


How to implement an online question and answer system using WebSocket and JavaScript
WebSocket is a full-duplex communication protocol based on the TCP protocol, which is widely used in Web development to implement real-time communication functions. In this article, we will introduce how to use WebSocket and JavaScript to implement a simple online question and answer system.
- Writing HTML page
We first write a basic HTML template and introduce the JavaScript library required by WebSocket into it.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>在线问答系统</title> </head> <body> <div id="messages"></div> <form id="message-form"> <input type="text" id="message-input" placeholder="请输入您的问题..."> <button type="submit">提交</button> </form> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="websocket.js"></script> </body> </html>
- Writing WebSocket code
We store the WebSocket-related JavaScript code in a separate file. In this file, we need to define several variables and functions and establish a WebSocket connection so that the server can send messages to the client.
// 声明变量 const serverUrl = 'ws://localhost:8080'; // WebSocket服务器地址 const socket = new WebSocket(serverUrl); // WebSocket连接 // 监听WebSocket事件 socket.onopen = function(event) { console.log('[WebSocket] 连接成功', event); }; socket.onclose = function(event) { console.log('[WebSocket] 连接关闭', event); }; socket.onerror = function(event) { console.error('[WebSocket] 发生错误', event); }; socket.onmessage = function(event) { console.log('[WebSocket] 收到消息', event); showMessage(event.data); }; // 发送消息到WebSocket服务器 function sendMessage(message) { socket.send(message); } // 显示消息 function showMessage(message) { $('#messages').append($('<p>').text(`机器人:${message}`)); } // 提交问题 $('#message-form').submit(function(event) { event.preventDefault(); const messageInput = $('#message-input'); const message = messageInput.val(); messageInput.val(''); $('#messages').append($('<p>').text(`你:${message}`)); sendMessage(message); });
- Writing server code
Finally, we need to write server-side code. In this article, we will use Node.js as the development environment of the server and use the ws library to provide the WebSocket service.
const WebSocketServer = require('ws').Server; const server = new WebSocketServer({ port: 8080 }); console.log('WebSocket服务器已启动'); // 监听WebSocket连接事件 server.on('connection', function(socket) { console.log('WebSocket连接已建立'); // 监听WebSocket消息事件 socket.on('message', function(message) { console.log('收到消息', message); const response = handleQuestion(message); socket.send(response); }); // 监听WebSocket关闭事件 socket.on('close', function() { console.log('WebSocket连接已关闭'); }); }); // 处理问题 function handleQuestion(question) { // TODO: 添加问题处理逻辑 return '这是机器人的回答'; }
In the above code, we create a WebSocketServer object and listen to the connection, message and close events on it. When receiving a message sent by the client, the server will call the handleQuestion function to process it, and then send the reply back to the client.
- Testing the online question and answer system
Now, we open two browser windows, one acting as the client and the other acting as the server. Enter your question in the client browser and click the submit button. The server will reply with a message indicating the robot's answer and display it in the client browser.
So far, we have successfully implemented a simple online question and answer system. Of course, this is just a basic example and you can extend and optimize it according to your own needs.
The above is the detailed content of How to implement an online question and answer system using WebSocket and JavaScript. For more information, please follow other related articles on the PHP Chinese website!
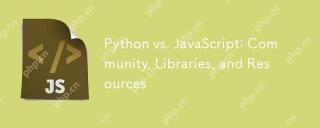
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
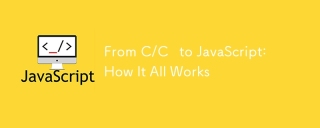
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
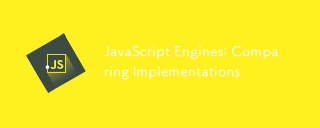
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
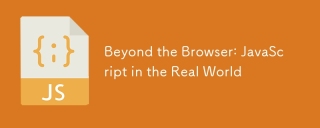
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
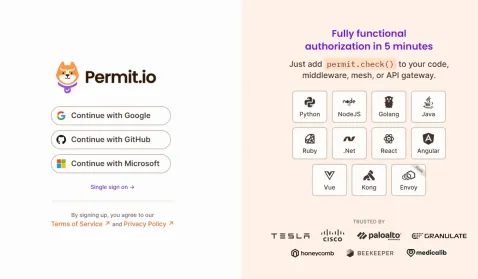
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
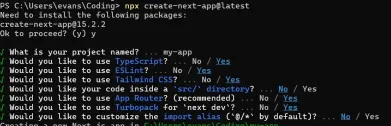
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
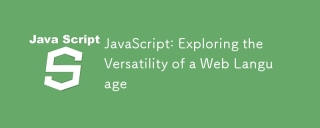
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
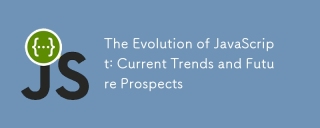
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
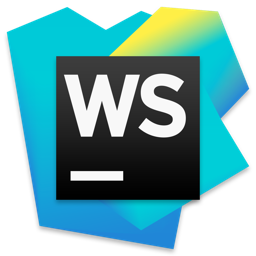
WebStorm Mac version
Useful JavaScript development tools
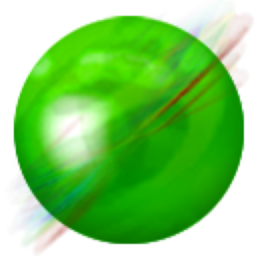
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment