Use numpy to achieve efficient random number generation
Random numbers have important applications in many fields, such as simulation experiments, initialization of machine learning algorithms, cryptography, etc. . Numpy is an efficient scientific computing library that also provides rich functions and tools for generating random numbers. This article will introduce how to use numpy to efficiently generate random numbers and give specific code examples.
The random number generation functions in the numpy library are mainly concentrated in the random module. The following are some commonly used random number generation functions and examples of their use:
- Generate random numbers that obey uniform distribution
Uniformly distributed random numbers within a given interval equally likely to be generated. Numpy provides the rand function to generate uniformly distributed random numbers. The code example is as follows:
import numpy as np # 生成一个服从[0, 1)区间均匀分布的随机数 random_num = np.random.rand() print(random_num) # 生成一个服从[10, 20)区间均匀分布的随机数 random_num = np.random.uniform(10, 20) print(random_num) # 生成一个3x3的数组,其中的元素服从[0, 1)区间均匀分布 random_array = np.random.rand(3, 3) print(random_array)
- Generate random numbers that obey normal distribution
Normally distributed Random numbers have a bell-shaped distribution centered on the mean. The randn function is provided in numpy to generate random numbers that obey the standard normal distribution. You can also use the normal function to generate normal distributed random numbers with arbitrary mean and variance. The following is a code example:
import numpy as np # 生成一个服从标准正态分布的随机数 random_num = np.random.randn() print(random_num) # 生成一个服从均值为5,方差为2的正态分布随机数 random_num = np.random.normal(5, 2) print(random_num) # 生成一个4x4的数组,其中的元素服从标准正态分布 random_array = np.random.randn(4, 4) print(random_array)
- Random arrangement and selection
Sometimes you need to randomly arrange an array, or randomly select a part of elements from an array. Numpy provides shuffle and choice functions to complete these operations. The following is a code example:
import numpy as np # 随机排列一个数组 array = np.array([1, 2, 3, 4, 5]) np.random.shuffle(array) print(array) # 从一个数组中随机选择3个元素 array = np.array([1, 2, 3, 4, 5]) random_choice = np.random.choice(array, size=3, replace=False) print(random_choice)
- Generate random integers
In addition to generating random floating point numbers, numpy also provides functions for generating random integers. The randint function can generate random integers within a specified range, and the choice function can also be used to generate random integers within a specified range. The following is a code example:
import numpy as np # 生成一个[1, 10]范围内的随机整数 random_int = np.random.randint(1, 11) print(random_int) # 从一个数组中随机选择一个整数 array = np.array([1, 2, 3, 4, 5]) random_choice = np.random.choice(array) print(random_choice)
Through the above examples, we can see that numpy provides a wealth of random number generation functions to meet the needs of various application scenarios. When generating a large number of random numbers, numpy's efficiency advantage is particularly obvious, which can greatly improve the running speed of the program.
To sum up, it is very convenient to use numpy to achieve efficient random number generation. I hope the introduction in this article can help readers better understand and use the random number generation function in the numpy library.
The above is the detailed content of Efficient random number generation using numpy. For more information, please follow other related articles on the PHP Chinese website!
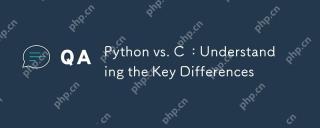
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
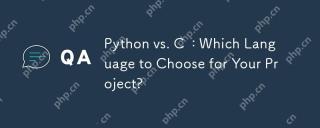
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
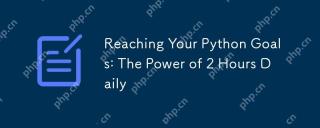
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
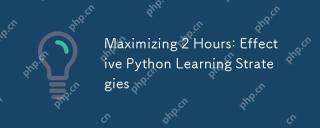
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
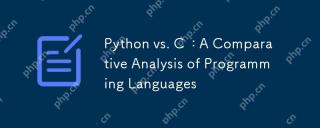
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
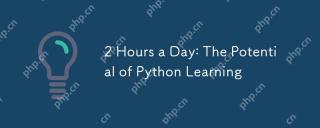
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
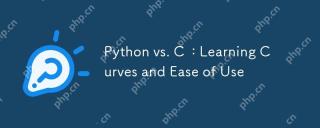
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
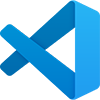
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 English version
Recommended: Win version, supports code prompts!

Atom editor mac version download
The most popular open source editor