Go Language Websocket Development Guide: To solve common errors, specific code examples are needed
In today's Internet applications, real-time communication has become a key technical requirement. As a two-way communication protocol, Websocket is widely used in real-time communication scenarios. In the Go language, using Websocket for development is also an important skill.
However, although the Go language provides many powerful libraries for Websocket development, there are still some common errors encountered during the development process. This article will explain these common errors and provide specific code examples to solve the problems.
First of all, a common mistake is not handling the closing of the Websocket connection correctly. In the process of using Websocket, sometimes the connection is closed unexpectedly, such as when the client is disconnected, the server is restarted, etc. If we do not handle this situation correctly, it may cause the program to crash or cause other problems.
In order to solve this problem, we can add an error type channel to the code to receive error information about closing the connection. The specific method is as follows:
func main() { // 创建一个Websocket服务器 server := websocket.NewServer() // 处理连接关闭的错误 go func() { err := <-server.Error() fmt.Println("连接关闭:", err) // 其他处理逻辑 }() // 启动服务器 server.Run(":8000") }
By starting a goroutine in the main function to receive server.Error() information, we can know the connection closing error in real time. This ensures the stability of the program.
Another common error is when sending messages. In Websocket communication, sometimes the message sent may fail to be sent due to network problems or other reasons. If we do not handle this error correctly, it may result in message loss or program exception.
In order to solve this problem, we can add a select structure before sending the message, and one of the cases is used to process the result of sending the message. The specific method is as follows:
err := conn.WriteMessage(websocket.TextMessage, []byte("Hello, WebSocket!")) if err != nil { if websocket.IsCloseError(err, websocket.CloseNormalClosure) { fmt.Println("连接已关闭") return } fmt.Println("发送消息失败:", err) }
By judging the error returned by WriteMessage, we can know whether the message was sent successfully. For connection closing errors, we can return directly to avoid subsequent operations. For other types of errors, we can handle them on a case-by-case basis.
