


In the development process of Python, we often use object-oriented programming ideas and techniques to achieve modularization and reuse of code. But at the same time, functional programming is also another useful programming paradigm. It can help us better manage the state and side effects of the code, and improve the readability and maintainability of the code. This article will introduce the ideas and techniques of functional programming in detail, and put forward some suggestions for learning and application.
1. What is functional programming?
Functional programming is a programming paradigm. Its core idea is to regard the calculation process as an interaction between functions, rather than as an object-oriented or procedural programming. Changes in series status. In functional programming, functions are first-class citizens and can be passed, nested, and manipulated like other data types. This also makes functional programming more flexible in handling complex computing tasks.
Functional programming emphasizes stateless and side-effect-free functions, that is, the output of the function only depends on the input and will not be affected by or affect the external state. Such functions are reusable and testable, making it easier for developers to encapsulate, combine and test them. In addition, functional programming also draws on many concepts and methods in mathematics, such as higher-order functions, pure functions, recursion, etc., to simplify and optimize code implementation.
2. Skills and applications of functional programming
1. Use lambda and higher-order functions
lambda is an anonymous function in Python, which can be used to quickly define some simple functions Functions, such as:
add = lambda x, y: x + y print(add(1, 2)) # 输出: 3
Higher-order functions refer to functions that use functions as parameters or return values. It can help us abstract the code for better combination and encapsulation, such as:
def apply(func, x): return func(x) print(apply(lambda x: x * x, 3)) # 输出: 9
2. Use generators and iterators
Generators and iterators are commonly used iteration tools in Python, which can help us deal with large data and lazy calculations, thereby improving the efficiency and scalability of the code. sex. For example:
def squares(n): for i in range(n): yield i * i for x in squares(5): print(x) # 输出: 0 1 4 9 16
3. Use pure functions and avoid side effects
Pure functions refer to functions whose input and output are uniquely determined and will not affect the external state. This function has the ability to be reproduced. Usability and testability also avoid common mistakes and debugging headaches. Avoiding side effects means avoiding operations on stateful resources such as global variables, files, and databases in functions, thereby making the code more concise and easier to maintain.
# 非纯函数 x = 0 def increment(): global x x += 1 return x print(increment()) # 输出: 1 print(increment()) # 输出: 2 # 纯函数 def increment(x): return x + 1 print(increment(1)) # 输出: 2 print(increment(2)) # 输出: 3
4. Use functional toolboxes and frameworks
There are many functional programming toolboxes and frameworks in Python, such as toolz, funcy, PyMonad, etc. They provide many useful functions, data types and data processing tools that can help us better handle complex data and business logic. For example:
from toolz import pipe data = [1, 2, 3, 4, 5] result = pipe(data, (map(lambda x: x * x)), (filter(lambda x: x > 10)), (reduce(lambda x, y: x + y))) print(result) # 输出: 29
3. Suggestions for learning and applying functional programming
1. Understand the core ideas and concepts of functional programming, such as higher-order functions, pure functions, lazy calculation, etc.
2. Learn to use lambda and functional toolboxes, such as toolz, funcy, etc., to better process data and business logic.
3. Understand the specific implementation and application scenarios of functional programming in Python, such as functional API, streaming computing, event-driven, etc.
4. In actual development, pay attention to avoid side effects and the use of global variables, and try to treat the calculation process as an interaction between functions.
5. Combine object-oriented and functional programming, and flexibly use different programming paradigms to better meet the needs and complexity of the code.
The above is the detailed content of Python development advice: Learn and apply functional programming ideas and techniques. For more information, please follow other related articles on the PHP Chinese website!
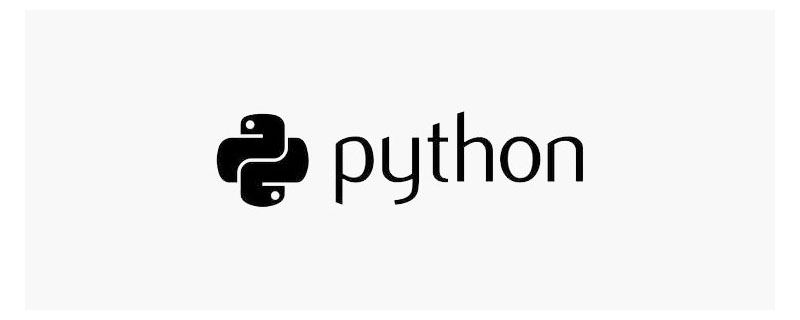
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
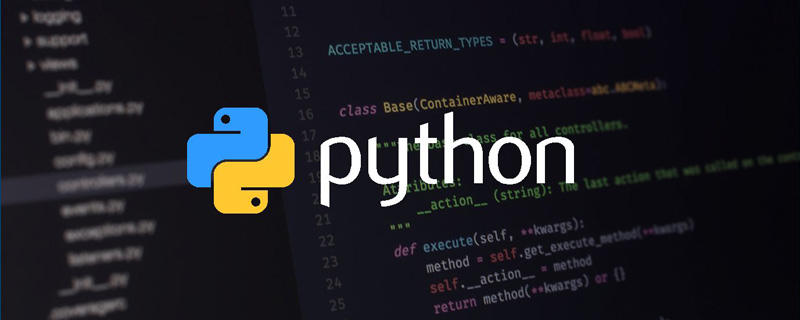
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
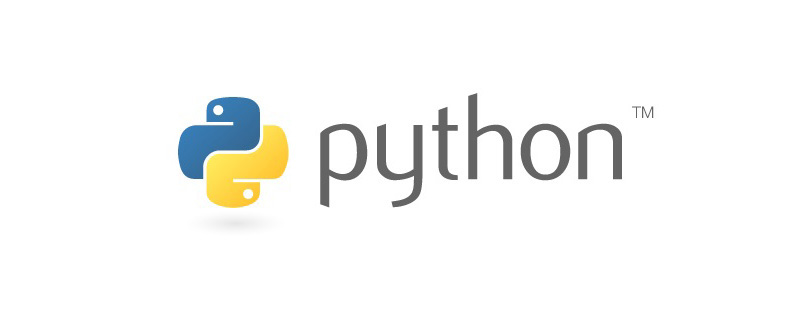
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
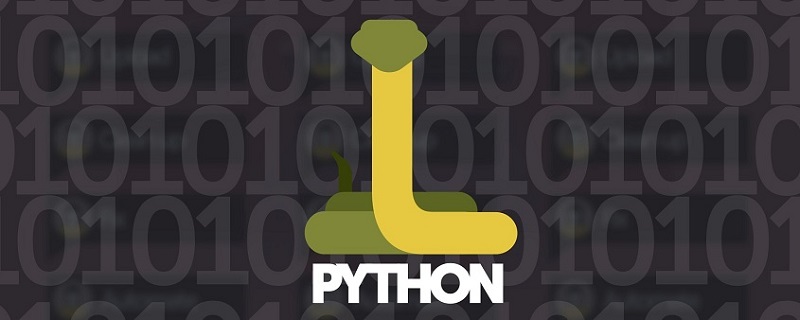
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
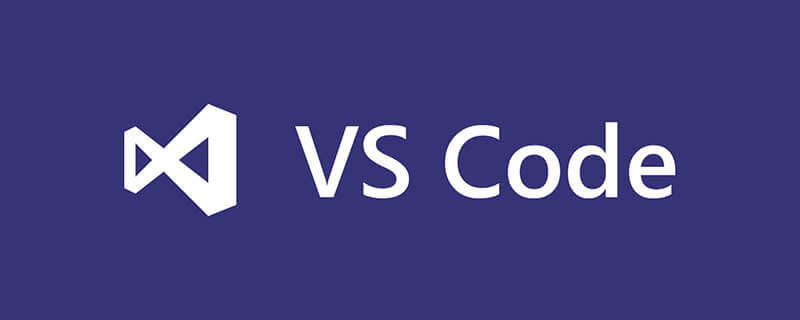
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
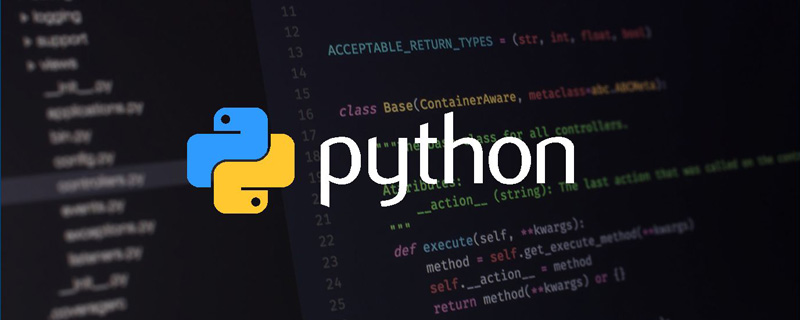
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。
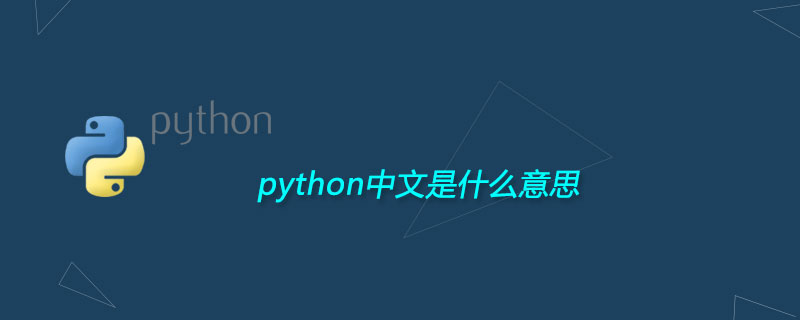
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
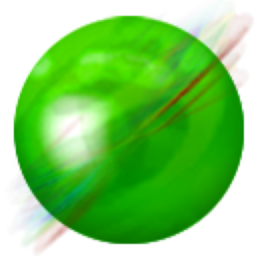
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
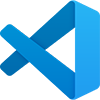
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
