


Use PHP to develop WebSocket to create real-time weather forecast function
Preface
WebSocket is a network communication protocol that can be established between the client and the server Persistent connection enables two-way real-time communication. In Web development, WebSocket is widely used in scenarios such as instant chat, real-time push, and real-time data updates. This article will introduce how to use PHP to develop WebSocket to implement real-time weather forecast function.
Step 1: Create a WebSocket server
First, we need to create a WebSocket server to handle client connections and message sending. In PHP, you can use the Ratchet library to implement the functionality of a WebSocket server.
- First, make sure Composer is installed. Composer is a dependency management tool for PHP.
- Create a new PHP project and create a new composer.json file in the project directory and add the following content:
{ "require": { "cboden/ratchet": "^0.4" } }
- In the project directory , execute the
composer install
command to install the Ratchet library. - Create a new PHP file named server.php to implement the main logic of the WebSocket server. The code example is as follows:
<?php require __DIR__ . '/vendor/autoload.php'; use RatchetMessageComponentInterface; use RatchetConnectionInterface; use RatchetServerIoServer; use RatchetHttpHttpServer; use RatchetWebSocketWsServer; class WeatherForecast implements MessageComponentInterface { public function onOpen(ConnectionInterface $conn) { // 当有新的客户端连接时,触发该方法 } public function onClose(ConnectionInterface $conn) { // 当有客户端断开连接时,触发该方法 } public function onMessage(ConnectionInterface $from, $msg) { // 当收到客户端发送的消息时,触发该方法 } public function onError(ConnectionInterface $conn, Exception $e) { // 当发生错误时,触发该方法 } public function broadcastMessage($msg) { // 向所有连接的客户端广播消息 } } $server = IoServer::factory( new HttpServer( new WsServer( new WeatherForecast() ) ), 8080 ); $server->run();
In the above code, we created a class named WeatherForecast, which implements Ratchet’s MessageComponentInterface interface, which contains various event processing methods of the WebSocket server. For example, onOpen, onClose, onMessage, etc. We can write specific logic in these methods to handle client connection, disconnection and message sending.
In the WeatherForecast class, we also define a broadcastMessage method to broadcast messages to all connected clients.
Finally, connect the three objects HttpServer, WsServer and WeatherForecast together through the factory method of IoServer, and specify the port number of the server as 8080.
Step 2: Access the weather forecast API
Next, we need to obtain real-time weather forecast data and send it to the connected client. In this article, we use a public weather forecast API, but you can also choose other APIs based on actual needs.
In the onOpen method of the WeatherForecast class, we can initiate an HTTP request to obtain weather forecast data. The code example is as follows:
public function onOpen(ConnectionInterface $conn) { $url = 'https://api.weatherapi.com/v1/current.json?key=YOUR_API_KEY&q=Beijing'; $response = file_get_contents($url); $data = json_decode($response, true); // 处理天气预报数据,比如将数据发送给客户端 }
Among them, YOUR_API_KEY needs to be replaced with your API Key, and the queried city needs to be modified according to actual needs.
Step 3: Send real-time weather forecast data
After obtaining the weather forecast data, we can send the data to the connected client through the WebSocket server.
In the onOpen method of the WeatherForecast class, we can call the broadcastMessage method to broadcast messages to all connected clients. The code example is as follows:
public function onOpen(ConnectionInterface $conn) { $url = 'https://api.weatherapi.com/v1/current.json?key=YOUR_API_KEY&q=Beijing'; $response = file_get_contents($url); $data = json_decode($response, true); $this->broadcastMessage($data['current']); }
In the above code, we use the current field of the weather forecast data as the message content and broadcast it to all connected clients through the broadcastMessage method.
In the onMessage method of the WeatherForecast class, we can process the messages sent by the client and send corresponding data according to actual needs. The code example is as follows:
public function onMessage(ConnectionInterface $from, $msg) { if ($msg === 'getWeather') { $url = 'https://api.weatherapi.com/v1/current.json?key=YOUR_API_KEY&q=Beijing'; $response = file_get_contents($url); $data = json_decode($response, true); $from->send($data['current']); } }
In the above code, when the client sends the getWeather message, we will initiate an HTTP request again to obtain the latest weather forecast data and send it to the client.
Step 4: Client access and display
Finally, we need to write client code to access and display real-time weather forecast data. In this article, we use JavaScript to implement client-side functionality.
<script> const socket = new WebSocket('ws://localhost:8080'); socket.onopen = function(event) { socket.send('getWeather'); } socket.onmessage = function(event) { const weatherData = JSON.parse(event.data); // 处理天气预报数据,比如展示在网页上 } </script>
In the above code, we create a WebSocket object and specify the server address as ws://localhost:8080. In the onopen event, we sent the getWeather message to the server to trigger the server to send real-time weather forecast data. In the onmessage event, we process the message sent by the server and display it on the web page.
Summary
By developing WebSocket in PHP, we can realize the real-time weather forecast function. Through the WebSocket server, two-way real-time communication between the client and the server can be achieved. By accessing the weather forecast API and sending real-time weather forecast data, we can send the latest weather forecast data to the connected client in a timely manner and display it on the client. This method can not only meet the needs of real-time weather forecasting, but can also be applied to other real-time data push scenarios.
The above is the detailed content of Use php to develop Websocket to create real-time weather forecast function. For more information, please follow other related articles on the PHP Chinese website!
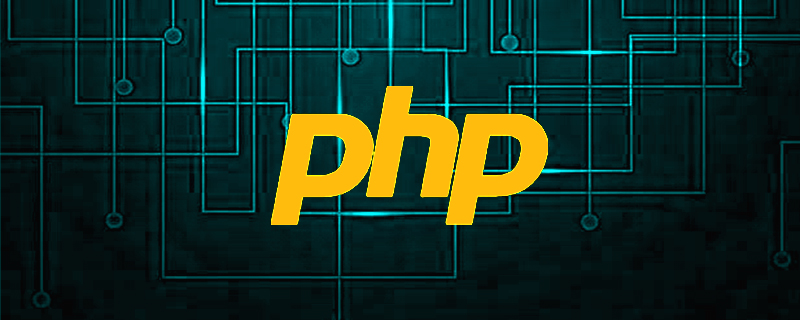
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
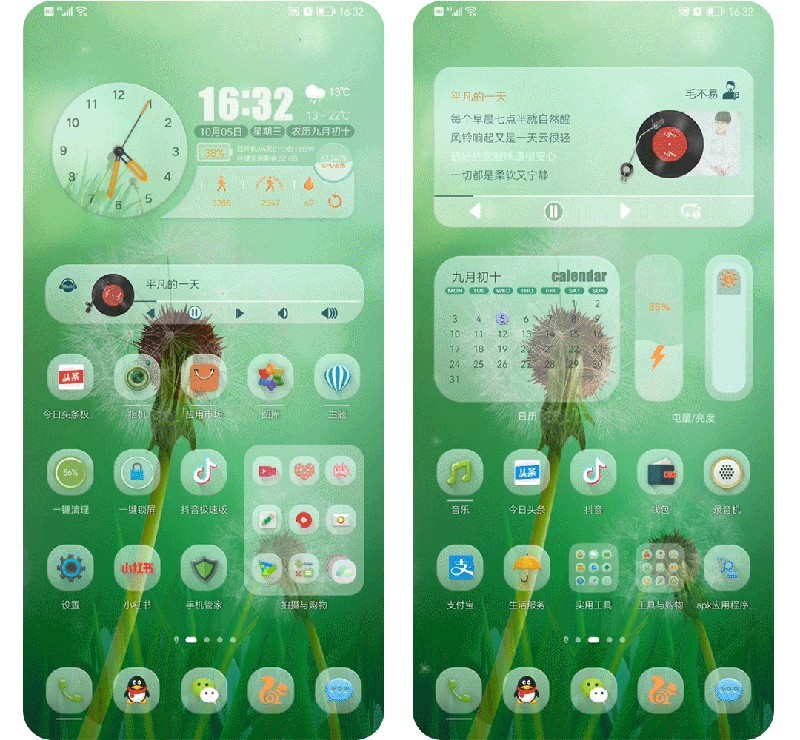
自2021年12月开始华为&荣耀手机上线了万象桌面小组件的功能。为诸多用户手机桌面添加很多便捷功能、视觉优化的桌面控件等等;到今年8月两大商家平台也开放了运动健康数据、天气数据、音乐数据、系统数据等等,让用户在手机桌面的交互操作更加方便快捷还具备较多趣味性,让用户自己DIY创意组合自己的个性桌面。添加小组件后的手机桌面最近,许多华为手机用户反映他们对于如何在华为和荣耀手机上添加桌面小组件的操作方式不太清楚,抱怨这一过程过于复杂和繁琐。为了帮助大家解决这个问题,钱舒娴准备了详细的操作流程,希望能够
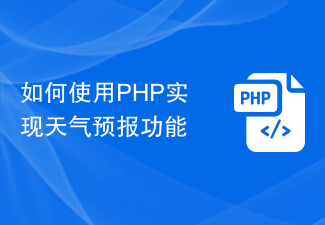
PHP作为一款流行的后端编程语言,在Web开发领域广受欢迎。天气预报功能是一种常见的Web应用场景,基于PHP实现天气预报功能相对简单易懂。本文将介绍如何使用PHP实现天气预报功能。一、获取天气数据API要实现天气预报功能,首先需要获取天气数据。我们可以使用第三方天气API来获取实时、准确的天气数据。目前,国内主流的天气API供应商包括免费的“心知天气”和收
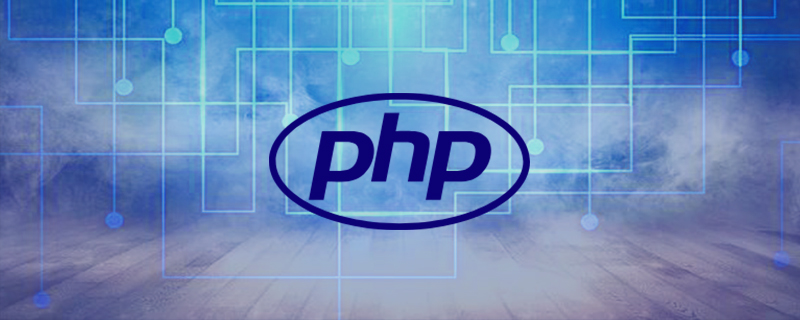
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
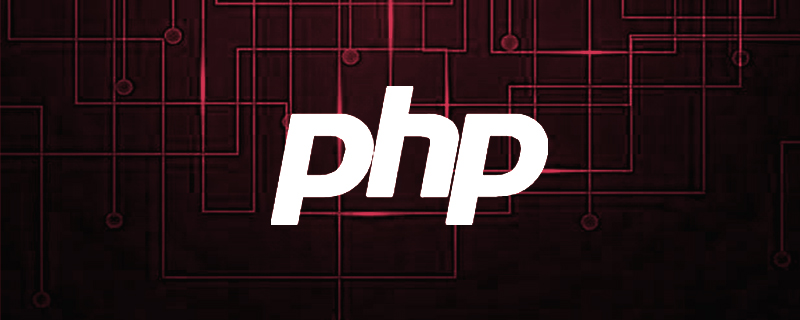
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
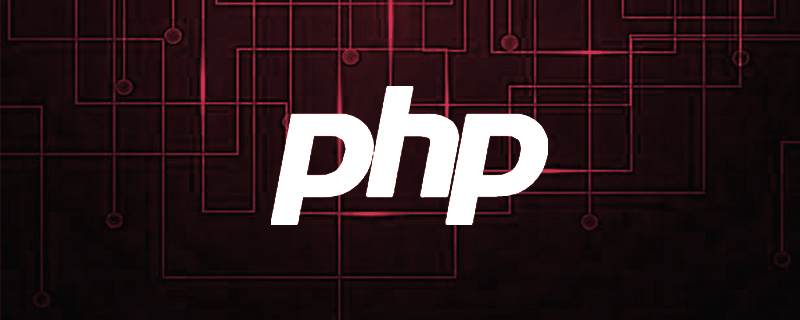
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
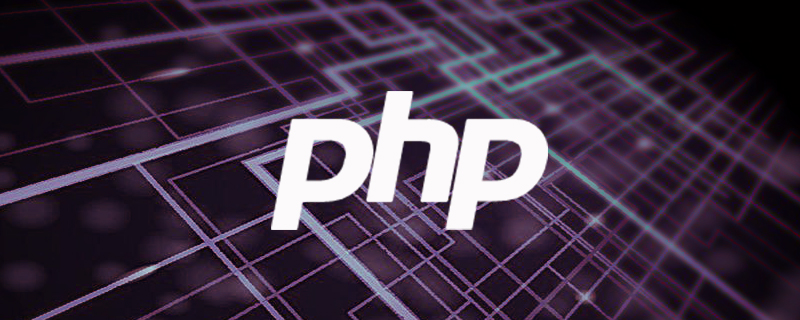
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
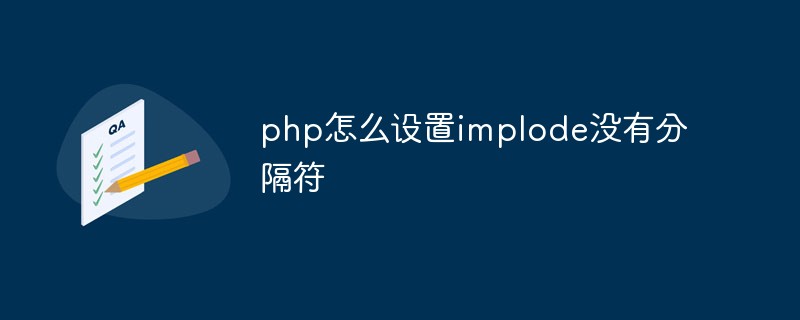
在PHP中,可以利用implode()函数的第一个参数来设置没有分隔符,该函数的第一个参数用于规定数组元素之间放置的内容,默认是空字符串,也可将第一个参数设置为空,语法为“implode(数组)”或者“implode("",数组)”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
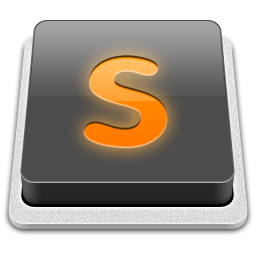
SublimeText3 Mac version
God-level code editing software (SublimeText3)
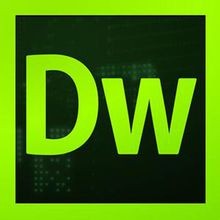
Dreamweaver CS6
Visual web development tools
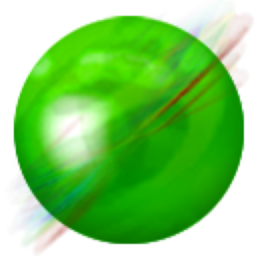
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
