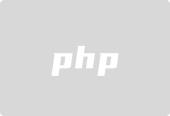
Title: In-depth analysis: The role and importance of the Java virtual machine
Abstract:
Java Virtual Machine (JVM) as the Java language The core component is mainly responsible for interpreting and executing Java bytecode. This article will deeply analyze the role and importance of the Java virtual machine, introduce the working principle, memory management, garbage collection and performance optimization of the JVM, and provide specific code examples.
- Introduction
The Java virtual machine is the core of running Java programs. It is responsible for interpreting and executing the bytecode generated by the Java compiler, so that Java programs can run on different operating systems and hardware platforms. run on. The virtual machine is an abstraction layer that provides developers with a unified programming model and simplifies cross-platform development.
- The working principle of JVM
The working principle of JVM includes bytecode interpretation, just-in-time compilation and other optimization technologies. First, the Java source code is compiled into a bytecode file by the compiler, and then the bytecode file is loaded by the virtual machine and interpreted and executed. During the running process, the hot code is compiled into local machine code through a just-in-time compiler to improve execution efficiency. In addition, the JVM also uses dynamic compilation technology, escape analysis, method inlining and other optimization strategies to further optimize code execution.
- JVM memory management
JVM manages the memory of Java programs, including heap, stack and method area. Heap space is used to store object instances, and objects that are no longer used are automatically recycled through the garbage collection mechanism. The stack space is used to store local variables and temporary data for method calls. The popping and pushing operations of stack frames are very efficient. The method area is used to store class information, constants, static variables, etc.
- Garbage Collection
Garbage collection is one of the important features of the JVM. It improves program performance and reliability by automatically detecting and recycling memory that is no longer used. The JVM uses a generational collection algorithm to divide the heap space into the new generation and the old generation, and adopts different garbage collection strategies based on the survival time of the object. Common garbage collection algorithms include mark-sweep algorithm, copy algorithm, mark-compact algorithm, etc.
- Performance Optimization
JVM provides a wealth of performance tuning options, and developers can optimize program performance by adjusting parameters and using tools. Commonly used performance optimization techniques include using an appropriate garbage collector, adjusting the heap size and new generation ratio, optimizing code to reduce memory usage, etc. This article will use specific code examples to show how to use JVM parameters and tools for performance optimization.
- Code Example
The following is a simple Java code example that shows how to use JVM parameters to set the heap size and new generation ratio, and how to use JVM tools to analyze the performance of the program.
public class MemoryExample {
public static void main(String[] args) {
// 设置堆大小为256M
//-Xmx256m
// 设置新生代比例为30%
//-XX:NewRatio=3
// 打印总内存和可用内存
long totalMemory = Runtime.getRuntime().totalMemory();
long freeMemory = Runtime.getRuntime().freeMemory();
System.out.println("Total Memory: " + totalMemory + " bytes");
System.out.println("Free Memory: " + freeMemory + " bytes");
// 进行一些耗内存的操作
int[] array = new int[1000000];
for (int i = 0; i < array.length; i++) {
array[i] = i;
}
// 打印操作后的可用内存
freeMemory = Runtime.getRuntime().freeMemory();
System.out.println("Free Memory after allocation: " + freeMemory + " bytes");
}
}
- Conclusion
As the core component of the Java language, the Java virtual machine is responsible for interpreting and executing Java bytecode. It greatly simplifies the difficulty of cross-platform development and provides rich memory management, garbage collection and performance optimization mechanisms. Developers can optimize the performance and reliability of Java programs by having a deep understanding of how the JVM works and using the appropriate parameters and tools.
Reference:
- "Understanding JVM Internals" by Singh Gaurav
- "Java Performance: The Definitive Guide" by Scott Oaks
The above is the detailed content of Explore the Deep: Understand the capabilities of the Java Virtual Machine and its importance. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn