


Realize the design of special statistical charts such as regional heat maps and maps based on ECharts and Java interfaces
With the development of data visualization, various special statistical charts have gradually become popular. More attention and applications. Regional heat maps and maps are two extremely common and useful statistical charts. This article will introduce how to implement the design of regional heat maps and maps based on ECharts and Java interfaces, and provide specific code examples.
1. Introduction to ECharts
ECharts is a flexible and powerful data visualization library open sourced by Baidu. It is based on JavaScript language and can provide beautiful and interactive chart display effects on web pages. The types of charts drawn by ECharts are diverse and can meet different statistical needs.
2. Design and implementation of regional heat map
The regional heat map uses the depth of color to represent the density distribution of regional data. The following is a design example for implementing a regional heat map based on ECharts and Java interfaces.
- Back-end code (Java):
@RestController @RequestMapping("/api") public class HeatMapController { @Autowired private HeatMapService heatMapService; @GetMapping("/heatMapData") public List<HeatMapData> getHeatMapData() { return heatMapService.getHeatMapData(); } } @Service public class HeatMapService { public List<HeatMapData> getHeatMapData() { // 从数据库或其他数据源获取热力图数据 List<HeatMapData> heatMapDataList = new ArrayList<>(); // 假设数据格式为:{x, y, value} heatMapDataList.add(new HeatMapData(10, 20, 100)); heatMapDataList.add(new HeatMapData(20, 30, 150)); heatMapDataList.add(new HeatMapData(30, 40, 200)); return heatMapDataList; } } public class HeatMapData { private int x; private int y; private int value; // getters and setters }
- Front-end code (JavaScript):
$.ajax({ url: '/api/heatMapData', method: 'GET', success: function(data) { var heatData = []; for (var i = 0; i < data.length; i++) { heatData.push([data[i].x, data[i].y, data[i].value]); } // 使用ECharts绘制区域热力图 var myChart = echarts.init(document.getElementById('heatMap')); var option = { tooltip: {}, series: [{ type: 'heatmap', data: heatData }] }; myChart.setOption(option); } });
- HTML page :
<!DOCTYPE html> <html> <head> <title>区域热力图</title> <link rel="stylesheet" href="https://cdn.bootcss.com/echarts/4.3.0/echarts.min.css"> <script src="https://cdn.bootcss.com/jquery/3.4.1/jquery.min.js"></script> <script src="https://cdn.bootcss.com/echarts/4.3.0/echarts.min.js"></script> </head> <body> <div id="heatMap" style="width: 600px; height: 400px;"></div> </body> </html>
Through the above code example, we can implement a regional heat map design based on ECharts and Java interface. First, the back-end Java code provides an interface /api/heatMapData
for obtaining heat map data. Then, the front end requested data through Ajax and used the ECharts library to draw a regional heat map.
3. Map design and implementation
Map is another common statistical chart type and can be implemented through ECharts and Java interfaces. The following is an example of map design based on ECharts and Java interface.
- Back-end code (Java):
@RestController @RequestMapping("/api") public class MapController { @Autowired private MapService mapService; @GetMapping("/mapData") public List<MapData> getMapData() { return mapService.getMapData(); } } @Service public class MapService { public List<MapData> getMapData() { // 从数据库或其他数据源获取地图数据 List<MapData> mapDataList = new ArrayList<>(); // 假设数据格式为:{name, value} mapDataList.add(new MapData("北京", 100)); mapDataList.add(new MapData("上海", 150)); mapDataList.add(new MapData("广州", 200)); return mapDataList; } } public class MapData { private String name; private int value; // getters and setters }
- Front-end code (JavaScript):
$.ajax({ url: '/api/mapData', method: 'GET', success: function(data) { var mapData = []; for (var i = 0; i < data.length; i++) { mapData.push({name: data[i].name, value: data[i].value}); } // 使用ECharts绘制地图 var myChart = echarts.init(document.getElementById('map')); var option = { tooltip: {}, visualMap: { min: 0, max: 500, left: 'left', top: 'bottom', text: ['高', '低'], calculable: true }, series: [{ type: 'map', map: 'china', data: mapData }] }; myChart.setOption(option); } });
- HTML page :
<!DOCTYPE html> <html> <head> <title>地图</title> <link rel="stylesheet" href="https://cdn.bootcss.com/echarts/4.3.0/echarts.min.css"> <script src="https://cdn.bootcss.com/jquery/3.4.1/jquery.min.js"></script> <script src="https://cdn.bootcss.com/echarts/4.3.0/echarts.min.js"></script> </head> <body> <div id="map" style="width: 600px; height: 400px;"></div> </body> </html>
Through the above code example, we can implement a map design based on ECharts and Java interface. The back-end Java code provides an interface /api/mapData
for obtaining map data. The front end requests data through Ajax and uses the ECharts library to draw a map of China.
