C language qsort function algorithm performance test
qsort is a sorting function in the C language standard library. Its performance (that is, its running speed) mainly depends on the number of comparisons and exchanges of elements. The number of comparisons and exchanges of elements depends mainly on the size and distribution of the input array.
The following is an example of a simple qsort performance test. You can copy this code and run it to see the results:
#include <stdio.h> #include <stdlib.h> #include <time.h> // 定义一个比较函数,用于 qsort int compare(const void* a, const void* b) { return (*(int*)a - *(int*)b); } int main() { // 生成一个随机数组 srand(time(0)); int n = 1000000; // 数组大小,你可以根据需要调整这个数值 int* arr = (int*)malloc(n * sizeof(int)); for(int i = 0; i < n; i++) { arr[i] = rand(); } // 记录开始时间 clock_t start = clock(); // 使用 qsort 对数组进行排序 qsort(arr, n, sizeof(int), compare); // 记录结束时间 clock_t end = clock(); // 输出运行时间(以毫秒为单位) printf("Sorting %d elements took %f milliseconds\n", n, ((double)end - start) * 1000 / CLOCKS_PER_SEC); // 释放内存 free(arr); return 0; }
Please note that this test is only to provide an approximate performance indicator , does not guarantee the same results in all cases. Actual performance will be affected by many factors, including hardware performance, operating system scheduling, memory access patterns, etc. If you need more precise performance testing, you may need to use specialized performance analysis tools such as gprof, Valgrind's callgrind, kcachegrind, etc.
The above is the detailed content of C language qsort function algorithm performance test. For more information, please follow other related articles on the PHP Chinese website!
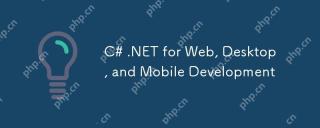
C# and .NET are suitable for web, desktop and mobile development. 1) In web development, ASP.NETCore supports cross-platform development. 2) Desktop development uses WPF and WinForms, which are suitable for different needs. 3) Mobile development realizes cross-platform applications through Xamarin.
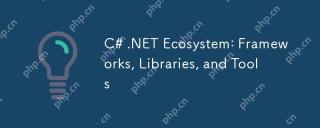
The C#.NET ecosystem provides rich frameworks and libraries to help developers build applications efficiently. 1.ASP.NETCore is used to build high-performance web applications, 2.EntityFrameworkCore is used for database operations. By understanding the use and best practices of these tools, developers can improve the quality and performance of their applications.

How to deploy a C# .NET app to Azure or AWS? The answer is to use AzureAppService and AWSElasticBeanstalk. 1. On Azure, automate deployment using AzureAppService and AzurePipelines. 2. On AWS, use Amazon ElasticBeanstalk and AWSLambda to implement deployment and serverless compute.
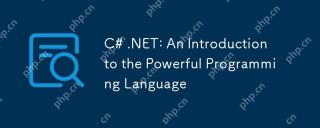
The combination of C# and .NET provides developers with a powerful programming environment. 1) C# supports polymorphism and asynchronous programming, 2) .NET provides cross-platform capabilities and concurrent processing mechanisms, which makes them widely used in desktop, web and mobile application development.
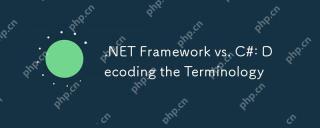
.NETFramework is a software framework, and C# is a programming language. 1..NETFramework provides libraries and services, supporting desktop, web and mobile application development. 2.C# is designed for .NETFramework and supports modern programming functions. 3..NETFramework manages code execution through CLR, and the C# code is compiled into IL and runs by CLR. 4. Use .NETFramework to quickly develop applications, and C# provides advanced functions such as LINQ. 5. Common errors include type conversion and asynchronous programming deadlocks. VisualStudio tools are required for debugging.
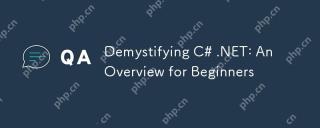
C# is a modern, object-oriented programming language developed by Microsoft, and .NET is a development framework provided by Microsoft. C# combines the performance of C and the simplicity of Java, and is suitable for building various applications. The .NET framework supports multiple languages, provides garbage collection mechanisms, and simplifies memory management.
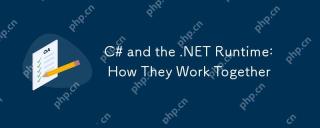
C# and .NET runtime work closely together to empower developers to efficient, powerful and cross-platform development capabilities. 1) C# is a type-safe and object-oriented programming language designed to integrate seamlessly with the .NET framework. 2) The .NET runtime manages the execution of C# code, provides garbage collection, type safety and other services, and ensures efficient and cross-platform operation.
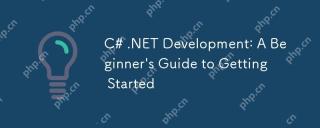
To start C#.NET development, you need to: 1. Understand the basic knowledge of C# and the core concepts of the .NET framework; 2. Master the basic concepts of variables, data types, control structures, functions and classes; 3. Learn advanced features of C#, such as LINQ and asynchronous programming; 4. Be familiar with debugging techniques and performance optimization methods for common errors. With these steps, you can gradually penetrate the world of C#.NET and write efficient applications.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Atom editor mac version download
The most popular open source editor
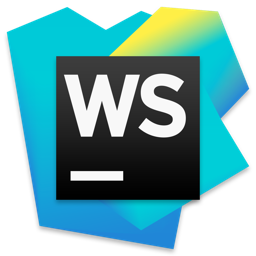
WebStorm Mac version
Useful JavaScript development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
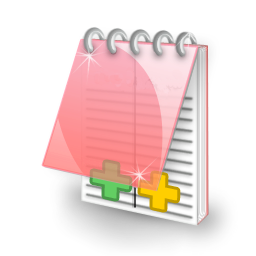
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