In addition to the above two common errors, there are some other errors that also require our attention and resolution. For example, when using Websocket, you may sometimes encounter cross-domain access problems. The solution to this problem can be to add relevant cross-domain configuration on the server side, or make some special front-end settings on the client side.
To sum up, you may encounter some common errors in Go language Websocket development, but we can solve these problems through some simple methods. In future work, we should pay attention to and master these methods and improve our development capabilities.
We hope that the specific code examples provided in this article can help readers better understand and solve common errors in Websocket development. I wish you all the best in your Go language Websocket development!
The above is the detailed content of Go Language Websocket Development Guide: Solving Common Mistakes. For more information, please follow other related articles on the PHP Chinese website!
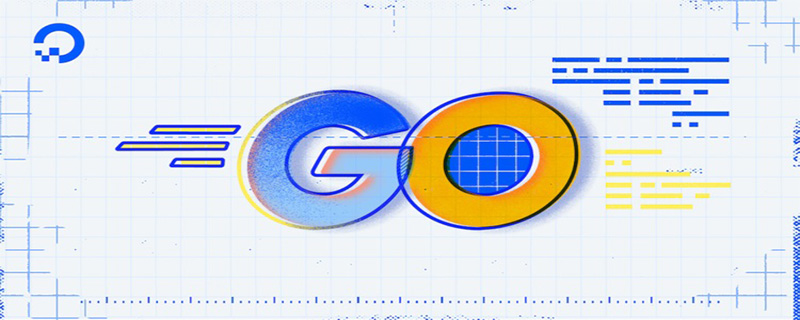
go语言有缩进。在go语言中,缩进直接使用gofmt工具格式化即可(gofmt使用tab进行缩进);gofmt工具会以标准样式的缩进和垂直对齐方式对源代码进行格式化,甚至必要情况下注释也会重新格式化。
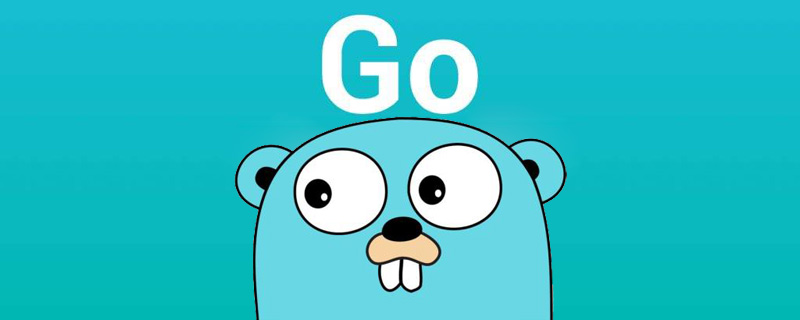
go语言叫go的原因:想表达这门语言的运行速度、开发速度、学习速度(develop)都像gopher一样快。gopher是一种生活在加拿大的小动物,go的吉祥物就是这个小动物,它的中文名叫做囊地鼠,它们最大的特点就是挖洞速度特别快,当然可能不止是挖洞啦。
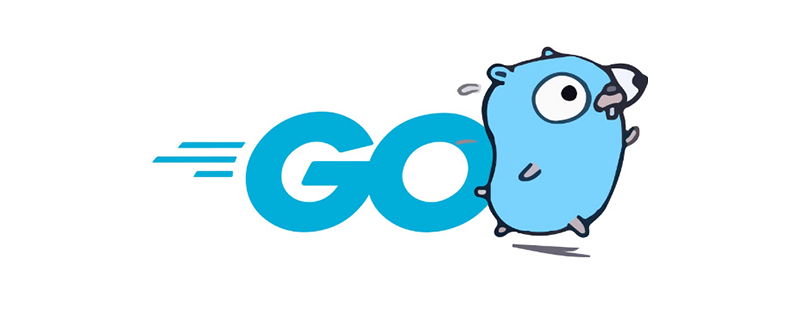
go语言需要编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言,也就说Go语言程序在运行之前需要通过编译器生成二进制机器码(二进制的可执行文件),随后二进制文件才能在目标机器上运行。
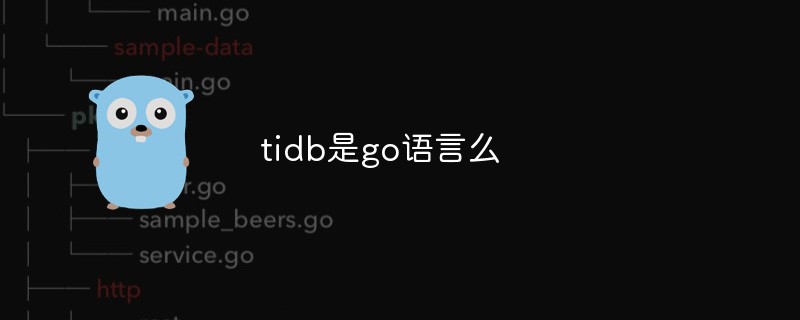
是,TiDB采用go语言编写。TiDB是一个分布式NewSQL数据库;它支持水平弹性扩展、ACID事务、标准SQL、MySQL语法和MySQL协议,具有数据强一致的高可用特性。TiDB架构中的PD储存了集群的元信息,如key在哪个TiKV节点;PD还负责集群的负载均衡以及数据分片等。PD通过内嵌etcd来支持数据分布和容错;PD采用go语言编写。
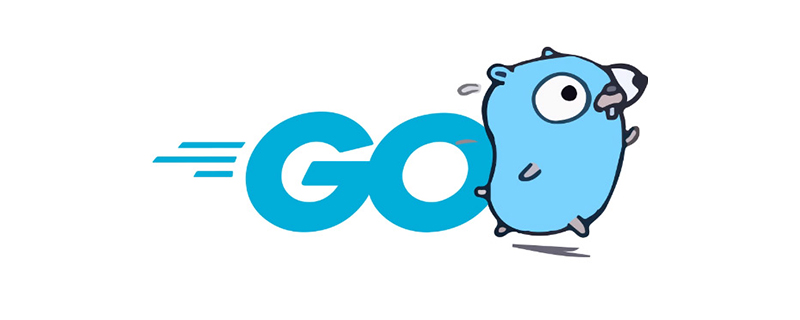
go语言能编译。Go语言是编译型的静态语言,是一门需要编译才能运行的编程语言。对Go语言程序进行编译的命令有两种:1、“go build”命令,可以将Go语言程序代码编译成二进制的可执行文件,但该二进制文件需要手动运行;2、“go run”命令,会在编译后直接运行Go语言程序,编译过程中会产生一个临时文件,但不会生成可执行文件。
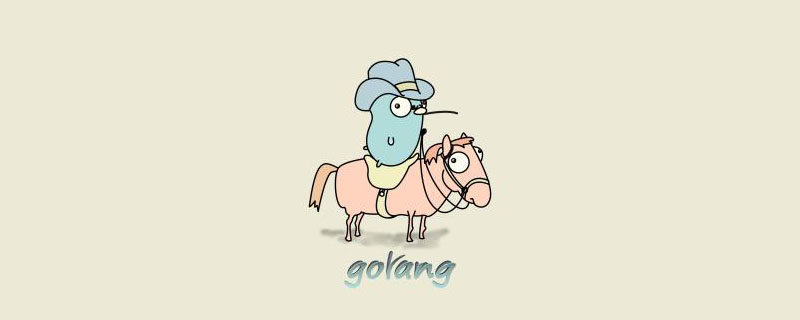
删除map元素的两种方法:1、使用delete()函数从map中删除指定键值对,语法“delete(map, 键名)”;2、重新创建一个新的map对象,可以清空map中的所有元素,语法“var mapname map[keytype]valuetype”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use
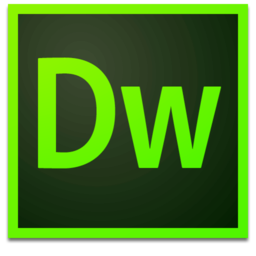
Dreamweaver Mac version
Visual web development tools
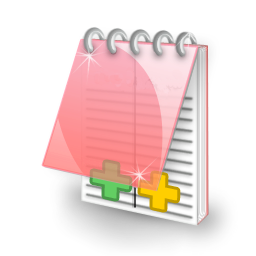
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.