How to choose a suitable fast fixed positioning structure requires specific code examples
In modern software development, fast fixed positioning is a very important function. Whether it's web design, mobile app development, or embedded systems, we all need to be able to pinpoint the element or object that needs to be manipulated. A good fixed positioning structure can not only improve development efficiency, but also improve user experience. This article explains how to choose a suitable fast fixed positioning structure and provides specific code examples.
First of all, we need to clarify the definition of rapid fixed positioning. Fast fixed positioning refers to quickly finding elements that meet specific conditions in large-scale data through certain algorithms and data structures. Choosing an appropriate fixed positioning structure can greatly improve query efficiency and reduce resource consumption.
When choosing a fixed positioning structure, you need to consider the following factors:
- Data scale: Different data scales require different data structures. For small-scale data, you can choose simple data structures (such as arrays, linked lists). For large-scale data, more efficient data structures (such as hash tables, trees, graphs) should be selected.
- Query requirements: Choose the appropriate data structure according to the specific query requirements. For example, if you need to find an element quickly, you can use a hash table or a binary search tree. If you need to find a set of elements that meet specific conditions, you can use a hash table, red-black tree, or B-tree.
- Memory occupation: Different data structures occupy different memory spaces. When choosing a fixed positioning structure, consider the memory limitations of your system. If memory resources are limited, you can choose to compress the data structure or use external storage.
- Platform adaptability: Fast fixed positioning usually needs to run on different platforms, so it is necessary to choose a data structure with good platform adaptability. For example, you can choose a cross-platform data structure library or use language-specific data structures.
Next, we will use several sample codes to demonstrate how to choose a suitable fast fixed positioning structure.
Example 1: Quickly find specified elements
Suppose we have a student information database that contains students' names, student numbers, and ages. We need to quickly find information about a student. In this case, a hash table can be used to store student information.
// 学生信息数据库 std::unordered_map<std::string, StudentInfo> studentDatabase; // 添加学生信息 StudentInfo student; student.name = "张三"; student.number = "2001001"; student.age = 20; studentDatabase.insert(std::make_pair(student.number, student)); // 查找学生信息 std::string number = "2001001"; auto iter = studentDatabase.find(number); if (iter != studentDatabase.end()) { StudentInfo student = iter->second; std::cout << "姓名:" << student.name << std::endl; std::cout << "学号:" << student.number << std::endl; std::cout << "年龄:" << student.age << std::endl; }
Example 2: Quickly find a set of elements that meet the conditions
Suppose we have a personnel management system that contains employees' names, departments, and salary information. We need to find all employees whose salary is within a certain range. In this case, a binary search tree or a red-black tree can be used to store employee information.
// 员工信息结构体 struct EmployeeInfo { std::string name; std::string department; int salary; }; // 员工信息比较函数 bool compareBySalary(const EmployeeInfo& employee1, const EmployeeInfo& employee2) { return employee1.salary < employee2.salary; } // 员工信息数据库 std::set<EmployeeInfo, decltype(compareBySalary)*> employeeDatabase(compareBySalary); // 添加员工信息 EmployeeInfo employee1; employee1.name = "张三"; employee1.department = "销售部"; employee1.salary = 3000; employeeDatabase.insert(employee1); EmployeeInfo employee2; employee2.name = "李四"; employee2.department = "技术部"; employee2.salary = 5000; employeeDatabase.insert(employee2); // 查找工资在[4000, 6000]范围内的员工信息 EmployeeInfo employee; employee.salary = 4000; auto iter = employeeDatabase.lower_bound(employee); while (iter != employeeDatabase.end() && iter->salary <= 6000) { std::cout << "姓名:" << iter->name << std::endl; std::cout << "部门:" << iter->department << std::endl; std::cout << "工资:" << iter->salary << std::endl; ++iter; }
The above example codes demonstrate the scenarios of quickly finding specified elements and finding a set of elements that meet the conditions respectively. By choosing a suitable fixed positioning structure, we can complete these operations efficiently and improve development efficiency.
In summary, choosing a suitable fast fixed positioning structure requires considering factors such as data size, query requirements, memory usage, and platform adaptability. According to specific needs, choosing the appropriate data structure can improve query efficiency and improve user experience. In actual development, we can comprehensively evaluate these factors and select the most appropriate fixed positioning structure.
The above is the detailed content of How to choose a suitable and efficient fixed positioning architecture. For more information, please follow other related articles on the PHP Chinese website!
