Master regular expressions and string processing in Go language
Go language, as a modern programming language, provides powerful regular expressions and string processing functions, allowing developers to process string data more efficiently. It is very important for developers to master regular expressions and string processing in Go language. This article will introduce in detail the basic concepts and usage of regular expressions in Go language, and how to use Go language to process strings.
1. Regular expressions
Regular expression is a tool used to describe string patterns, which can easily implement operations such as string matching, search, and replacement. In the Go language, the regexp package is used to support regular expression operations.
- Basic syntax of regular expressions
In Go language, regular expressions consist of ordinary characters and special characters. Ordinary characters can be letters, numbers or other general symbols, while special characters represent some specific meanings. The following are some common regular expression special characters:
- . Represents any single character, except newline characters;
- indicates matching the previous character Zero or more times;
- means matching the previous character one or more times;
- ? means matching the previous character character zero or once;
- ^ means matching the beginning of the string;
- $ means matching the end of the string;
- [] is used to specify a character set, Matches any one of the characters;
- | represents an OR operation, matching the expression to its left or right;
- () is used to group subexpressions.
- Use of regular expressions
In the Go language, you can use the functions in the regexp package to process regular expressions. Commonly used functions include: - MatchString(pattern, str): Determine whether the string str matches the regular expression pattern;
- FindString(pattern, str): Return the first string str substrings that match the regular expression pattern;
- FindAllString(pattern, str, n): Returns all substrings in the string str that match the regular expression pattern, and returns at most n results.
The following is a simple example that demonstrates how to use regular expressions to determine whether a string is a legal email address:
package main import ( "fmt" "regexp" ) func main() { email := "test@email.com" pattern := `^[a-zA-Z0-9]+@[a-zA-Z0-9]+.[a-zA-Z0-9]+$` matched, _ := regexp.MatchString(pattern, email) if matched { fmt.Println("Valid email address") } else { fmt.Println("Invalid email address") } }
2. String processing
Go The language has built-in rich string processing functions, making string processing more convenient.
- String interception
String slicing (slice) can be used in the Go language to implement string interception operations. The slicing operation uses the form[start:end]
, which means intercepting the string from start to end-1. For example:
package main import "fmt" func main() { str := "Hello, World!" substr := str[0:5] fmt.Println(substr) // 输出:Hello }
- String splicing
The
package main import "fmt" func main() { str1 := "Hello" str2 := "World!" str := str1 + ", " + str2 fmt.Println(str) // 输出:Hello, World! }
- String search and replacement
In Go language, you can use the functions in the strings package to implement string search and replace operations. Commonly used functions include:
- Contains(str, substr): determine whether the string str contains substr;
- Index(str, substr): return the string str Index of the first occurrence of substr;
- Replace(str, old, new, n): Replace the first n olds in the string str with new.
The following is a sample code that demonstrates the use of string functions to implement character search and replacement:
package main import ( "fmt" "strings" ) func main() { str := "Hello, World!" contains := strings.Contains(str, "Hello") fmt.Println(contains) // 输出:true index := strings.Index(str, "World") fmt.Println(index) // 输出:7 newStr := strings.Replace(str, "Hello", "Hola", 1) fmt.Println(newStr) // 输出:Hola, World! }
3. Conclusion
In the Go language, master regular expressions and String processing is a very important skill. Through the flexible application of regular expressions, we can quickly and accurately match, find, and replace strings. The related functions of string processing can help us perform string interception, splicing and other operations more conveniently. I hope this article will be helpful to everyone in mastering regular expressions and string processing in Go language.
The above is the detailed content of Master regular expressions and string processing in Go language. For more information, please follow other related articles on the PHP Chinese website!
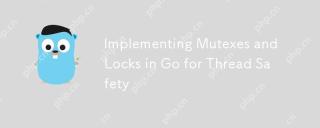
In Go, using mutexes and locks is the key to ensuring thread safety. 1) Use sync.Mutex for mutually exclusive access, 2) Use sync.RWMutex for read and write operations, 3) Use atomic operations for performance optimization. Mastering these tools and their usage skills is essential to writing efficient and reliable concurrent programs.
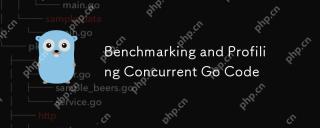
How to optimize the performance of concurrent Go code? Use Go's built-in tools such as getest, gobench, and pprof for benchmarking and performance analysis. 1) Use the testing package to write benchmarks to evaluate the execution speed of concurrent functions. 2) Use the pprof tool to perform performance analysis and identify bottlenecks in the program. 3) Adjust the garbage collection settings to reduce its impact on performance. 4) Optimize channel operation and limit the number of goroutines to improve efficiency. Through continuous benchmarking and performance analysis, the performance of concurrent Go code can be effectively improved.
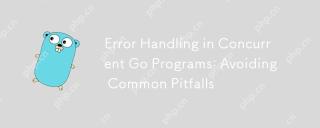
The common pitfalls of error handling in concurrent Go programs include: 1. Ensure error propagation, 2. Processing timeout, 3. Aggregation errors, 4. Use context management, 5. Error wrapping, 6. Logging, 7. Testing. These strategies help to effectively handle errors in concurrent environments.
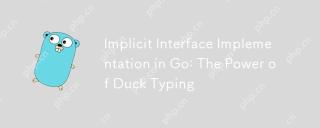
ImplicitinterfaceimplementationinGoembodiesducktypingbyallowingtypestosatisfyinterfaceswithoutexplicitdeclaration.1)Itpromotesflexibilityandmodularitybyfocusingonbehavior.2)Challengesincludeupdatingmethodsignaturesandtrackingimplementations.3)Toolsli
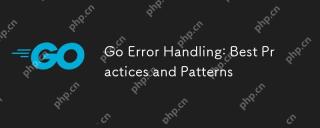
In Go programming, ways to effectively manage errors include: 1) using error values instead of exceptions, 2) using error wrapping techniques, 3) defining custom error types, 4) reusing error values for performance, 5) using panic and recovery with caution, 6) ensuring that error messages are clear and consistent, 7) recording error handling strategies, 8) treating errors as first-class citizens, 9) using error channels to handle asynchronous errors. These practices and patterns help write more robust, maintainable and efficient code.
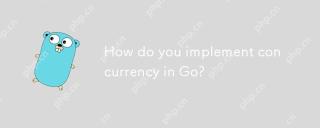
Implementing concurrency in Go can be achieved by using goroutines and channels. 1) Use goroutines to perform tasks in parallel, such as enjoying music and observing friends at the same time in the example. 2) Securely transfer data between goroutines through channels, such as producer and consumer models. 3) Avoid excessive use of goroutines and deadlocks, and design the system reasonably to optimize concurrent programs.
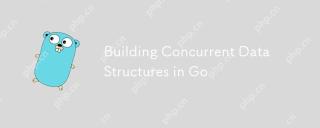
Gooffersmultipleapproachesforbuildingconcurrentdatastructures,includingmutexes,channels,andatomicoperations.1)Mutexesprovidesimplethreadsafetybutcancauseperformancebottlenecks.2)Channelsofferscalabilitybutmayblockiffullorempty.3)Atomicoperationsareef
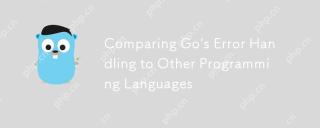
Go'serrorhandlingisexplicit,treatingerrorsasreturnedvaluesratherthanexceptions,unlikePythonandJava.1)Go'sapproachensureserrorawarenessbutcanleadtoverbosecode.2)PythonandJavauseexceptionsforcleanercodebutmaymisserrors.3)Go'smethodpromotesrobustnessand


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Atom editor mac version download
The most popular open source editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
