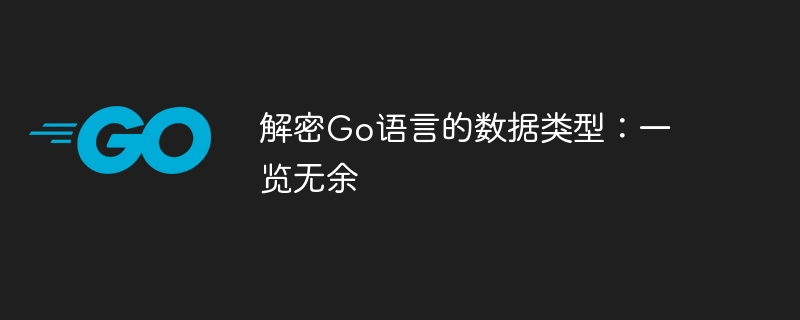
Decrypting the data types of Go language: a clear overview, specific code examples are needed
1. Introduction
Data types in programming languages are for developers Very importantly, it determines what kinds of data we can store and manipulate. In Go language, the concept of data types is similar to other programming languages, but Go language has its own unique data type system and characteristics. This article will give you an in-depth understanding of the data types of the Go language and decipher this family through specific code examples.
2. Basic data types
The basic data types of Go language include: Boolean, integer, floating point, complex number, string and character. They are bool, int, float, complex, string and rune. Let's take a look at them separately.
- Boolean type (bool)
The Boolean type has only two values, namely true and false. Like other languages, we can use Boolean types to judge and control the flow of the program.
Sample code:
package main
import "fmt"
func main() {
var b bool = true
fmt.Println(b)
}
- Integer type (int)
The integer data type can store integer values, and its size is related to the underlying computer architecture. In Go language, integers are divided into signed integers and unsigned integers. The following are some commonly used integer data types:
- int8: signed 8-bit integer, ranging from -128 to 127.
- int16: signed 16-bit integer, the value range is -32768 to 32767.
- int32: signed 32-bit integer, the value range is -2147483648 to 2147483647.
- int64: signed 64-bit integer, the value range is -9223372036854775808 to 9223372036854775807.
- uint8: Unsigned 8-bit integer, value range is 0 to 255.
- uint16: Unsigned 16-bit integer, value range is 0 to 65535.
- uint32: Unsigned 32-bit integer, the value range is 0 to 4294967295.
- uint64: Unsigned 64-bit integer, the value range is 0 to 18446744073709551615.
- int: Depending on the number of bits in the operating system and compiler, 32-bit operating systems are 32-bit integers, and 64-bit operating systems are 64-bit integers.
- uint: Depending on the number of bits in the operating system and compiler, 32-bit operating systems are 32-bit unsigned integers, and 64-bit operating systems are 64-bit unsigned integers.
Sample code:
package main
import "fmt"
func main() {
var i int = 10
fmt.Println(i)
}
- Floating point type (float)
The floating point data type can store decimal values and has two precisions: float32 and float64 .
- float32: Single-precision floating point number, accurate to 7 decimal places.
- float64: Double-precision floating point number, accurate to 15 digits after the decimal point.
Sample code:
package main
import "fmt"
func main() {
var f float32 = 3.14
fmt.Println(f)
}
- Complex number (complex)
The complex number type is used to represent complex numbers whose real and imaginary parts are both floating point types. In Go language, complex types have two precisions: complex64 and complex128.
- complex64: The real part and the imaginary part are represented by two float32.
- complex128: The real part and the imaginary part are represented by two float64.
Sample code:
package main
import "fmt"
func main() {
var c complex64 = complex(1, 2)
fmt.Println(c)
}
- String (string)
String is a data type in Go language, which is used to represent a string of characters. Strings are immutable, that is, they cannot be modified in the original string, but we can process strings through some functions and operations. In the Go language, strings are enclosed in double quotes (").
Sample code:
package main
import "fmt"
func main() {
var s string = "Hello, World!"
fmt.Println(s)
}
- Characters (rune)
Characters (rune ) is the data type used to represent Unicode characters in the Go language. It is actually an integer type that represents the code point of the Unicode character. Characters are represented by single quotes (').
Sample code:
package main
import "fmt"
func main() {
var r rune = '你'
fmt.Println(r)
}
3. Composite data types
In addition to basic data types, Go language also provides some composite data types, including arrays, slices, dictionaries, and structures. Structure (struct), interface (interface) and function (function).
- Array (array)
An array is a fixed-size data structure that can store a series of elements of the same type .In the Go language, the size of the array is fixed and cannot be changed.
Sample code:
package main
import "fmt"
func main() {
var arr [3]int
arr[0] = 1
arr[1] = 2
arr[2] = 3
fmt.Println(arr)
}
- Slice
Slice is a dynamic sized data structure, which can automatically expand as needed. Slices are created using the make function, or they can be created from arrays or other slices through the slice operator [:].
Sample code:
package main
import "fmt"
func main() {
arr := []int{1, 2, 3}
fmt.Println(arr)
}
- Dictionary (map)
A dictionary is an unordered collection consisting of keys and values. In the Go language, a dictionary is created using the make function.
Sample code:
package main
import "fmt"
func main() {
dict := make(map[string]int)
dict["apple"] = 1
dict["banana"] = 2
dict["cherry"] = 3
fmt.Println(dict)
}
- Structure (struct)
Structure is a custom data type that can contain multiple fields. Each field has its own type and name. In the Go language, structures are defined using the type keyword.
Sample code:
package main
import "fmt"
type Person struct {
Name string
Age int
}
func main() {
p := Person{"Alice", 18}
fmt.Println(p)
}
- Interface (interface)
Interface is an abstract data type , which defines a set of methods. The interface can be implemented by a specific type, and the method that implements the interface can be called through the interface type.
Sample code:
package main
import "fmt"
type Shape interface {
Area() float64
}
type Circle struct {
Radius float64
}
func (c Circle) Area() float64 {
return c.Radius * c.Radius * 3.14
}
func main() {
var s Shape
c := Circle{5}
s = c
fmt.Println(s.Area())
}
- 函数(function)
函数是一段可重复使用的代码块,它可以接受参数并返回结果。在Go语言中,函数是一等公民,可以像其他值类型一样被传递和赋值。
示例代码:
package main
import "fmt"
func Add(a, b int) int {
return a + b
}
func main() {
sum := Add(1, 2)
fmt.Println(sum)
}
总结
本文通过具体的代码示例对Go语言的数据类型进行了解密,详细介绍了Go语言的基本数据类型和复合数据类型。希望本文可以帮助大家更好地理解和应用Go语言的数据类型。
The above is the detailed content of Go language data types revealed: fully displayed. For more information, please follow other related articles on the PHP Chinese website!