


In-depth discussion of Golang variable escape principle: performance impact and optimization methods
Exploration on the principle of variable escape in Golang: impact on program performance and optimization solutions
Introduction:
Golang is a programming language known for its efficient performance. It provides an automatic garbage collection mechanism so that developers do not need to worry about memory allocation and release issues. However, in Golang's concurrent programming, the escape of variables will have a certain impact on program performance. This article will explore the principles of Golang variable escape, propose corresponding performance optimization solutions, and give specific code examples.
1. Golang variable escape principle
In Golang, the compiler allocates memory for variables created inside the function. Generally speaking, local variables are allocated on the stack, while global variables are allocated on the heap. When the compiler cannot determine when a variable will be freed, it will allocate it on the heap. This situation is called the escape of the variable.
Variable escape will cause the memory allocation and release of variables to be done at runtime rather than at compile time. This increases runtime overhead and reduces program performance.
2. The impact of variable escape on program performance
- The cost of heap memory allocation is high
Compared with allocation on the stack, memory allocation on the heap requires more calls System functions, and the way of heap memory allocation is more complicated than allocation on the stack. Therefore, the cost of heap memory allocation will be higher than the cost of allocation on the stack. - Increased overhead of garbage collection
Escape variables require garbage collection to release memory, and garbage collection is a relatively time-consuming operation. The increase in escape variables will lead to an increase in the frequency of garbage collection, thus increasing the overhead of the program. - Reduction in concurrency security
Escape variables will cause dynamic allocation of memory. Multiple concurrent goroutines may access and modify the same memory at the same time, resulting in data inconsistency and reducing the security of concurrent programs. .
3. Optimization plan
- Allocation on stack
With the "stack escape" feature of Go language, we can allocate escape variables on the stack through some optimization methods superior. This can reduce the cost of heap memory allocation and garbage collection overhead. - Reduce escape situations
Through reasonable program design and code optimization, the situations of escaped variables can be reduced, thereby improving program performance. Specifically: - Avoid creating a large number of temporary variables in loops and try to reuse existing variables.
- Use pointers or reference types to reduce copy creation.
- Avoid passing escape variables to external functions.
- Optimize garbage collection
For codes with many escape variables, you can reduce program overhead by optimizing garbage collection. Specifically include: - Select the appropriate garbage collection algorithm and parameter configuration.
- Limit the duration of garbage collection to avoid long pauses.
4. Code Example
The following is a simple example code to demonstrate the situation of variable escape and the corresponding optimization plan:
func foo() *int { x := 10 // 局部变量,分配在栈上 return &x // 返回局部变量的指针,产生逃逸 } func main() { a := foo() fmt.Println(*a) }
In the above code, the variables x
is allocated on the stack. But since the pointer of x
is returned outside the main
function, x
escapes.
For this example, we can use the following optimization solution:
- Allocate
x
on the heap to reduce escape situations.
func foo() *int { x := new(int) // 将x分配在堆上 *x = 10 return x } func main() { a := foo() fmt.Println(*a) }
In the optimized code, we allocate the variable x
directly on the heap by using the new
keyword to avoid variable escape.
Conclusion:
Variable escape in Golang has a certain impact on the performance of the program. Reasonable optimization solutions can reduce the generation of escape variables, thereby improving program performance. By allocating on the stack, reducing escape situations, and optimizing garbage collection, we can optimize the execution efficiency of Golang programs. In actual development, developers should select and use appropriate optimization solutions based on specific circumstances to improve the overall performance of the program.
The above is the detailed content of In-depth discussion of Golang variable escape principle: performance impact and optimization methods. For more information, please follow other related articles on the PHP Chinese website!
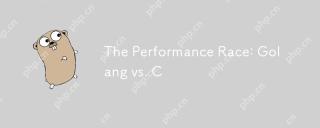
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
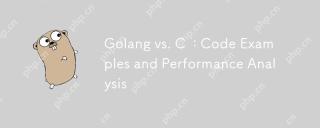
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
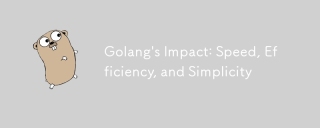
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
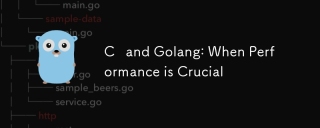
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
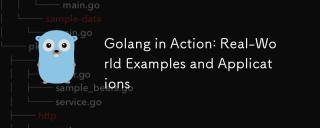
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
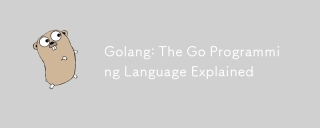
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
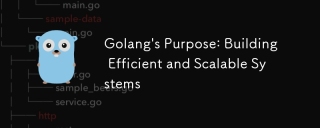
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
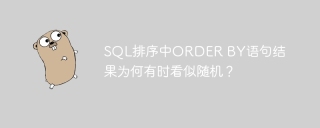
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
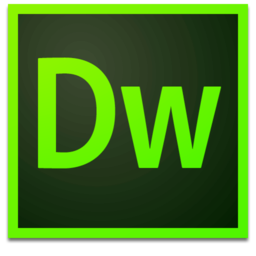
Dreamweaver Mac version
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
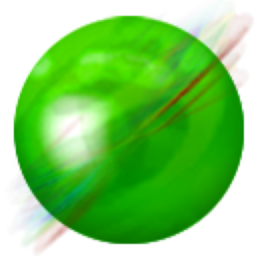
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!