


In-depth understanding of wait and notify in Java: Analysis of thread synchronization mechanism
Thread synchronization in Java: Analyzing the working principles of wait and notify methods
In Java multi-threaded programming, synchronization between threads is a very important concept. In actual development, we often need to control the execution sequence and resource access between multiple threads. In order to achieve thread synchronization, Java provides wait and notify methods.
The wait and notify methods are two methods in the Object class. They use the monitor (monitor) mechanism in java to achieve coordination and communication between threads. When a thread is waiting for a certain condition, it can call the wait method of the object, and the thread will enter the waiting state and release the object's lock. When other threads change the state of the object, the object's notify method can be called to notify the waiting thread, allowing it to compete for the lock again and continue execution.
The main working principles of the wait and notify methods are as follows:
- The function of the wait method is to put the current thread into a waiting state until a certain condition is met. Before calling the wait method, the thread must first acquire the object's lock. If it does not acquire it, an IllegalMonitorStateException will be thrown. Once the thread enters the waiting state, it releases the object's lock and enters the waiting queue. The waiting thread can be awakened only when other threads call the notify method or notifyAll method of the corresponding object.
- The notify method is to wake up a thread in the waiting queue so that it can re-compete to acquire the lock and continue execution. If there are multiple threads in the waiting queue, which thread is awakened is undefined and depends on the operating system's scheduling policy. It should be noted that the notify method will only wake up one thread, while the notifyAll method will wake up all waiting threads.
A sample code is given below to demonstrate the use of wait and notify methods:
public class WaitNotifyDemo { private static final Object lock = new Object(); private static boolean flag = false; public static void main(String[] args) { Thread waitThread = new Thread(new WaitTask()); Thread notifyThread = new Thread(new NotifyTask()); waitThread.start(); try { Thread.sleep(2000); } catch (InterruptedException e) { e.printStackTrace(); } notifyThread.start(); } static class WaitTask implements Runnable { @Override public void run() { synchronized (lock) { while (!flag) { try { System.out.println("等待线程进入等待状态"); lock.wait(); } catch (InterruptedException e) { e.printStackTrace(); } } System.out.println("等待线程被唤醒,继续执行"); } } } static class NotifyTask implements Runnable { @Override public void run() { synchronized (lock) { System.out.println("通知线程唤醒等待线程"); lock.notify(); flag = true; } } } }
In the above example, waitThread starts executing first, and when it tries to enter the synchronized block, Since the initial value of flag is false, it will call the wait method to enter the waiting state. Then notifyThread starts and sleeps for 2 seconds. After that, it acquires the lock, sets the value of flag to true, and calls the notify method to wake up the waiting thread. Eventually, waitThread is awakened and execution continues from the place after the wait method.
Through this example, we can better understand how the wait and notify methods work. They are important tools for synchronization and communication between threads, effectively solving competition and resource access problems between threads. In practical applications, reasonable use of wait and notify methods can ensure smooth collaboration between multiple threads.
The above is the detailed content of In-depth understanding of wait and notify in Java: Analysis of thread synchronization mechanism. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
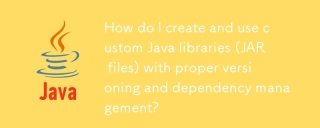
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
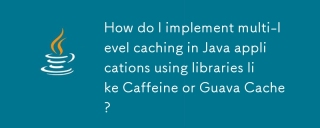
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
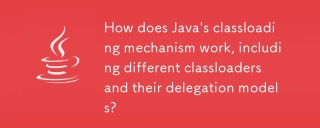
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
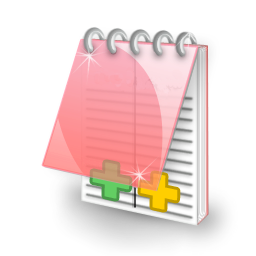
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor