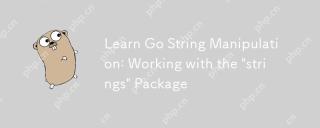
Go's "strings" package provides rich features to make string operation efficient and simple. 1) Use strings.Contains() to check substrings. 2) strings.Split() can be used to parse data, but it should be used with caution to avoid performance problems. 3) strings.Join() is suitable for formatting strings, but for small datasets, looping = is more efficient. 4) For large strings, it is more efficient to build strings using strings.Builder.
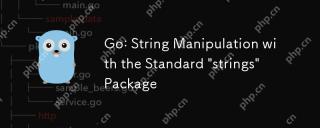
Go uses the "strings" package for string operations. 1) Use strings.Join function to splice strings. 2) Use the strings.Contains function to find substrings. 3) Use the strings.Replace function to replace strings. These functions are efficient and easy to use and are suitable for various string processing tasks.
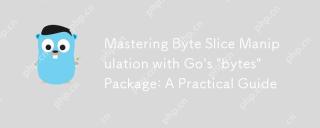
ThebytespackageinGoisessentialforefficientbyteslicemanipulation,offeringfunctionslikeContains,Index,andReplaceforsearchingandmodifyingbinarydata.Itenhancesperformanceandcodereadability,makingitavitaltoolforhandlingbinarydata,networkprotocols,andfileI
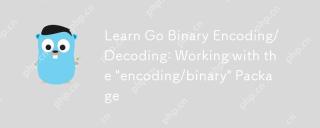
Go uses the "encoding/binary" package for binary encoding and decoding. 1) This package provides binary.Write and binary.Read functions for writing and reading data. 2) Pay attention to choosing the correct endian (such as BigEndian or LittleEndian). 3) Data alignment and error handling are also key to ensure the correctness and performance of the data.
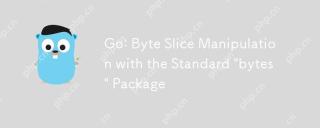
The"bytes"packageinGooffersefficientfunctionsformanipulatingbyteslices.1)Usebytes.Joinforconcatenatingslices,2)bytes.Bufferforincrementalwriting,3)bytes.Indexorbytes.IndexByteforsearching,4)bytes.Readerforreadinginchunks,and5)bytes.SplitNor
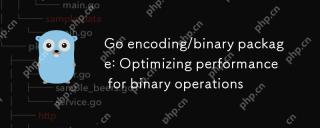
Theencoding/binarypackageinGoiseffectiveforoptimizingbinaryoperationsduetoitssupportforendiannessandefficientdatahandling.Toenhanceperformance:1)Usebinary.NativeEndianfornativeendiannesstoavoidbyteswapping.2)BatchReadandWriteoperationstoreduceI/Oover
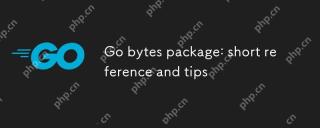
Go's bytes package is mainly used to efficiently process byte slices. 1) Using bytes.Buffer can efficiently perform string splicing to avoid unnecessary memory allocation. 2) The bytes.Equal function is used to quickly compare byte slices. 3) The bytes.Index, bytes.Split and bytes.ReplaceAll functions can be used to search and manipulate byte slices, but performance issues need to be paid attention to.
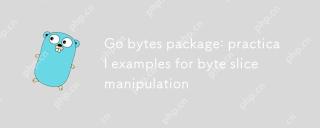
The byte package provides a variety of functions to efficiently process byte slices. 1) Use bytes.Contains to check the byte sequence. 2) Use bytes.Split to split byte slices. 3) Replace the byte sequence bytes.Replace. 4) Use bytes.Join to connect multiple byte slices. 5) Use bytes.Buffer to build data. 6) Combined bytes.Map for error processing and data verification.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 Linux new version
SublimeText3 Linux latest version
