


In-depth analysis of the functions and uses of NumPy functions
NumPy (Numerical Python) is an open source Python library for scientific computing. It provides efficient processing of arrays and comes with many convenient mathematical functions and tools. This article will provide an in-depth analysis of the functions and uses of some common functions in NumPy and provide specific code examples.
- Creating Arrays
NumPy provides a variety of methods to create arrays. These include using the array
function, arange
function and zeros
function, etc. Here are some examples of creating arrays:
import numpy as np # 使用array函数,将列表转换为数组 arr1 = np.array([1, 2, 3, 4, 5]) print(arr1) # 使用arange函数,创建一个从0到9的数组 arr2 = np.arange(10) print(arr2) # 使用zeros函数,创建一个元素全为0的3x3数组 arr3 = np.zeros((3, 3)) print(arr3)
- Array Operations
NumPy provides a number of functions for operations between arrays. These functions include addition, subtraction, multiplication, division, etc. The following are some examples of array operations:
import numpy as np # 加法 arr1 = np.array([1, 2, 3]) arr2 = np.array([4, 5, 6]) print(arr1 + arr2) # 减法 arr3 = np.array([7, 8, 9]) print(arr2 - arr3) # 乘法 print(arr1 * arr2) # 除法 print(arr2 / arr3)
- Array statistics
NumPy provides a rich set of statistical functions for calculating various statistical indicators of arrays. These functions include sum, mean, standard deviation, maximum, etc. Here are some examples of statistical functions:
import numpy as np arr = np.array([1, 2, 3, 4, 5]) # 求和 print(np.sum(arr)) # 平均值 print(np.mean(arr)) # 标准差 print(np.std(arr)) # 最大值 print(np.max(arr))
- Array Slicing
NumPy allows slicing operations on arrays to obtain parts or subsets of the array. Slicing operations use a colon (:) to specify a range. Here are some examples of array slicing:
import numpy as np arr = np.array([1, 2, 3, 4, 5]) # 获取数组的前三个元素 print(arr[:3]) # 获取数组的第三个到最后一个元素 print(arr[2:]) # 获取数组的第二个和第四个元素 print(arr[1:4:2])
- Multidimensional array operations
NumPy can create and manipulate multidimensional arrays. Multidimensional arrays can be two-dimensional, three-dimensional or even higher-dimensional. Here are some examples of multi-dimensional array operations:
import numpy as np # 创建一个3x3的二维数组 arr1 = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]]) print(arr1) # 计算二维数组的行和列的和 print(np.sum(arr1, axis=0)) # 列和 print(np.sum(arr1, axis=1)) # 行和 # 创建一个3x3x3的三维数组 arr2 = np.array([[[1, 2, 3], [4, 5, 6], [7, 8, 9]], [[10, 11, 12], [13, 14, 15], [16, 17, 18]], [[19, 20, 21], [22, 23, 24], [25, 26, 27]]]) print(arr2) # 获取三维数组的第一个二维数组 print(arr2[0])
In summary, NumPy provides rich functions and tools to process arrays, and provides many convenient mathematical functions and operations. By mastering the usage of these functions, the efficiency and convenience of array processing can be greatly improved. The above is only a small part of the function functions and uses in NumPy. I hope it will be helpful to readers' learning and practice.
The above is the detailed content of In-depth analysis of the functions and applications of numpy functions. For more information, please follow other related articles on the PHP Chinese website!
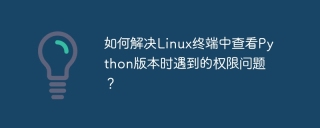
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
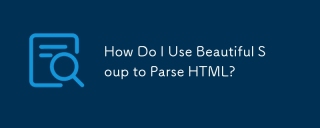
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
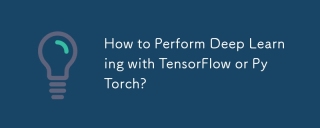
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
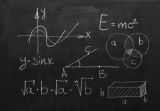
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
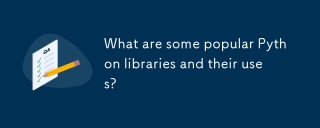
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
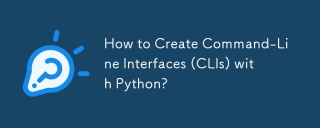
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.

When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
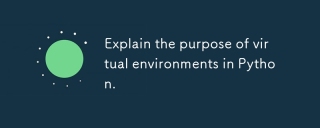
The article discusses the role of virtual environments in Python, focusing on managing project dependencies and avoiding conflicts. It details their creation, activation, and benefits in improving project management and reducing dependency issues.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Zend Studio 13.0.1
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool