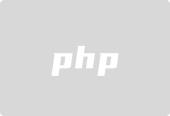
C Development Suggestions: How to Handle Elegant Errors in C Code
Introduction:
In C development, error handling is an important technology. Involves how to identify errors, handle abnormal situations, and ensure the normal operation of the program. A good error handling mechanism can improve the maintainability and reliability of the code, while poor error handling may lead to program crashes, resource leaks and other problems. This article will introduce some elegant error handling techniques to help developers better master C error handling.
1. Reasonable use of exception handling mechanism
Exception handling is a very powerful error handling mechanism in C. It can pass error information from the error generation point to the error handling point, so that the code can enables more graceful error recovery. When using exception handling, you should pay attention to the following points:
- Select the appropriate exception type: When designing a custom exception type, choose the appropriate exception type according to the specific error situation, and do not abuse it. Exception type. At the same time, you should try to use the existing exception types in the standard library to avoid reinventing the wheel.
- Be precise when catching exceptions: only catch exceptions that you can handle. Exceptions that cannot be handled should be re-thrown and let the upper layer code handle them. Catching exceptions that are too broad can lead to confusion and poor error handling.
- When an exception is thrown, a clear error message should be provided: When an exception is thrown, a clear error message should be provided to facilitate the upper-level code to understand and process it. When customizing exception types, you can consider overloading the constructor of the exception type to provide error information with different granularities.
2. Use RAII (resource acquisition and initialization) mechanism
RAII is a resource management technology that obtains resources in the constructor of the object and releases the resources in the destructor. Ensure the correct acquisition and release of resources. In error handling, RAII can be used to effectively handle the release of resources and ensure that resources will not be leaked when errors occur.
- Use smart pointers to manage resources: Smart pointers can automatically manage the allocation and release of resources. For resources that do not require manual management (such as heap memory), you can use smart pointers such as unique_ptr and shared_ptr for management. This ensures the correct release of resources.
- Use constructors and destructors to process resources: Obtain resources in the constructor of a class and release resources in the destructor to ensure the correct release of resources and avoid resource leaks. This method can also be used to manage custom resources, such as file handles, database connections, etc.
3. Proper use of return values and error codes
In addition to using exception handling and RAII mechanisms, return values and error codes are also a commonly used error handling method. In some cases, using return values and error codes allows for more flexible error handling. When using return values and error codes, you should pay attention to the following points:
- When returning error codes, you must distinguish error types: When using return values to represent error codes, the value range of the error code should be designed. , to be able to distinguish between different error types. At the same time, clear documentation should be provided for error codes to facilitate callers to understand the meaning of the error codes.
- Reasonable use of return values and error codes: In the return value of a function, you can use a special value (such as nullptr, -1) to indicate an error, and use error codes or error messages to provide more detailed errors. information. When using return values and error codes, pay attention to the consistency and readability of error handling.
Conclusion:
Good error handling is an important and tedious task in C development, but it can improve the maintainability and reliability of the code. By rationally utilizing the exception handling mechanism, using the RAII mechanism, and appropriately using return values and error codes, we can perform elegant error handling in C code. I hope this article helps you to better handle error conditions when writing C code.
The above is the detailed content of C++ development advice: How to handle elegant errors in C++ code. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn