The steps for java to call webservice are to generate client code, create the client and run the client. In addition, in a production environment, various abnormal situations, performance optimization, call logging, etc. of web services also need to be considered. If you need a deeper understanding, it is recommended to refer to the documentation and sample code of different frameworks, as well as related best practices.
To call Web services in Java, you can use JAX-WS (Java API for XML Web Services) provided by Java or Frameworks such as Apache CXF are implemented. In the following answer, I will limit myself to using JAX-WS to call web services. The following are the basic steps:
Step 1: Generate client code
First, you need to generate the client based on the WSDL file (Web Services Description Language) of the Web service code. can use The wsimport tool generates Java classes from WSDL files.
wsimport -s src -d bin http://example.com/yourWebService?wsdl
The above command will generate client code and save the generated class file in the specified directory (src).
Step 2: Create the client
Next, you need to write a Java client to call the web service. Here is a simple example:
import javax.xml.namespace.QName; import javax.xml.ws.Service; import java.net.URL; public class WebServiceClient { public static void main(String[] args) throws Exception { URL url = new URL("http://example.com/yourWebService?wsdl"); QName qname = new QName("http://example.com/", "YourWebServiceName"); Service service = Service.create(url, qname); YourWebServiceInterface port = service.getPort(YourWebServiceInterface.class); // 调用 Web 服务的方法 String result = port.yourMethod(); System.out.println(result); } }
In the above code, you need to replace http://example.com/yourWebService?wsdl with your actual web service address and replace Replace YourWebServiceName with the name of your web service. YourWebServiceInterface is based on WSDL File generated client interface.
Step 3: Run the client
Compile and run the above client code, you will be able to see that it calls the web service and prints the return result.
It should be noted that the examples mentioned above are the most basic Web Service calling method. But in real applications, you may need to handle exceptions, use parameters, etc. In addition, there are some advanced features such as security, transaction management, etc., which need to be considered and implemented according to your actual needs.
In addition, in a production environment, you also need to consider Web Various abnormal situations of services, performance optimization, call logging, etc. If you need a deeper understanding, it is recommended to refer to the documentation and sample code of different frameworks, as well as related best practices.
The above is the detailed content of java method of calling webservice. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
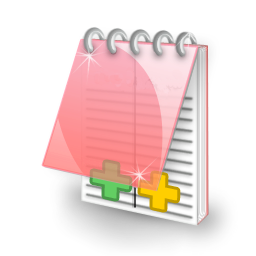
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
