MySQL lock internal implementation analysis and code examples
Introduction:
In a multi-user environment, the data in the database may be read by multiple users at the same time For write operations, the lock mechanism needs to be used to ensure data consistency and concurrency control. MySQL is an open source relational database management system that implements multiple types of locks internally to achieve data concurrency control. This article will analyze the internal implementation of MySQL locks and provide specific code examples.
1. Basic concepts and classification methods of MySQL locks
Locks in MySQL are mainly divided into two categories: Shared Lock and Exclusive Lock.
- Shared lock (read lock): Multiple users can acquire the same shared lock at the same time to protect read operations. Other concurrent users can also obtain the shared lock at the same time, but cannot perform write operations.
- Exclusive lock (write lock): Only one user can obtain an exclusive lock at the same time, which is used to protect write operations. Other concurrent users cannot acquire the exclusive lock at the same time.
2. The internal implementation mechanism of MySQL lock
- Table lock: Table lock is the most basic locking mechanism, with the largest granularity. It's the entire table. After a user acquires a table lock, other users cannot perform read and write operations at the same time.
Code example:
--Request table lock
LOCK TABLES table_name READ/WRITE;
--Release table lock
UNLOCK TABLES; - Row Lock : The row lock has the smallest granularity, and a certain row in the table is locked. After a user acquires a row lock, other users can only operate on other rows and cannot read and write the same row at the same time.
Code example:
--Request row lock
SELECT * FROM table_name WHERE id = 1 FOR UPDATE;
--Release row lock
COMMIT; - Page lock ( Page Lock): Page lock is a compromise between table lock and row lock. It locks in units of data pages. When a user acquires a page lock, other users cannot operate on the rows on the page at the same time.
Code example:
--Request page lock (Innodb engine support)
LOCK TABLES table_name WHERE id = 1;
--Release page lock
UNLOCK TABLES;
3. MySQL lock usage scenarios and precautions
- When designing the database, it is necessary to select an appropriate lock mechanism based on actual needs and concurrent operations. A lighter-weight lock mechanism can improve the performance of concurrent operations, but may cause data consistency problems; a heavier-weight lock mechanism can ensure data consistency, but may reduce concurrency performance.
- When writing SQL statements, you need to make reasonable use of the lock mechanism to control concurrent access. For example, when performing some complex data update operations, transactions and exclusive locks can be used to ensure data integrity and consistency.
Code example:
START TRANSACTION;
LOCK TABLES table_name WRITE;
--Perform update operation
UPDATE table_name SET column = new_value WHERE condition;
--Release lock
UNLOCK TABLES;
COMMIT;
Conclusion:
The internal implementation of MySQL locks is an important tool to ensure database data consistency and concurrency control. Based on actual needs and concurrent operations, choosing an appropriate lock mechanism can effectively improve database performance and data consistency. When writing SQL statements, proper use of locking mechanisms can ensure data integrity and consistency. The article explains the basic concepts and internal implementation mechanisms of MySQL locks through specific code examples, hoping to be helpful to readers.
The above is the detailed content of Analyze the lock mechanism implemented internally in MySQL. For more information, please follow other related articles on the PHP Chinese website!
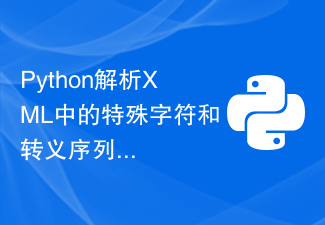
Python解析XML中的特殊字符和转义序列XML(eXtensibleMarkupLanguage)是一种常用的数据交换格式,用于在不同系统之间传输和存储数据。在处理XML文件时,经常会遇到包含特殊字符和转义序列的情况,这可能会导致解析错误或者误解数据。因此,在使用Python解析XML文件时,我们需要了解如何处理这些特殊字符和转义序列。一、特殊字符和
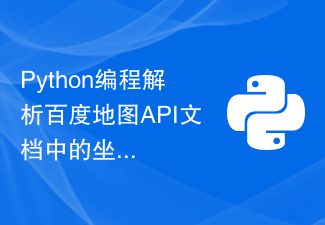
Python编程解析百度地图API文档中的坐标转换功能导读:随着互联网的快速发展,地图定位功能已经成为现代人生活中不可或缺的一部分。而百度地图作为国内最受欢迎的地图服务之一,提供了一系列的API供开发者使用。本文将通过Python编程,解析百度地图API文档中的坐标转换功能,并给出相应的代码示例。一、引言在开发中,我们有时会涉及到坐标的转换问题。百度地图AP
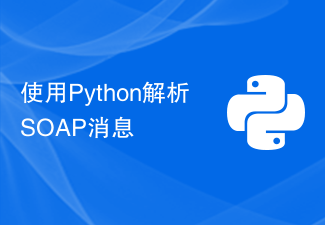
使用Python解析SOAP消息SOAP(SimpleObjectAccessProtocol)是一种基于XML的远程过程调用(RPC)协议,用于在网络上不同的应用程序之间进行通信。Python提供了许多库和工具来处理SOAP消息,其中最常用的是suds库。suds是Python的一个SOAP客户端库,可以用于解析和生成SOAP消息。它提供了一种简单而
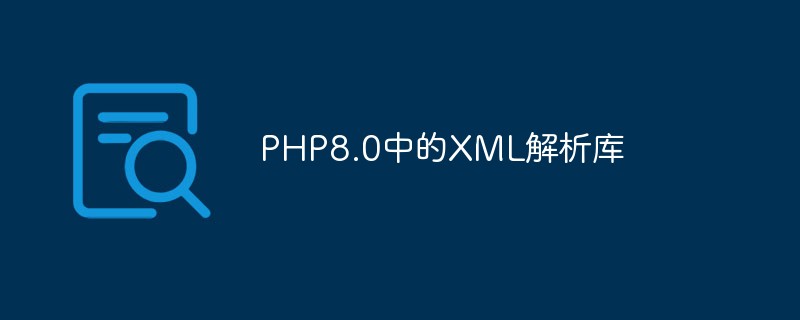
随着PHP8.0的发布,许多新特性都被引入和更新了,其中包括XML解析库。PHP8.0中的XML解析库提供了更快的解析速度和更好的可读性,这对于PHP开发者来说是一个重要的提升。在本文中,我们将探讨PHP8.0中的XML解析库的新特性以及如何使用它。什么是XML解析库?XML解析库是一种软件库,用于解析和处理XML文档。XML是一种用于将数据存储为结构化文档
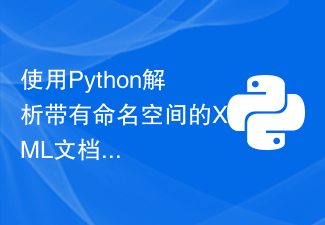
使用Python解析带有命名空间的XML文档XML是一种常用的数据交换格式,能够适应各种应用场景。在处理XML文档时,有时会遇到带有命名空间(namespace)的情况。命名空间可以防止不同XML文档中元素名的冲突,提高了XML的灵活性和可扩展性。本文将介绍如何使用Python解析带有命名空间的XML文档,并给出相应的代码示例。首先,我们需要导入xml.et
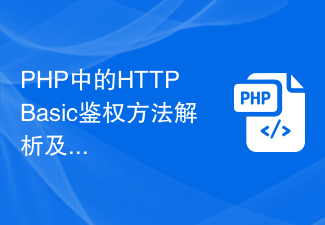
PHP中的HTTPBasic鉴权方法解析及应用HTTPBasic鉴权是一种简单但常用的身份验证方法,它通过在HTTP请求头中添加用户名和密码的Base64编码字符串进行身份验证。本文将介绍HTTPBasic鉴权的原理和使用方法,并提供PHP代码示例供读者参考。一、HTTPBasic鉴权原理HTTPBasic鉴权的原理非常简单,当客户端发送一个请求时
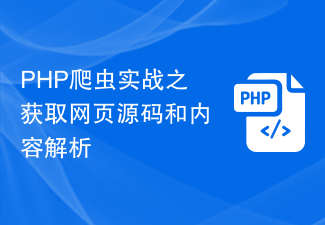
PHP爬虫是一种自动化获取网页信息的程序,它可以获取网页代码、抓取数据并存储到本地或数据库中。使用爬虫可以快速获取大量的数据,为后续的数据分析和处理提供巨大的帮助。本文将介绍如何使用PHP实现一个简单的爬虫,以获取网页源码和内容解析。一、获取网页源码在开始之前,我们应该先了解一下HTTP协议和HTML的基本结构。HTTP是HyperText
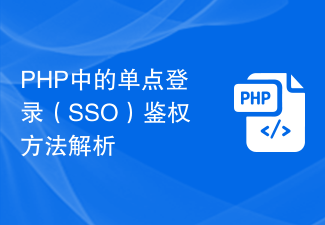
PHP中的单点登录(SSO)鉴权方法解析引言:随着互联网的发展,用户通常要同时访问多个网站进行各种操作。为了提高用户体验,单点登录(SingleSign-On,简称SSO)应运而生。本文将探讨PHP中的SSO鉴权方法,并提供相应的代码示例。一、什么是单点登录(SSO)?单点登录(SSO)是一种集中化认证的方法,在多个应用系统中,用户只需要登录一次,就能访问


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
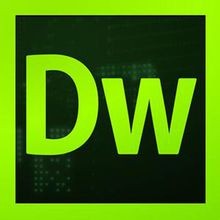
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
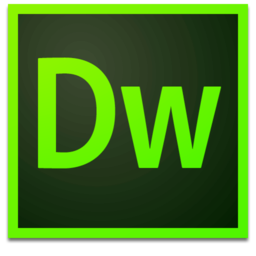
Dreamweaver Mac version
Visual web development tools