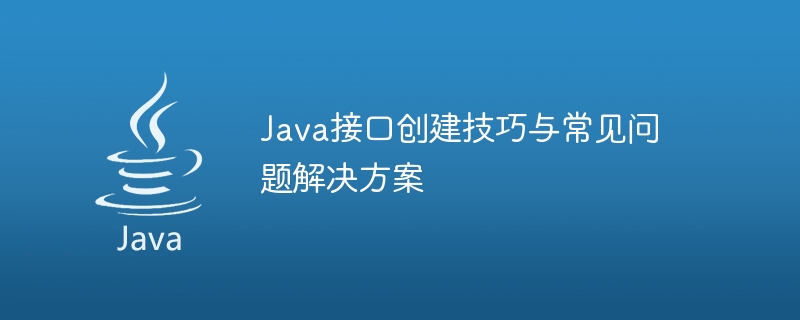
Java interface creation tips and solutions to common problems
Introduction:
Java interface is a pure abstract type that defines abstract methods and constants. It enables multiple inheritance and polymorphism in Java. This article will introduce some tips for creating interfaces and provide solutions to common problems, including implementing interfaces, using default methods, and common programming mistakes.
1. Tips for creating interfaces
- Name the interface: The name of the interface should be a noun or noun phrase, start with a capital letter, and use camel case nomenclature. For example, Runnable is a good interface name. Avoid using verbs as interface names because an interface represents a specification or contract, not a behavior.
- Define abstract methods: The main purpose of the interface is to define abstract methods. Method signatures should be clear and explain what the method does. Avoid defining too many methods in the interface to avoid it becoming complex and difficult to use. A good interface should be streamlined and highly reusable.
- Use default methods with caution: The default method is a specific implementation provided in the interface. New methods can be added to the interface without destroying the existing interface implementation. However, default methods should be used with great caution and only to ensure that they do not cause conflicts with existing code.
- Use the single responsibility principle: The interface should follow the single responsibility principle, that is, an interface should only define a single function or behavior. This makes the interface clearer, more organized, and easier to maintain.
2. Implementing the interface
Implementing the interface means that a class obtains the specific implementation of the abstract method defined in the interface by implementing one or more interfaces. In Java, a class can implement multiple interfaces.
- Steps to implement the interface:
public interface MyInterface {
void doSomething();
}
public class MyClass implements MyInterface {
// 实现接口中的方法
public void doSomething() {
// 具体实现逻辑
}
}
- Multiple implementations of the interface:
public interface MyInterface1 {
void doSomething1();
}
public interface MyInterface2 {
void doSomething2();
}
public class MyClass implements MyInterface1, MyInterface2 {
// 实现接口中的方法
public void doSomething1() {
// 具体实现逻辑
}
public void doSomething2() {
// 具体实现逻辑
}
}
3. Solutions to common problems
- Compilation error: Class does not implement all methods in the interface
When a class implements an interface, it needs to provide concrete implementations of all abstract methods in the interface. Make sure that the method signature in the class matches the method signature defined in the interface.
- Compatibility issues between classes
Interfaces provide a decoupled way to achieve polymorphism. Ensure that the behavior of the class is consistent with the contract of the interface, and compatibility between classes can be achieved through the interface.
- Interface extension issues
When the interface needs to add new methods, you can use the default method to add new methods to the interface to be compatible with existing implementation classes. However, care needs to be taken to avoid the misuse of default methods, which can lead to confusion and complexity in the code.
Summary:
This article introduces Java interface creation techniques and solutions to common problems, and provides specific code examples. Through the correct use of interfaces, code flexibility and extensibility can be achieved. I hope this article will be helpful to everyone's Java programming.
The above is the detailed content of Java interface creation tips and solutions to common problems. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn