


Exploring the world of Java software: To understand common Java software applications, specific code examples are required
Introduction:
Java is widely used as a software Developed programming language with rich functionality and strong cross-platform features. In the world of Java software, there are many common applications that we can utilize and learn from. In this article, we will explore some common Java software applications and provide specific code examples to help readers better understand and master these applications.
1. Graphical User Interface (GUI) Application
GUI application is one of the most common applications in Java software development. Java provides powerful graphics libraries that developers can use to create a variety of user interfaces. The following is a sample code for a simple Java GUI application:
import javax.swing.*; public class SimpleGUI extends JFrame { public SimpleGUI() { super("Simple GUI"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setSize(320, 240); setLocationRelativeTo(null); setVisible(true); } public static void main(String[] args) { SwingUtilities.invokeLater(() -> new SimpleGUI()); } }
In this example, we create a window named "Simple GUI" and set the size and position of the window. By calling the setVisible(true)
method, we display the window on the screen. In the main
method, we use the SwingUtilities.invokeLater
method to start the window creation and display process.
2. Network Applications
Java is also a programming language that is very suitable for developing network applications. Using Java's network library, developers can easily create various network applications, such as web servers, clients, and chat applications. The following is a sample code for a simple Java network application:
import java.io.*; import java.net.*; public class SimpleServer { public static void main(String[] args) { try (ServerSocket serverSocket = new ServerSocket(8080)) { System.out.println("Server started on port 8080"); while (true) { Socket socket = serverSocket.accept(); System.out.println("Accepted connection from " + socket.getInetAddress()); new Thread(() -> handleRequest(socket)).start(); } } catch (IOException e) { e.printStackTrace(); } } private static void handleRequest(Socket socket) { try (BufferedReader reader = new BufferedReader(new InputStreamReader(socket.getInputStream())); BufferedWriter writer = new BufferedWriter(new OutputStreamWriter(socket.getOutputStream()))) { String request = reader.readLine(); System.out.println("Received request: " + request); String response = "Hello, client!"; writer.write(response); writer.newLine(); writer.flush(); } catch (IOException e) { e.printStackTrace(); } } }
In this example, we create a simple server application that listens to port 8080 and returns a simple response when receiving a client request. When a new connection comes in, we create a new thread to handle the request in order to handle multiple client requests at the same time. Through the IO stream, we can read the request sent by the client and send the response to the client.
3. Database Application
Java also has rich support for database applications. Developers can use the JDBC library provided by Java to access various relational databases, such as MySQL, Oracle, and PostgreSQL. The following is a sample code for a simple Java database application:
import java.sql.*; public class SimpleDatabaseApp { public static void main(String[] args) { try (Connection conn = DriverManager.getConnection("jdbc:mysql://localhost/mydatabase", "username", "password"); Statement stmt = conn.createStatement()) { System.out.println("Connected to database"); String sql = "SELECT * FROM users"; ResultSet rs = stmt.executeQuery(sql); while (rs.next()) { String name = rs.getString("name"); int age = rs.getInt("age"); System.out.println("Name: " + name + ", Age: " + age); } } catch (SQLException e) { e.printStackTrace(); } } }
In this example, we connect to a MySQL database named "mydatabase" and query a table named "users". By executing the SQL statement and iterating through the result set, we print out each row of data in the table.
Conclusion:
Java is a very powerful and versatile programming language that is widely used in software development. This article introduces common application types in Java software development, including graphical user interfaces, network and database applications, and gives corresponding code examples. By understanding and studying these examples, readers can better master the basic knowledge and skills of Java software development and further expand their application capabilities in the Java software world. I hope readers can continue to improve their Java programming abilities through continued learning and practice, and make more contributions to the world of software development.
The above is the detailed content of Perspective on the Java Software Land: Get Familiar with Common Java Applications. For more information, please follow other related articles on the PHP Chinese website!
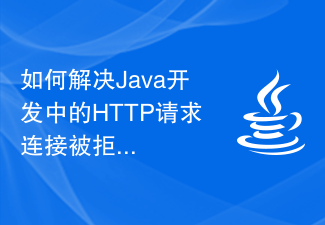
如何解决Java开发中的HTTP请求连接被拒绝问题在进行Java开发中,经常会遇到HTTP请求连接被拒绝的问题。这种问题的出现可能是由于服务器端限制了访问权限,或是网络防火墙阻止了HTTP请求的访问。解决这个问题需要对代码和环境进行一些调整。本文将介绍几种常见的解决方法。检查网络连接和服务器状态首先,确认你的网络连接是正常的,可以尝试访问其他的网站或服务,看
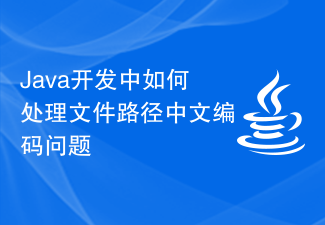
在Java开发中处理文件路径中的中文编码问题是一个常见的挑战,特别是在涉及文件上传、下载和处理等操作时。由于中文字符在不同的编码方式下可能会有不同的表现形式,如果不正确处理,可能会出现乱码或路径无法识别的问题。本文将探讨如何正确处理Java开发中的文件路径中文编码问题。首先,我们需要了解Java中的编码方式。Java内部使用Unicode字符集来表示字符。而
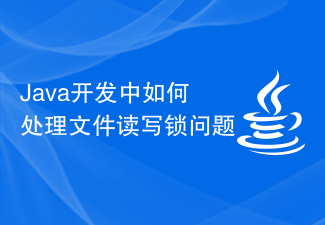
Java是一种功能强大的编程语言,广泛应用于各种领域的开发中,特别是在后端开发中。在Java开发中,处理文件读写锁问题是一个常见的任务。本文将介绍如何在Java开发中处理文件读写锁问题。文件读写锁是为了解决多线程同时读写文件时可能出现的并发冲突问题。当多个线程同时读取一个文件时,不会产生冲突,因为读取是安全的。但是,当一个线程在写入文件时,其他线程可能正在读
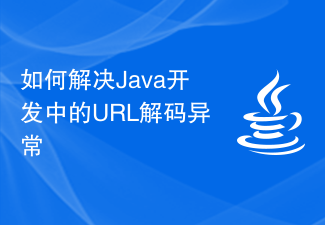
如何解决Java开发中的URL解码异常在Java开发中,我们经常会遇到需要解码URL的情况。然而,由于不同的编码方式或者不规范的URL字符串,有时候会出现URL解码异常的情况。本文将介绍一些常见的URL解码异常以及对应的解决方法。一、URL解码异常的产生原因编码方式不匹配:URL中的特殊字符需要进行URL编码,即将其转换为以%开头的十六进制值。解码时,需要使
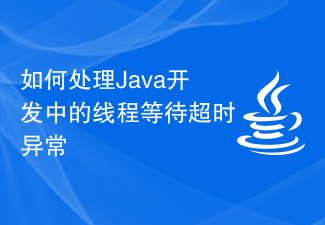
如何处理Java开发中的线程等待超时异常在Java开发中,我们经常会遇到一种情况:当一个线程等待其他线程完成某个任务时,如果等待的时间超过了我们设定的超时时间,我们需要对该异常情况进行处理。这是一个常见的问题,因为在实际应用中,我们无法保证其他线程能在我们设定的超时时间内完成任务。那么,如何处理这种线程等待超时异常呢?下面,我将为你介绍一种常见的处理方法。首
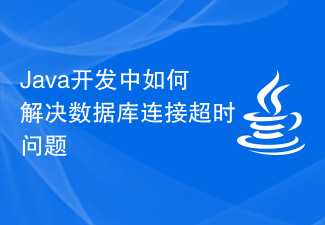
Java开发中如何解决数据库连接超时问题简介:在Java开发中,处理数据库是非常常见的任务之一。尤其是在Web应用程序或后端服务中,与数据库的连接经常需要进行长时间的操作。然而,随着数据库的规模不断增大和访问请求的增加,数据库连接超时问题也开始变得常见。本文将讨论在Java开发中如何解决数据库连接超时问题的方法和技巧。一、理解数据库连接超时问题在开始解决数据
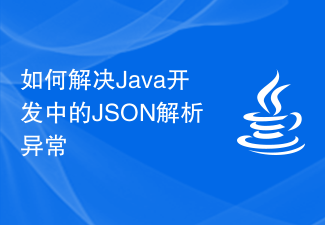
如何解决Java开发中的JSON解析异常JSON(JavaScriptObjectNotation)是一种轻量级的数据交换格式,由于其易读性、易于解析和生成等特点,被广泛应用于网络数据传输、前后端交互等场景。在Java开发中,使用JSON进行数据的序列化和反序列化是非常常见的操作。然而,由于数据的结构和格式多种多样,JSON解析异常在Java开发中时常出
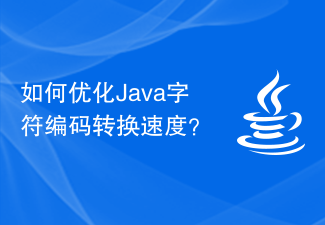
标题:如何处理Java开发中的字符编码转换速度问题导语:随着互联网的发展,字符编码问题在计算机领域变得愈发重要。Java作为一种常用的编程语言,其字符编码转换的速度对于处理大量数据和提供高性能的应用程序至关重要。本文将介绍一些有效的方法和技巧,帮助开发者解决Java开发中的字符编码转换速度问题。一、了解字符编码在解决字符编码转换速度问题之前,我们需要了解一些


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
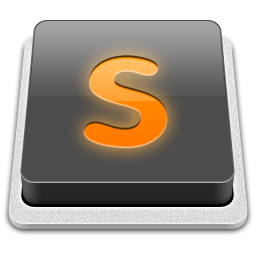
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