To sum up, by combining ECharts and Java interfaces, we can easily realize the design of special statistical charts such as regional heat maps and maps. The above code examples are only basic implementations, and specific business logic and data sources need to be expanded and modified according to actual needs.
The above is the detailed content of Realize the design of special statistical charts such as regional heat maps and maps based on ECharts and Java interfaces. For more information, please follow other related articles on the PHP Chinese website!
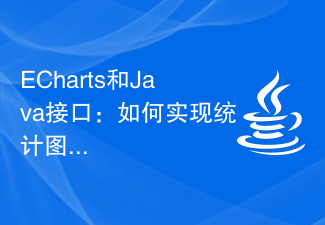
ECharts是一款功能强大、灵活可定制的开源图表库,可用于数据可视化和大屏展示。在大数据时代,统计图表的数据导出和分享功能变得越来越重要。本文将介绍如何通过Java接口实现ECharts的统计图表数据导出和分享功能,并提供具体的代码示例。一、ECharts简介ECharts是百度开源的一款基于JavaScript和Canvas的数据可视化库,具有丰富的图表
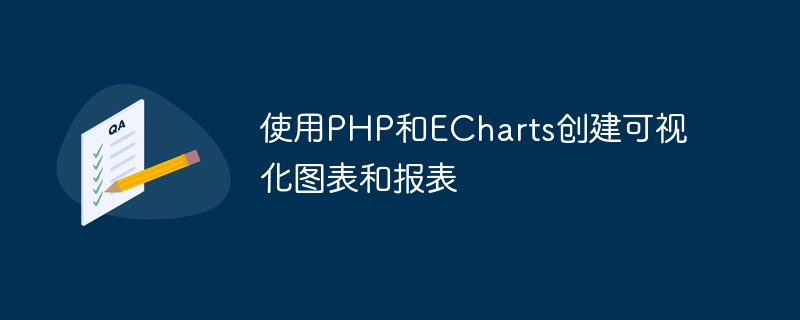
随着大数据时代的来临,数据可视化成为企业决策的重要工具。千奇百怪的数据可视化工具层出不穷,其中ECharts以其强大的功能和良好的用户体验受到了广泛的关注和应用。而PHP作为一种主流的服务器端语言,也提供了丰富的数据处理和图表展示功能。本文将介绍如何使用PHP和ECharts创建可视化图表和报表。ECharts简介ECharts是一个开源的可视化图表库,它由
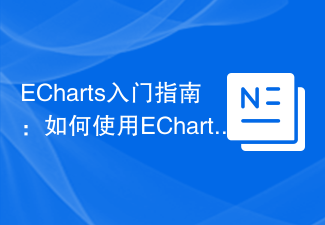
ECharts入门指南:如何使用ECharts,需要具体代码示例ECharts是一款基于JavaScript的数据可视化库,通过使用ECharts,用户可以轻松地展示各种各样的图表,如折线图、柱状图、饼图等等。本文将为您介绍如何使用ECharts,并提供详细的代码示例。安装ECharts要使用ECharts,您首先需要安装它。您可以从ECharts官网htt

