


Implementation and application analysis of Python multiple inheritance
Analysis of the principles and practices of multiple inheritance in Python
In Python's object-oriented programming, multiple inheritance is a very powerful and flexible feature. Through multiple inheritance, a class can inherit properties and methods from multiple parent classes, thereby better realizing code reuse and function combination. This article will analyze the principles of multiple inheritance in Python and demonstrate the practice of multiple inheritance through specific code examples.
First of all, we need to understand the principle of multiple inheritance in Python. In Python, a class can inherit properties and methods from multiple parent classes at the same time. This inheritance method is called multiple inheritance. The feature of multiple inheritance allows a subclass to have the characteristics of multiple parent classes, thereby achieving a higher level of code reuse.
In Python, multiple inheritance is achieved by specifying multiple parent classes when creating a subclass. The following is a simple example:
class Parent1: def method1(self): print("This is Parent1's method1") class Parent2: def method2(self): print("This is Parent2's method2") class Child(Parent1, Parent2): pass # 创建子类实例 child = Child() # 调用父类的方法 child.method1() # 输出:This is Parent1's method1 child.method2() # 输出:This is Parent2's method2
In the above example, Parent1 and Parent2 are two parent classes, and Child is a subclass. By specifying multiple parent classes in the definition of the Child class, it is achieved Multiple inheritance. The subclass Child can call the methods method1 and method2 defined in the parent class Parent1 and Parent2.
It should be noted that the method search order in multiple inheritance is based on the order of subclass inheritance. In the above example, the Child class first inherits the Parent1 class and then the Parent2 class. Therefore, when calling a method, it first checks whether there is a corresponding method in the Parent1 class. If so, it calls it. If not, it then searches for the Parent2 class. If there are methods with the same name in multiple parent classes, Python will give priority to calling the method of the parent class that is first inherited in order of inheritance.
The following is a more complex example that implements a child class Child through multiple inheritance. This class inherits the properties and methods of both the father class Father and the mother class Mother:
class Father: def __init__(self, name): self.name = name def info(self): print("My name is", self.name) print("I'm your father") class Mother: def __init__(self, age): self.age = age def info(self): print("I'm", self.age, "years old") print("I'm your mother") class Child(Father, Mother): def __init__(self, name, age): Father.__init__(self, name) Mother.__init__(self, age) child = Child("Tom", 10) child.info() # 输出:My name is Tom I'm 10 years old
In the above In the example, the Father class and the Mother class represent father and mother respectively, and the Child class inherits the properties and methods of both through multiple inheritance. By calling the constructor of the parent class sequentially in the constructor of the Child class, the properties of the parent class can be initialized. When calling the info method of the Child class, the info method of the Father class will be called first according to the inheritance order, and then the info method of the Mother class will be called, thus realizing the calling order of methods in multiple inheritance.
Multiple inheritance is a very powerful feature in Python's object-oriented programming. Through reasonable use of multiple inheritance, a higher level of code reuse and function combination can be achieved. However, multiple inheritance also has some problems, such as method name conflicts and confusing inheritance relationships. When using multiple inheritance, you need to pay attention to conflicts in inheritance order and method names to avoid unexpected results and errors.
To sum up, multiple inheritance is a very useful feature in Python object-oriented programming, which can realize the inheritance of attributes and methods of multiple parent classes, thereby achieving a higher level of code reuse and function combination. By using multiple inheritance appropriately, you can improve the readability and maintainability of your code, and achieve more elegant and efficient code.
The above is the detailed content of Implementation and application analysis of Python multiple inheritance. For more information, please follow other related articles on the PHP Chinese website!
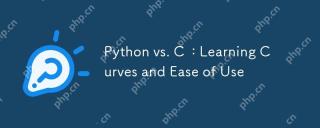
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.

Python and C have significant differences in memory management and control. 1. Python uses automatic memory management, based on reference counting and garbage collection, simplifying the work of programmers. 2.C requires manual management of memory, providing more control but increasing complexity and error risk. Which language to choose should be based on project requirements and team technology stack.
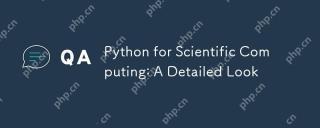
Python's applications in scientific computing include data analysis, machine learning, numerical simulation and visualization. 1.Numpy provides efficient multi-dimensional arrays and mathematical functions. 2. SciPy extends Numpy functionality and provides optimization and linear algebra tools. 3. Pandas is used for data processing and analysis. 4.Matplotlib is used to generate various graphs and visual results.
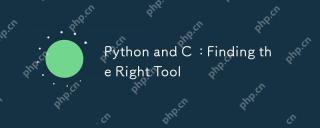
Whether to choose Python or C depends on project requirements: 1) Python is suitable for rapid development, data science, and scripting because of its concise syntax and rich libraries; 2) C is suitable for scenarios that require high performance and underlying control, such as system programming and game development, because of its compilation and manual memory management.
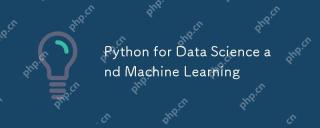
Python is widely used in data science and machine learning, mainly relying on its simplicity and a powerful library ecosystem. 1) Pandas is used for data processing and analysis, 2) Numpy provides efficient numerical calculations, and 3) Scikit-learn is used for machine learning model construction and optimization, these libraries make Python an ideal tool for data science and machine learning.
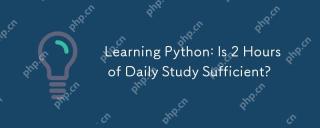
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
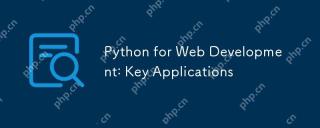
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
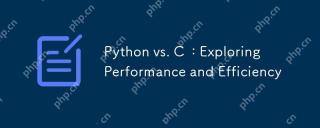
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
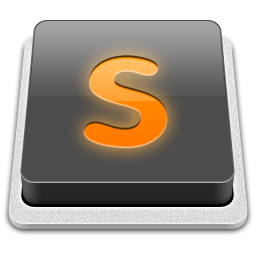
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.