


An in-depth analysis of the Java factory pattern: distinguishing and applying the differences between simple factories, factory methods and abstract factories
Detailed explanation of Java factory pattern: Understand the differences and application scenarios of simple factories, factory methods and abstract factories
Introduction
In the process of software development, faced with complex In the object creation and initialization process, we often need to use the factory pattern to solve this problem. As a commonly used object-oriented programming language, Java provides a variety of factory pattern implementations. This article will introduce in detail the three common implementation methods of the Java factory pattern: simple factory, factory method and abstract factory, and conduct an in-depth analysis of their differences and application scenarios.
1. Simple Factory Pattern
Simple factory pattern is also called static factory pattern. It is a creational design pattern. In the simple factory pattern, a factory class is responsible for creating instances of multiple product classes. According to the client's request, the factory class decides which instance to create through simple logical judgment.
- Structure
The structure of the simple factory pattern includes three main roles: - Factory class (Factory): responsible for creating instances of various products.
- Abstract product class (Product): defines the public interface of the product and abstracts the common characteristics of specific product classes.
- Concrete Product Class (ConcreteProduct): Implements the interface defined in the abstract product class. The concrete product class is an object created by the factory class.
- Implementation steps
The steps to implement the simple factory pattern are as follows: - Define the abstract product class, including the public interface of the product.
- Create a specific product class and implement the interface in the abstract product class.
- Create a factory class and return corresponding specific product class objects according to different client requests.
- Advantages and Disadvantages
The advantage of the simple factory model is that it is simple to implement. The client does not need to pay attention to the creation process of specific products, and only needs to create products through the factory class. The disadvantage is that it violates the opening and closing principle. If you need to add a new product, you need to modify the logic code of the factory class. - Application scenarios
The simple factory pattern is suitable for the following situations: - Need to create different types of objects according to client requests.
- The client only needs to care about the interface of the product class and does not care about the specific implementation class.
2. Factory method pattern
The factory method pattern is also called the polymorphic factory pattern, which is a creational design pattern. In the factory method pattern, an interface is defined for creating objects, and the subclass determines the specific class to be instantiated.
- Structure
The structure of the factory method pattern includes four main roles: - Abstract factory class (Factory): defines the interface for creating objects, which can be an interface or an abstract class .
- Concrete Factory Class (ConcreteFactory): Implements the interface defined in the abstract factory class and is responsible for the creation of specific objects.
- Abstract product class (Product): defines the public interface of the product and abstracts the common characteristics of specific product classes.
- Concrete Product Class (ConcreteProduct): Implements the interface defined in the abstract product class. The concrete product class is an object created by the concrete factory class.
- Implementation steps
The steps to implement the factory method pattern are as follows: - Define the abstract product class, including the public interface of the product.
- Define an abstract factory class and declare the methods used to create products.
- Create a specific product class and implement the interface in the abstract product class.
- Create a specific factory class, implement the methods in the abstract factory class, and return the corresponding specific product class objects according to requirements.
- Advantages and Disadvantages
The advantage of the factory method model is that it overcomes the shortcomings of the simple factory model and complies with the opening and closing principle. When adding new products, you only need to add specific factory classes. But the disadvantage is that it is cumbersome. Every time you add a product, you need to add a specific factory class. - Application scenarios
The factory method pattern is suitable for the following situations: - The object that the client needs to create is determined by the subclass.
- The client needs to handle the details of the specific product.
3. Abstract Factory Pattern
The abstract factory pattern is the most abstract and complex form of the factory pattern. It is a creational design pattern. In the abstract factory pattern, multiple factory methods are organized together to form a collection of factories.
- Structure
The structure of the abstract factory pattern includes four main roles: - Abstract Factory Class (AbstractFactory): defines a set of interfaces for creating objects.
- Concrete Factory Class (ConcreteFactory): Implements the interface defined in the abstract factory class and is responsible for the creation of specific objects.
- Abstract Product Class (AbstractProduct): defines the public interface of the product and abstracts the common characteristics of specific product classes.
- Concrete Product Class (ConcreteProduct): Implements the interface defined in the abstract product class. The concrete product class is an object created by the concrete factory class.
- Implementation steps
The implementation steps of the abstract factory pattern are as follows: - Define the abstract product class, including the public interface of the product.
- Define an abstract factory class and declare the methods used to create products.
- Create a specific product class and implement the interface in the abstract product class.
- Create a specific factory class, implement the methods in the abstract factory class, and return the corresponding specific product class objects according to requirements.
- Advantages and Disadvantages
The advantage of the abstract factory pattern is that it overcomes the shortcomings of the factory method pattern and can create multiple product hierarchical structures. The disadvantage is that it is difficult to add a new product level structure, and the abstract factory class needs to be modified. - Application scenarios
The abstract factory pattern is suitable for the following situations: - It is necessary to create product families with multiple product hierarchical structures.
- The client needs to handle products with multiple product hierarchy structures.
Conclusion
The Java factory pattern is a commonly used design pattern. Proper use of the factory pattern can help us solve the creation and initialization process of complex objects. When choosing a specific factory pattern implementation method, make the choice based on actual needs. Reasonable and flexible use of different factory patterns can improve the maintainability and encapsulation of the code, reduce the coupling of the code, and make the software system more flexible and reliable. Extension. By deeply understanding the differences and application scenarios of simple factories, factory methods, and abstract factories, you can better apply the factory pattern in actual development.
The above is the detailed content of An in-depth analysis of the Java factory pattern: distinguishing and applying the differences between simple factories, factory methods and abstract factories. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
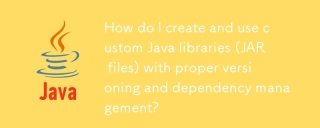
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
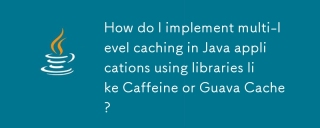
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
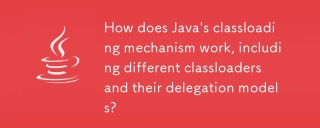
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
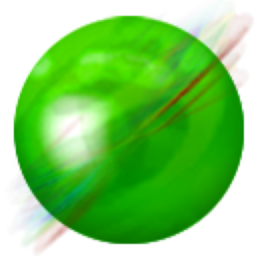
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
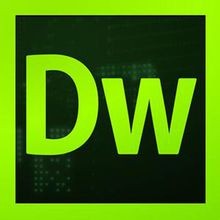
Dreamweaver CS6
Visual web development tools
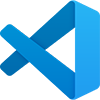
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
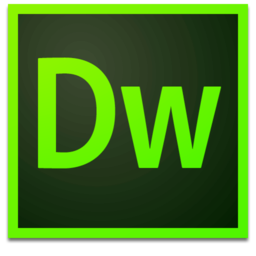
Dreamweaver Mac version
Visual web development tools