Load balancing techniques for routing in Go language
Load balancing techniques for routing in Go language, specific code examples are required
In Go language, routing is an important part of building web applications. Load balancing refers to distributing traffic to multiple servers to ensure that the load of each server can be maintained within a reasonable range and improve system availability and performance. In this article, we will introduce how to implement routing load balancing in Go language and provide specific code examples.
1. What is load balancing?
Load balancing refers to a technology that distributes traffic to multiple servers to achieve load balancing and improve availability and performance. The principle is to distribute requests to different servers to reduce the load pressure on a single server, and to adjust the traffic distribution strategy according to the actual situation of the server by monitoring the status of the system.
In web applications, common load balancing algorithms include polling, random, weighted random, etc. Regardless of the algorithm, load balancing can be performed by implementing a router in the Go language.
2. Use the Gin framework to implement routing load balancing
Gin is a lightweight Web framework that provides the ability to quickly build Web applications. We can use the Gin framework to implement routing load balancing.
1. First, we need to install the Gin framework. Use the following command to install:
go get -u github.com/gin-gonic/gin
2. Next, we can write a simple load balancing router. The sample code is as follows:
package main import ( "github.com/gin-gonic/gin" ) func main() { r := gin.Default() // 定义服务器列表 servers := []string{ "http://localhost:8081", "http://localhost:8082", "http://localhost:8083", } // 轮询算法的负载均衡 index := 0 r.GET("/", func(c *gin.Context) { target := servers[index] index = (index + 1) % len(servers) c.Redirect(http.StatusMovedPermanently, target) }) r.Run(":8080") }
In the above code, we define a server list and then use the polling algorithm to achieve load balancing. Each time a request is received, the request is redirected to the next server and the index is updated to ensure polling distribution.
3. Save and run the above program. Use a browser to visit http://localhost:8080, and you can see that requests are polled and distributed to different servers.
3. Use NGINX to achieve load balancing
Another commonly used load balancing solution is to use NGINX. NGINX is a high-performance web server and reverse proxy server that can be used for load balancing and distribution of HTTP requests.
We can achieve routing load balancing by configuring NGINX.
1. First, we need to install NGINX. It can be installed through the following command:
sudo apt-get install nginx
2. Then, we need to edit the NGINX configuration file. Open the /etc/nginx/nginx.conf file and add the following configuration:
http { upstream backend { server localhost:8081; server localhost:8082; server localhost:8083; } server { listen 80; location / { proxy_pass http://backend; } } }
In the above configuration, we defined an upstream server group named backend and specified three servers. In the server block, the proxy_pass directive is used to forward the request to the backend server group.
3. Save and close the configuration file, and then restart the NGINX service:
sudo systemctl restart nginx
4. Now, open the browser and visit http://localhost, you can see that the request is load balanced by NGINX to a different server.
Summary
This article introduces how to implement routing load balancing in Go language, and provides specific code examples using the Gin framework and NGINX. Load balancing can improve the availability and performance of the system and ensure that the load of each server is within a reasonable range. Whether you use the Go language framework or NGINX, you can achieve routing load balancing based on different load balancing algorithms.
The above is the detailed content of Load balancing techniques for routing in Go language. For more information, please follow other related articles on the PHP Chinese website!
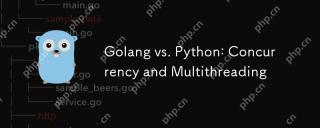
Golang is more suitable for high concurrency tasks, while Python has more advantages in flexibility. 1.Golang efficiently handles concurrency through goroutine and channel. 2. Python relies on threading and asyncio, which is affected by GIL, but provides multiple concurrency methods. The choice should be based on specific needs.
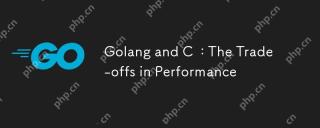
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
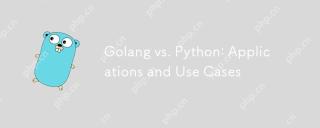
ChooseGolangforhighperformanceandconcurrency,idealforbackendservicesandnetworkprogramming;selectPythonforrapiddevelopment,datascience,andmachinelearningduetoitsversatilityandextensivelibraries.

Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
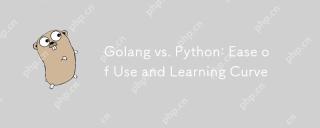
In what aspects are Golang and Python easier to use and have a smoother learning curve? Golang is more suitable for high concurrency and high performance needs, and the learning curve is relatively gentle for developers with C language background. Python is more suitable for data science and rapid prototyping, and the learning curve is very smooth for beginners.
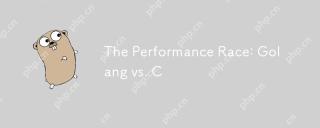
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
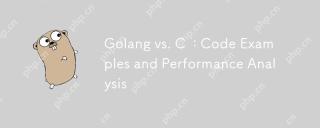
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
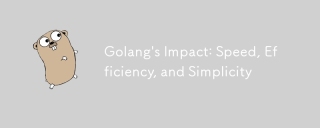
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool