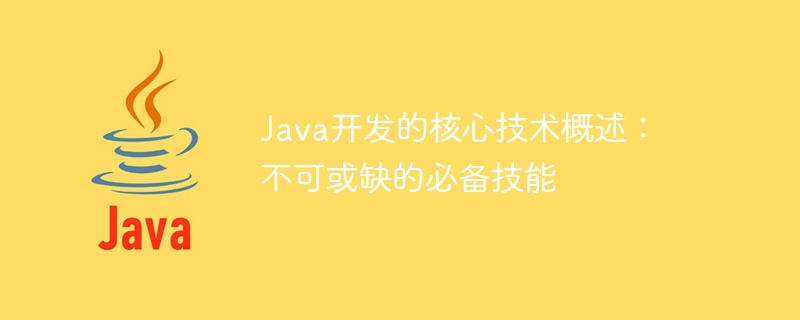
Overview of the core technologies of Java development: indispensable skills that require specific code examples
Introduction:
In today's software development In the industry, Java language is widely used in various fields. As a general-purpose, portable, object-oriented programming language, Java not only has a high degree of flexibility and stability, but also provides a wealth of development tools and powerful library support, allowing developers to build a variety of projects more quickly and efficiently. app. This article will outline the core technologies of Java development and provide some specific code examples to help readers better understand and master these technologies.
1. Java Language Basics
- Data types and variables: Java provides a variety of data types, including integers, floating point types, character types, Boolean types, etc. Developers You can choose the appropriate data type to store and manipulate data according to your needs. Variables are used to store and represent data. Variables can be created and used through declaration and assignment.
Code examples:
int age = 25;
float salary = 5000.50f;
char gender = 'M';
boolean isMarried = false;
- Control flow statements: Java provides a variety of control flow statements, including conditional statements (if-else, switch), loop statements (for , while, do-while) and jump statements (break, continue, return), which can realize program flow control and logical judgment.
Code sample:
int score = 85;
if (score >= 90) {
System.out.println("优秀");
} else if (score >= 80) {
System.out.println("良好");
} else if (score >= 60) {
System.out.println("及格");
} else {
System.out.println("不及格");
}
- Arrays and collections: In Java, arrays can be used to store a set of data of the same type, while collections are more flexible and can store Different types of data and provide rich operation methods. Developers can choose appropriate data structures to process data based on their needs.
Code example:
int[] numbers = {1, 2, 3, 4, 5};
System.out.println(numbers[0]); // 输出:1
List<String> names = new ArrayList<>();
names.add("张三");
names.add("李四");
System.out.println(names.get(1)); // 输出:李四
2. Object-oriented programming
- Classes and objects: Java is an object-oriented programming language that uses classes and The concept of objects is used to describe and structure programs. Classes are templates for object behavior and state, and objects are instances of classes. Developers can encapsulate and modularize programs by defining classes and creating objects.
Code sample:
class Person {
String name;
int age;
void sayHello() {
System.out.println("你好,我是" + name + ",今年" + age + "岁。");
}
}
Person person = new Person();
person.name = "张三";
person.age = 25;
person.sayHello(); // 输出:你好,我是张三,今年25岁。
- Inheritance and polymorphism: Inheritance is one of the important features of object-oriented programming, and code reuse and expansion can be achieved through inheritance. Polymorphism is a derived feature of inheritance, allowing different types of objects to implement different implementations of the same interface.
Code sample:
class Animal {
void eat() {
System.out.println("动物进食");
}
}
class Dog extends Animal {
@Override
void eat() {
System.out.println("狗进食");
}
}
class Cat extends Animal {
@Override
void eat() {
System.out.println("猫进食");
}
}
Animal dog = new Dog();
Animal cat = new Cat();
dog.eat(); // 输出:狗进食
cat.eat(); // 输出:猫进食
- Interface and abstract class: An interface is a specification that defines a set of methods to agree on the behavior of a class; abstract class It is an incomplete class used as a base class for other classes. Through interfaces and abstract classes, developers can define and maintain the overall structure of the program.
Code sample:
interface Shape {
double getArea();
}
class Circle implements Shape {
double radius;
Circle(double radius) {
this.radius = radius;
}
@Override
double getArea() {
return Math.PI * radius * radius;
}
}
Shape circle = new Circle(2.5);
System.out.println(circle.getArea()); // 输出:19.63
3. Common development frameworks and libraries
- Spring framework: Spring is a lightweight Java development framework. Provides a series of core modules, including IoC containers, AOP, MVC, etc., to simplify the development of enterprise-level applications. Developers can improve development efficiency and code quality by making reasonable use of the Spring framework.
- Hibernate framework: Hibernate is an open source object-relational mapping framework, which is used to establish a mapping relationship between object-oriented concepts and relational databases, simplifying the process of database operations. Developers can use the Hibernate framework to achieve fast and reliable interaction with the database.
- Apache Commons library: Apache Commons is a collection of open source Java class libraries that provides a variety of commonly used tool classes and components to simplify some common tasks in the Java development process, such as file processing, date and time processing, network communications, etc.
4. Summary
The core technologies of Java development include Java language foundation, object-oriented programming, common development frameworks and libraries, etc. Mastering these technologies is critical for developers to build applications more efficiently and flexibly. Through actual code examples, this article outlines the core technologies of Java development and hopes that readers can further consolidate and expand these skills through practice.
The above is the detailed content of Overview of key technologies for Java development: essential core skills. For more information, please follow other related articles on the PHP Chinese website!