一、前言前端开发需要经常使用ECharts图表渲染数据信息,在一个项目中我们经常需要使用多个图表,选择封装ECharts组件复用的方式可以减少代码量,增加开发效率。二、封装ECharts组件为什么要封装组件避免重复的工作量,提升复用性使代码逻辑更加清晰,方便项目的后期维护封装组件可以让使用者不去关心组件的内部实现以及原理,能够使一个团队更好的有层次的去运行封装的ECharts组件实现了以下的功能:使用组件传递ECharts中的option属性手动/自动设置chart尺寸chart自适应宽高动态展
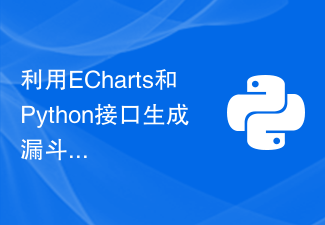
利用ECharts和Python接口生成漏斗图的步骤,需要具体代码示例漏斗图是一种常用的数据可视化工具,可以用于展示数据在不同阶段之间的变化情况。利用ECharts和Python接口,我们可以轻松地生成漂亮的漏斗图。下面,将按照以下步骤介绍如何实现漏斗图的生成,并给出具体的代码示例。步骤一:安装ECharts和Python接口首先,我们需要安装ECharts
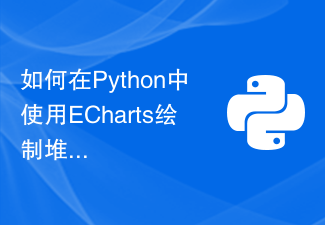
在数据可视化领域,堆叠柱状图是一种常见的可视化方式。它将多个数据系列绘制成一个条形,每个条形由多个子项组成,每个子项对应一个数据系列,在同一坐标系下进行展示。这种图表可以用于比较不同类别或数据系列的总大小、每个类别或数据系列的组成比例等。在Python中,我们可以使用ECharts库来绘制堆叠柱状图,而且该库具有丰富的可定制性和交互性。一、安装和导入ECha
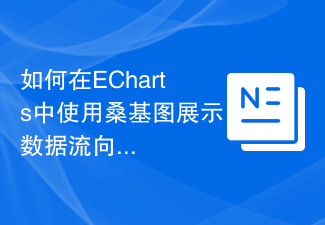
如何在ECharts中使用桑基图展示数据流向引言:数据可视化是数据分析中的重要环节,能够将复杂的数据通过图表等方式直观地展示出来。ECharts是一个功能强大的数据可视化库,支持多种图表类型,其中桑基图(SankeyDiagram)能够非常直观地展示数据的流向关系。本文将介绍如何在ECharts中使用桑基图展示数据流向,并提供具体的代码示例。引入EChar
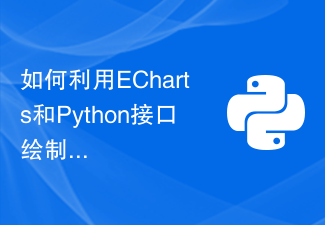
如何利用ECharts和Python接口绘制箱线图,需要具体代码示例引言:箱线图(Boxplot)是统计学中常用的一种可视化方法,用于显示实数型数据的分布情况,通过绘制数据的五数概括(最小值、下四分位数、中位数、上四分位数和最大值)以及异常值,可以直观地了解数据的偏态、离散程度和异常值情况。本文将介绍如何利用ECharts和Python接口来绘制箱线图,并


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
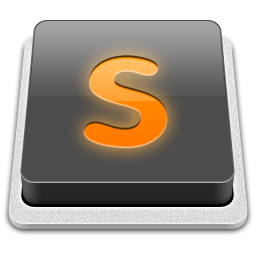
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
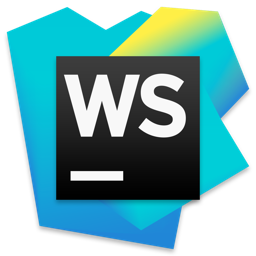
WebStorm Mac version
Useful JavaScript development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
