Golang development advice: How to do effective error handling
Golang, as a modern programming language, has received widespread attention and use for its efficiency, simplicity, security and other characteristics. Error handling is an unavoidable part of any program development, and Golang provides a powerful error handling mechanism. This article will introduce how to implement effective error handling in Golang to help developers write more robust code.
1. Use of error types
In Golang, errors are represented by the error type. Therefore, when we write functions, we should explicitly handle errors as return values. For example, a simple function might return two values, one as a normal result and one as an error. As shown below:
func divide(x, y int) (int, error) { if y == 0 { return 0, errors.New("division by zero") } return x / y, nil }
When calling this function, we can use multiple assignments to obtain results and errors at the same time:
result, err := divide(10, 0)
2. Error checking and processing
In Golang, errors Processing usually adopts a "check and process" approach. That is, after executing a function that may return an error, we need to check the returned error and decide how to handle it. A common way to handle it is to use an if statement to determine whether the error is empty and handle it accordingly.
result, err := divide(10, 0) if err != nil { // 处理错误 fmt.Println("出现错误:", err) } else { // 处理正常结果 fmt.Println("计算结果:", result) }
Common processing methods include printing error information, recording logs, returning specific error codes, etc. When handling errors, you need to handle them accordingly based on specific business needs.
3. Propagation of Errors
In actual development, a function may call other functions to complete a series of operations. If an error occurs in a function, we need to pass the error to the upper-level calling function so that the upper-level function can handle the error. In Golang, we can pass errors as return values.
func foo() error { _, err := divide(10, 0) if err != nil { return err } // 其他操作 return nil }
In the above example, if the divide function returns an error, the foo function will also return the error to its upper-level calling function. In this way, we can achieve error propagation and handling.
4. Encapsulation of error handling
In order to improve the readability and maintainability of the code, we usually encapsulate errors. In Golang, we can use the functions provided by the errors package for encapsulation. For example, we can use the fmt.Errorf function to construct an error with detailed error information.
func divide(x, y int) (int, error) { if y == 0 { return 0, fmt.Errorf("division by zero: %d / %d", x, y) } return x / y, nil }
In this way, when handling errors, we can obtain detailed error information to better locate and solve problems.
5. Best practices for error handling
When writing Golang programs, we should follow some best practices for error handling to ensure the quality and stability of the code.
First of all, error information must be returned accurately to ensure that the error information is readable and can help developers quickly locate and solve problems.
Secondly, errors should be handled in the underlying function and ensure that the error can be correctly captured and handled by the upper-layer function to achieve error propagation.
In addition, for some unrecoverable errors, we should use the panic function to terminate the execution of the program, and use the defer function to perform necessary resource cleanup.
Summary:
Golang provides a powerful error handling mechanism to effectively help developers write robust code. During the development process, we should make full use of these mechanisms to handle and deliver errors accurately, while following some error handling best practices. Only in this way can we write more reliable and maintainable Golang programs.
The above is the detailed content of Golang development advice: How to do effective error handling. For more information, please follow other related articles on the PHP Chinese website!
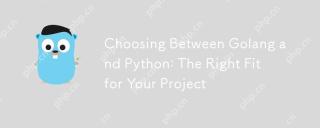
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
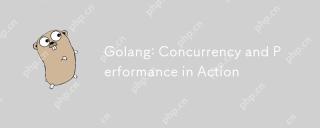
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
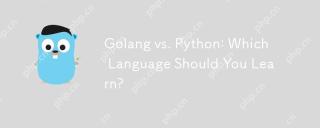
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
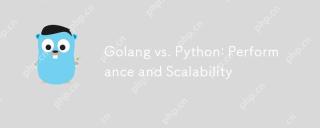
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
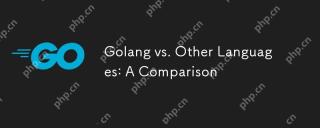
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
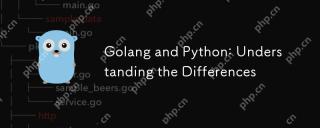
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
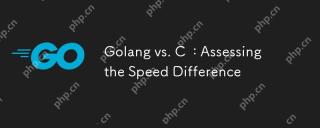
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
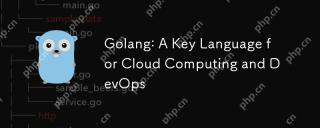
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
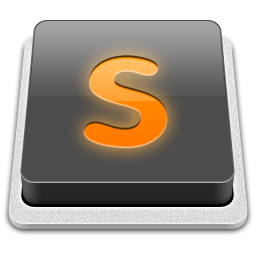
SublimeText3 Mac version
God-level code editing software (SublimeText3)
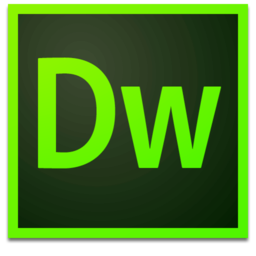
Dreamweaver Mac version
Visual web development tools
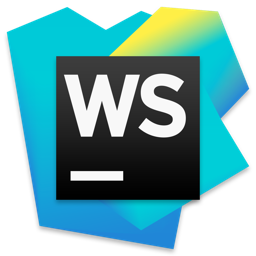
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment