Vue component development: multi-level linkage selector implementation
Vue component development: multi-level linkage selector implementation
In front-end development, multi-level linkage selector is a common requirement, such as province and city selection, Year, month, day selection, etc. This article will introduce how to use Vue components to implement multi-level linkage selectors, with specific code implementation examples.
How to implement multi-level linkage selector?
To implement multi-level linkage selectors, you need to use Vue's component development idea to split a large selector into several sub-components, which are responsible for rendering each level of options. Each time the level selection changes, the options of subsequent levels must be updated, which requires the use of a communication mechanism between Vue components.
In addition, the selector needs to receive the initial value from the outside and send event notifications to the outside when the selection changes. This can be achieved using props and $emit.
Let’s gradually implement this multi-level linkage selector component.
Step 1: Define subcomponents
We first define the selector subcomponent for each level. The following is the code for a simple subcomponent of the province selector:
<template> <select v-model="selected"> <option value="">请选择</option> <option v-for="item in options" :value="item">{{ item }}</option> </select> </template> <script> export default { props: { options: { type: Array, required: true }, value: { type: String, default: '' } }, data() { return { selected: this.value } }, watch: { selected(newValue) { this.$emit('change', newValue) } } } </script>
Code explanation:
- Use the select tag to render the drop-down option box, and use v-model to bind the current option The value of , and initialize the selected value through data();
- Use watch to monitor changes in the selected value, send a change event when the option changes, and notify the parent component of the new selected value.
- Step 2: Define the parent component
- Next, we define the parent component of the multi-level linkage selector. This component is responsible for rendering all child components and updating the options of subsequent child components when the options change.
The following is the code for a simple two-level linkage selector:
<template> <div> <CitySelect :options="provinces" v-model="selectedProvince"/> <CitySelect :options="cities" v-model="selectedCity"/> </div> </template> <script> import CitySelect from './CitySelect.vue' export default { components: { CitySelect }, data() { return { provinces: ['广东', '江苏', '浙江'], cities: { '广东': ['广州', '深圳'], '江苏': ['南京', '苏州'], '浙江': ['杭州', '宁波'] }, selectedProvince: '', selectedCity: '' } }, watch: { selectedProvince(newValue) { this.selectedCity = '' if (newValue) { this.cities = this.$data.cities[newValue] } else { this.cities = [] } } } } </script>
Code explanation:
Use two CitySelect subcomponents in the template and render them separately. The province and city selection boxes bind the currently selected provinces and cities through v-model; Define two arrays, provinces and cities, in the data function. The provinces array stores all provinces, and the cities object stores all Cities, use selectedProvince and selectedCity to record the currently selected provinces and cities;- Monitor changes in selectedProvince in the watch, and update the cities array when the province changes, which is used to render the next-level city selection box.
- Step 3: Combine all sub-components
- After we have defined all sub-components and parent components, we only need to combine all sub-components to form a complete The multi-level linkage selector.
The following is the code for a simple three-level linkage selector:
<template> <div> <RegionSelect :options="provinces" v-model="selectedProvince"/> <RegionSelect :options="cities" v-model="selectedCity"/> <RegionSelect :options="districts" v-model="selectedDistrict"/> </div> </template> <script> import RegionSelect from './RegionSelect.vue' export default { components: { RegionSelect }, data() { return { provinces: ['广东', '江苏', '浙江'], cities: { '广东': ['广州', '深圳'], '江苏': ['南京', '苏州'], '浙江': ['杭州', '宁波'] }, districts: { '广州': ['天河区', '海珠区'], '深圳': ['福田区', '南山区'], '南京': ['玄武区', '鼓楼区'], '苏州': ['姑苏区', '相城区'], '杭州': ['上城区', '下城区'], '宁波': ['江东区', '江北区'] }, selectedProvince: '', selectedCity: '', selectedDistrict: '' } }, watch: { selectedProvince(newValue) { if (newValue) { this.cities = this.$data.cities[newValue] this.selectedCity = '' this.districts = [] } else { this.cities = [] this.districts = [] } }, selectedCity(newValue) { if (newValue) { this.districts = this.$data.districts[newValue] this.selectedDistrict = '' } else { this.districts = [] } } } } </script>
Code explanation:
Use three RegionSelect subcomponents in the template and render them separately. The selection boxes for provinces, cities and districts are bound to the currently selected provinces, cities and districts through v-model; Define three objects provinces, cities and districts in the data function, and the provinces array stores all provinces , the cities object stores all cities, and the districts object stores all districts. Use selectedProvince, selectedCity and selectedDistrict to record the currently selected province, city and district;- Monitor the changes of selectedProvince and selectedCity in the watch. When the province or When the city changes, the options and selected values of subsequent selection boxes are updated.
- The three-level linkage selector has been completed! You can reference the component in the Vue component template, as shown below:
<template> <div> <RegionSelect v-model="selectedRegion"/> </div> </template> <script> import RegionSelect from './RegionSelect.vue' export default { components: { RegionSelect }, data() { return { selectedRegion: ['广东', '深圳', '南山区'] } } } </script>
Summary
This article introduces how to use Vue components to implement multi-level linkage selectors, including defining child components and parent components , and the process of combining all subcomponents. Through this example, we can understand the basic ideas of Vue component development and the communication mechanism between components. Of course, more details need to be considered in actual development, such as asynchronous data acquisition, modifying the style of the subcomponent itself, etc. These contents are not covered in this article.
The above is the detailed content of Vue component development: multi-level linkage selector implementation. For more information, please follow other related articles on the PHP Chinese website!

Vue.js is a progressive JavaScript framework released by You Yuxi in 2014 to build a user interface. Its core advantages include: 1. Responsive data binding, automatic update view of data changes; 2. Component development, the UI can be split into independent and reusable components.

Netflix uses React as its front-end framework. 1) React's componentized development model and strong ecosystem are the main reasons why Netflix chose it. 2) Through componentization, Netflix splits complex interfaces into manageable chunks such as video players, recommendation lists and user comments. 3) React's virtual DOM and component life cycle optimizes rendering efficiency and user interaction management.

Netflix's choice in front-end technology mainly focuses on three aspects: performance optimization, scalability and user experience. 1. Performance optimization: Netflix chose React as the main framework and developed tools such as SpeedCurve and Boomerang to monitor and optimize the user experience. 2. Scalability: They adopt a micro front-end architecture, splitting applications into independent modules, improving development efficiency and system scalability. 3. User experience: Netflix uses the Material-UI component library to continuously optimize the interface through A/B testing and user feedback to ensure consistency and aesthetics.

Netflixusesacustomframeworkcalled"Gibbon"builtonReact,notReactorVuedirectly.1)TeamExperience:Choosebasedonfamiliarity.2)ProjectComplexity:Vueforsimplerprojects,Reactforcomplexones.3)CustomizationNeeds:Reactoffersmoreflexibility.4)Ecosystema

Netflix mainly considers performance, scalability, development efficiency, ecosystem, technical debt and maintenance costs in framework selection. 1. Performance and scalability: Java and SpringBoot are selected to efficiently process massive data and high concurrent requests. 2. Development efficiency and ecosystem: Use React to improve front-end development efficiency and utilize its rich ecosystem. 3. Technical debt and maintenance costs: Choose Node.js to build microservices to reduce maintenance costs and technical debt.

Netflix mainly uses React as the front-end framework, supplemented by Vue for specific functions. 1) React's componentization and virtual DOM improve the performance and development efficiency of Netflix applications. 2) Vue is used in Netflix's internal tools and small projects, and its flexibility and ease of use are key.

Vue.js is a progressive JavaScript framework suitable for building complex user interfaces. 1) Its core concepts include responsive data, componentization and virtual DOM. 2) In practical applications, it can be demonstrated by building Todo applications and integrating VueRouter. 3) When debugging, it is recommended to use VueDevtools and console.log. 4) Performance optimization can be achieved through v-if/v-show, list rendering optimization, asynchronous loading of components, etc.

Vue.js is suitable for small to medium-sized projects, while React is more suitable for large and complex applications. 1. Vue.js' responsive system automatically updates the DOM through dependency tracking, making it easy to manage data changes. 2.React adopts a one-way data flow, and data flows from the parent component to the child component, providing a clear data flow and an easy-to-debug structure.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
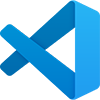
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
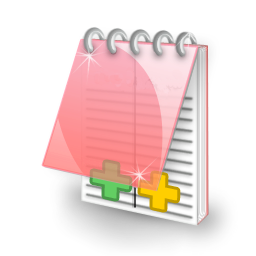
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function