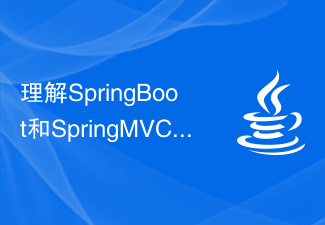
对比SpringBoot与SpringMVC,了解它们的差异随着Java开发的不断发展,Spring框架已经成为了许多开发人员和企业的首选。在Spring的生态系统中,SpringBoot和SpringMVC是两个非常重要的组件。虽然它们都是基于Spring框架的,但在功能和使用方式上却有一些区别。本文将重点对比一下SpringBoot与Sprin
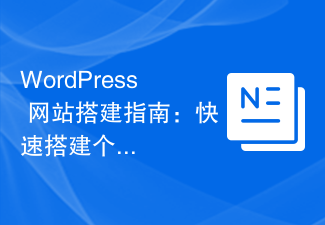
WordPress网站搭建指南:快速搭建个人网站随着数字化时代的到来,拥有一个个人网站已经成为了一种时尚和必要。而WordPress作为最受欢迎的网站搭建工具,让搭建个人网站变得更加容易和便捷。本文将为大家提供一个快速搭建个人网站的指南,包含具体的代码示例,希望可以帮助到想要拥有自己网站的朋友们。第一步:购买域名和主机在开始搭建个人网站之前,首先要购买自己
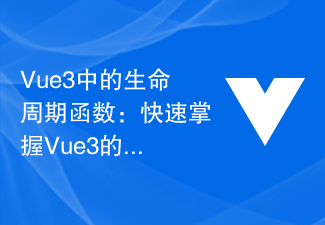
Vue3是目前前端界最热门的框架之一,而Vue3的生命周期函数是Vue3中非常重要的一部分。Vue3的生命周期函数可以让我们实现在特定的时机触发特定的事件,增强了组件的高度可控性。本文将从Vue3的生命周期函数的基本概念、各个生命周期函数的作用和使用方法以及实现案例等方面进行详细探究和讲解,帮助读者快速掌握Vue3的生命周期函数。一、Vue3的生命周期函数的
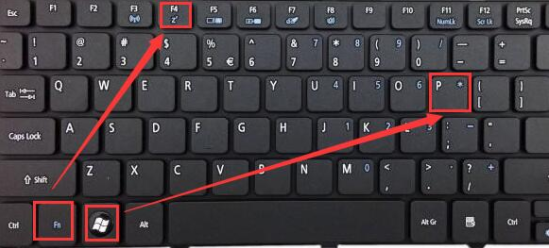
电脑怎么切屏?在使用电脑的时候,有的朋友会使用两个甚至三个显示屏,但是在使用的时候,就会遇到需要切换屏幕的问题,那么电脑怎么切屏呢?一些朋友不知道电脑快速切屏方法,所以本期将教大家win10电脑怎么快速切屏。win10电脑怎么快速切屏?具体的方法如下:1、外接显示屏以后,同时按下【Fn】+【F4】或者【win】+【P】即可选择外接显示器。2、第二种方法是,在桌面空白处鼠标右键,然后选择【屏幕分辨率】。3、然后在【多显示器】中,就能够切换屏幕了。以上就是小编带来的win10电脑怎么快速切屏的全部内
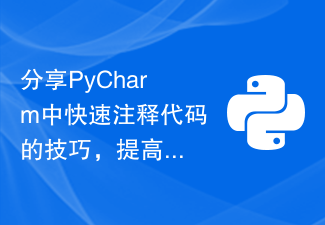
效率提升!PyCharm中快速注释代码的方法分享在日常的软件开发工作中,我们经常需要注释掉一部分代码进行调试或者调整。如果手动逐行添加注释,这无疑会增加我们的工作量和耗费时间。而PyCharm作为一款强大的Python集成开发环境,提供了快速注释代码的功能,大大提升了我们的开发效率。本文将分享一些在PyCharm中快速注释代码的方法,并提供具体的代码示例。单
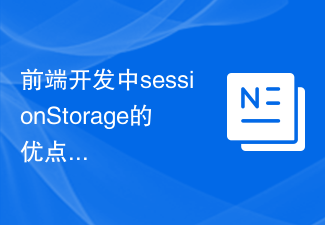
sessionStorage在前端开发中的优势与应用案例分析随着Web应用的发展,前端开发的需求也越来越多样化。前端开发人员需要使用各种工具和技术来提高用户体验,其中,sessionStorage是一个非常有用的工具。本文将介绍sessionStorage在前端开发中的优势,以及几个具体的应用案例。sessionStorage是HTML5提供的一种本地存储方
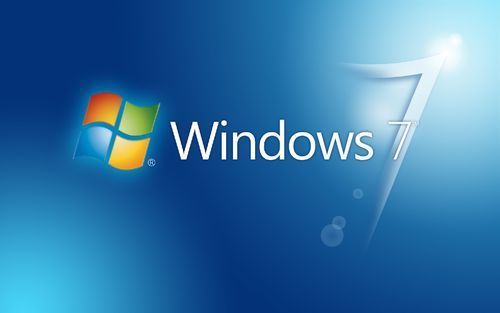
win7如何快速截屏?win7系统之中有着很多便捷操作功能,可以为各位提供非常多样化的便捷服务。很多win7系统的用户在使用电脑的过程中,想要通过win7系统之中的快捷键进行截屏,但是却不清楚具体的快捷键是哪些,因此无法正常使用,那么,这些快捷截屏键究竟是哪些呢?下面小编就为各位带来win7快速截屏键介绍。win7快速截屏键介绍1、按Prtsc键截图这样获取的是整个电脑屏幕的内容,按Prtsc键后,可以直接打开画图工具,接粘贴使用。也可以粘贴在QQ聊天框或者Word文档中,之后再选择保存即可。2
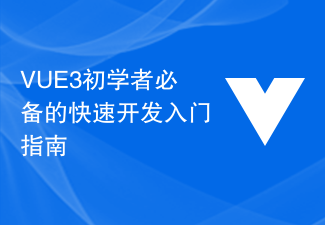
VUE3初学者必备的快速开发入门指南Vue是一款流行的JavaScript框架,它的易用性、高度定制性和快速开发模式使得它在前端开发中广受欢迎。而最新的Vue3则推出了更多强大的特性,包括性能优化、TypeScript支持、CompositionAPI以及更好的自定义渲染器等等。本篇文章将为Vue3初学者提供一份快速开发入门指南,帮助你快速上手Vue3开发


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
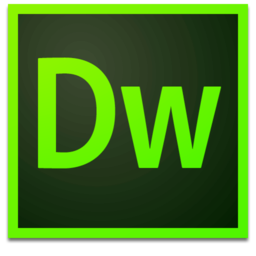
Dreamweaver Mac version
Visual web development tools
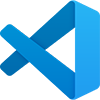
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
