In modern application development, real-time data transmission has become a very important topic. Real-time data transfer means delivering data to visitors immediately as it changes. This real-time feedback can be used in a variety of applications, such as gaming, social media, and financial transactions.
In this article, we will explore ways to achieve real-time data transmission using Java and WebSocket. We will explain what WebSocket is and provide a Java-based WebSocket example that can be used for real-time data transfer.
What is WebSocket
WebSocket is a new network protocol that allows a persistent connection between a client and a server to communicate in real time when data changes. Unlike traditional HTTP requests, a WebSocket connection always remains open, which allows the server to send data to the client and the client to send data to the server at any time. WebSocket is commonly used for real-time communication, such as chat applications, real-time games, etc.
How WebSocket works
When a client wants to connect to a WebSocket server, it sends a WebSocket handshake request. This handshake request is an HTTP protocol request, which contains some specific header information. If the server accepts this handshake request, it will return a WebSocket connection confirmation indicating that the WebSocket connection has been established.
Once a WebSocket connection is established, communication between the client and server can continue until one of the parties chooses to close the connection. The client can send messages to the server at any time, and the server can send messages to the client at any time. This real-time communication makes WebSocket ideal for real-time data transfer applications.
Implementing WebSocket
In Java, implementing WebSocket requires the use of a Java library named "javax.websocket". This library provides classes and interfaces for implementing WebSocket connections and data transmission.
The following is a simple Java WebSocket implementation sample:
1. Create a WebSocket endpoint (EndPoint)
@ServerEndpoint(value = "/wsendpoint") public class WSEndpoint { @OnOpen public void onOpen(Session session) { // 处理连接建立 } @OnClose public void onClose(Session session, CloseReason closeReason) { // 处理连接断开 } @OnMessage public void onMessage(String message, Session session) { // 处理消息 } @OnError public void onError(Throwable error, Session session) { // 处理错误 } }
2. Configure the endpoint in web.xml
<websocket-endpoint> <endpoint-class>com.example.WSEndpoint</endpoint-class> </websocket-endpoint>
In this example, we create a WebSocket endpoint named "WSEndpoint". This endpoint uses 4 annotations, namely @ServerEndpoint, @OnOpen, @OnClose, @OnMessage and @OnError. These annotations are used to handle various states of WebSocket connections, including connection establishment, disconnection, message reception, and error handling.
Real-time data transmission
After creating the WebSocket endpoint, you can start to implement real-time data transmission. In this example, we will send a message called "currentDate" to the client, which contains the current time of the server. This message will be sent every second to provide real-time updates.
The following is the JavaScript code that sends the "currentDate" message:
var ws = new WebSocket("ws://localhost:8080/wsendpoint"); ws.onopen = function() { console.log("Connection opened ..."); setInterval(function() { ws.send("currentDate"); }, 1000); }; ws.onmessage = function(e) { console.log("Received message: " + e.data); };
This JavaScript code will establish a WebSocket connection and send a "currentDate" message every second. When the server sends a message, the "onmessage" callback function will be used to process the message.
The following is the server-side Java code for sending the "currentDate" message:
@ServerEndpoint(value = "/wsendpoint") public class WSEndpoint { @OnOpen public void onOpen(Session session) { System.out.println("Connection opened ..."); Timer timer = new Timer(); timer.scheduleAtFixedRate(new TimerTask() { @Override public void run() { try { DateFormat dateFormat = new SimpleDateFormat("yyyy/MM/dd HH:mm:ss"); Date date = new Date(); session.getBasicRemote().sendText(dateFormat.format(date)); } catch (IOException e) { e.printStackTrace(); } } }, 0, 1000); } @OnClose public void onClose(Session session, CloseReason closeReason) { System.out.println("Connection closed ..."); } @OnMessage public void onMessage(String message, Session session) throws IOException { if (message.equals("currentDate")) { DateFormat dateFormat = new SimpleDateFormat("yyyy/MM/dd HH:mm:ss"); Date date = new Date(); session.getBasicRemote().sendText(dateFormat.format(date)); } } @OnError public void onError(Throwable error, Session session) { error.printStackTrace(); } }
This Java code uses a Timer class that will send a message to the client every second after the WebSocket connection is established. Send a current time. When the client sends a "currentDate" message, the server will send the current time back to the client.
Conclusion
In this article, we explored how to achieve real-time data transmission using Java and WebSocket. We introduced how WebSocket works and provided an example to demonstrate how to implement real-time data transfer using Java. We hope this article helped you understand WebSocket implementation and provide some reference for your real-time applications.
The above is the detailed content of How to implement real-time data transfer using Java and WebSocket. For more information, please follow other related articles on the PHP Chinese website!
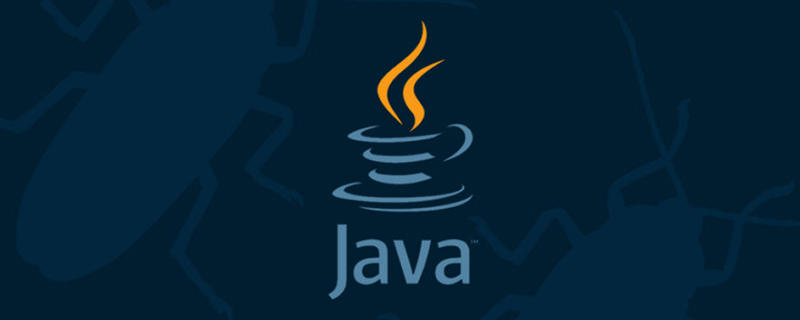
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
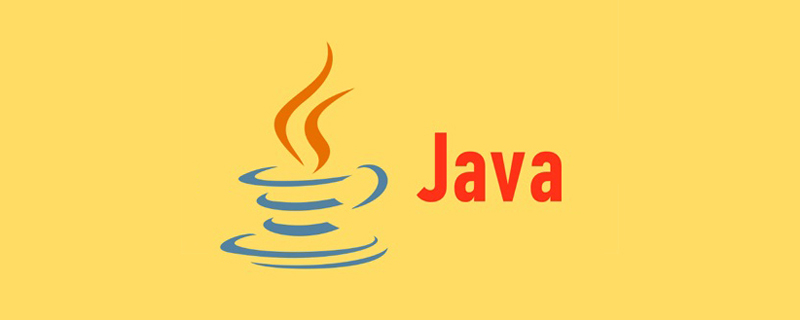
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
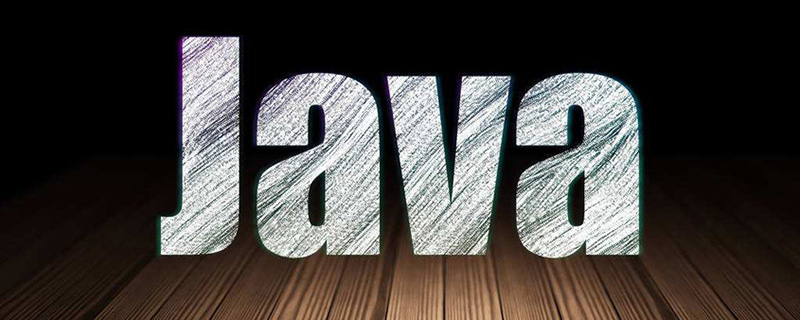
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
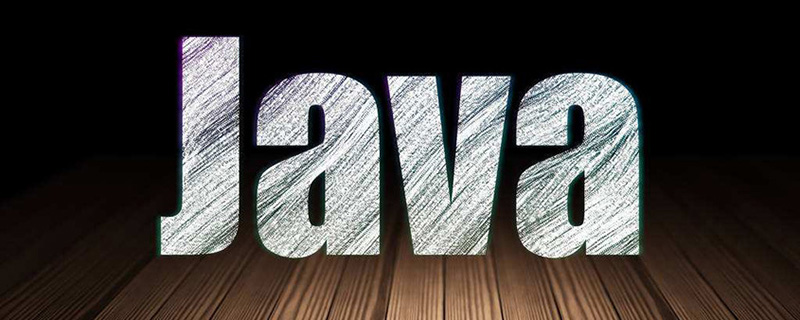
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
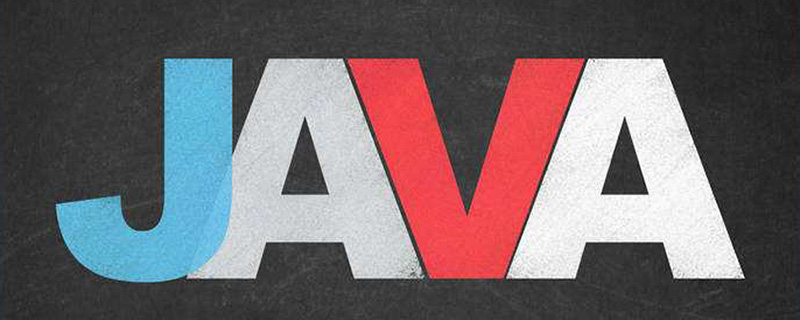
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
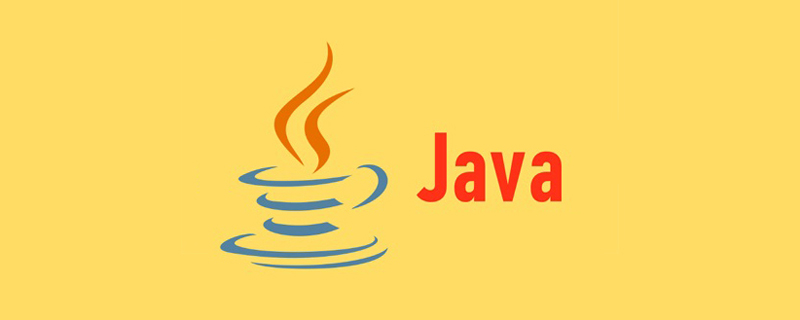
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
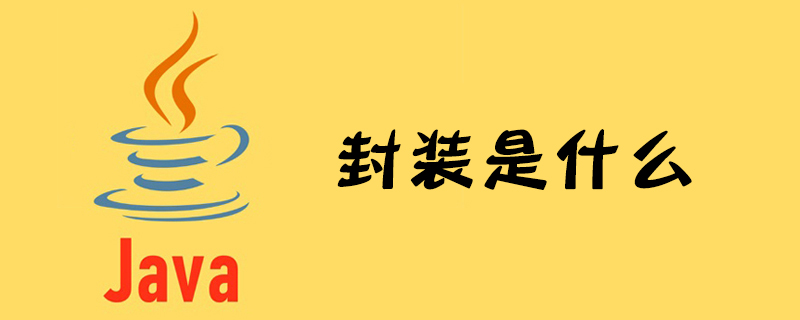
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
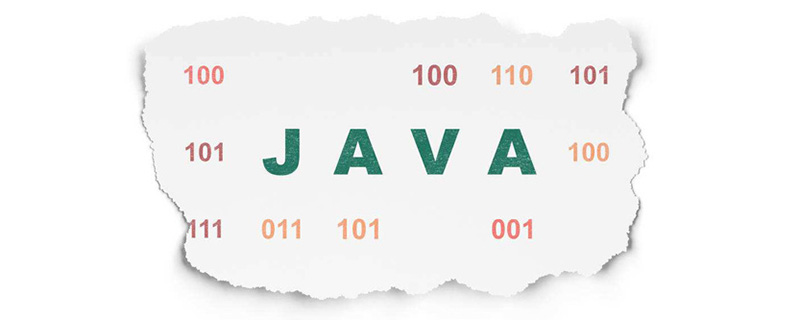
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于设计模式的相关问题,主要将装饰器模式的相关内容,指在不改变现有对象结构的情况下,动态地给该对象增加一些职责的模式,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
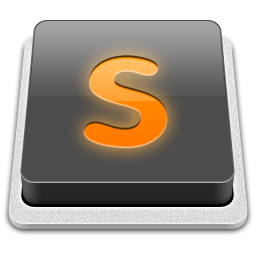
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
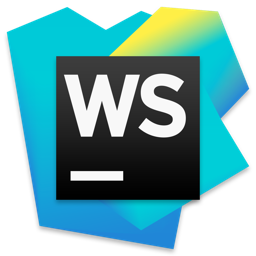
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
