


Title: Using JavaScript and Tencent Maps to implement map interactive navigation function
The navigation function plays an important role in modern society, it can help people more Get to know a place well, find your destination and plan your route. This article will introduce how to use JavaScript and Tencent Map API to implement map interactive navigation function, and provide specific code examples.
1. Preparation work
Before we start writing code, we need to do some preparation work:
- Obtain the developer key of Tencent Map, which can be opened on Tencent Map Platform application.
-
Create an HTML file and introduce Tencent Map’s JavaScript library:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>地图导览</title> <script src="https://map.qq.com/api/js?v=2.exp&key=YOUR_KEY"></script> </head> <body> <div id="map" style="width: 100%; height: 500px;"></div> </body> </html>
Please replace YOUR_KEY above with your Tencent Map developer key.
2. Display the map
Next, we will use JavaScript to display a map on the web page. Add the following JS code within the
<script> var map = new qq.maps.Map(document.getElementById("map"), { center: new qq.maps.LatLng(39.908722, 116.397499), // 地图中心点坐标 zoom: 13 // 地图缩放级别 }); </script>
This code creates a map instance and sets the map's center point coordinates and zoom level.
3. Add marker points and information window
Now, we can add marker points on the map and set an information window for each marker point. This window will pop up when the user clicks on the marker point.
First, we need to define the location of a marker point and the content of an information window. In the JS code, add the following code:
<script> var marker = new qq.maps.Marker({ position: new qq.maps.LatLng(39.908722, 116.397499), map: map }); var infoWindow = new qq.maps.InfoWindow({ content: "这是一个标记点的信息窗口!" }); qq.maps.event.addListener(marker, 'click', function() { infoWindow.open(); }); </script>
This code creates a marker point and an information window and adds them to the map. When the user clicks on the marker point, an information window will pop up to display the defined content.
4. Add navigation function
Now, we can provide users with navigation function, allowing them to plan a route from the current location to the target location by clicking on the marked point on the map.
First, we need to get the user's current location. In the JS code, add the following code:
<script> if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(function(position) { var currentPosition = new qq.maps.LatLng(position.coords.latitude, position.coords.longitude); var currentMarker = new qq.maps.Marker({ position: currentPosition, map: map }); var drivingService = new qq.maps.DrivingService({ map: map }); qq.maps.event.addListener(marker, 'click', function() { drivingService.search(currentPosition, marker.getPosition()); }); }); } </script>
This code uses HTML5’s Geolocation API to obtain the user’s current location and add a marker on the map that represents the current location. At the same time, we also created a DrivingService object for route planning, and called the drivingService.search() method to plan the route when the marker point is clicked.
The above is a specific code example that uses JavaScript and Tencent Map API to implement the interactive map navigation function. Through these codes, we can display the map on the web page, add markers and information windows, and provide navigation functions for users. I hope this article can help you understand and use Tencent Map API!
The above is the detailed content of Using JavaScript and Tencent Maps to implement map interactive navigation function. For more information, please follow other related articles on the PHP Chinese website!
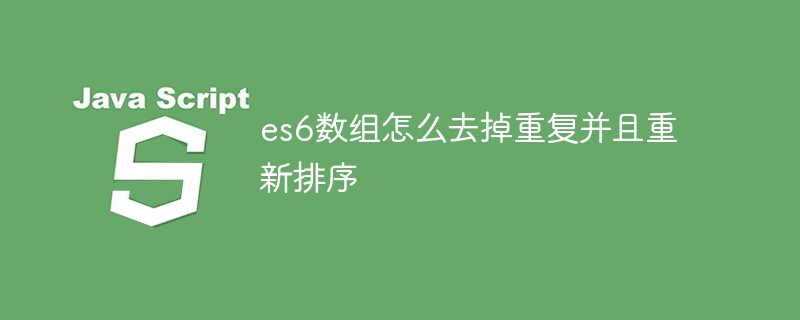
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
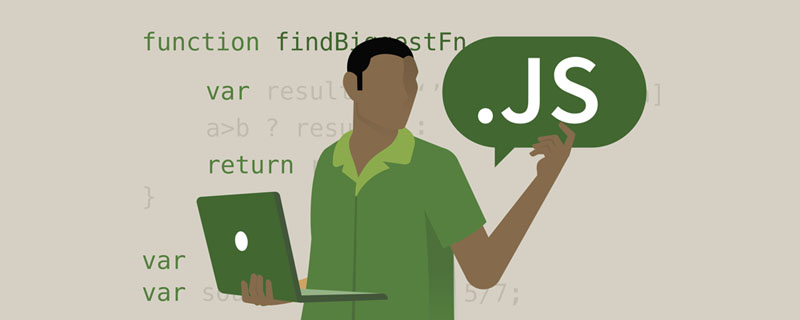
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
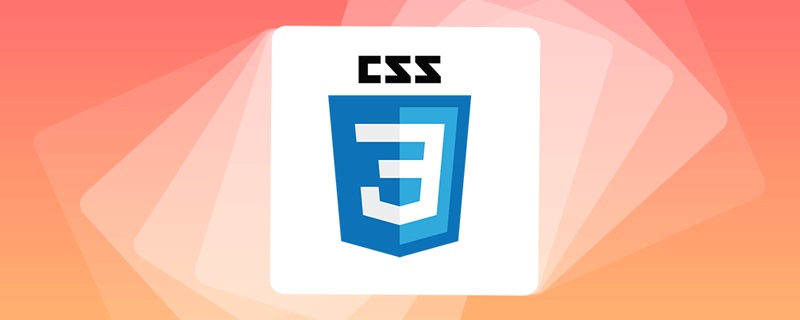
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
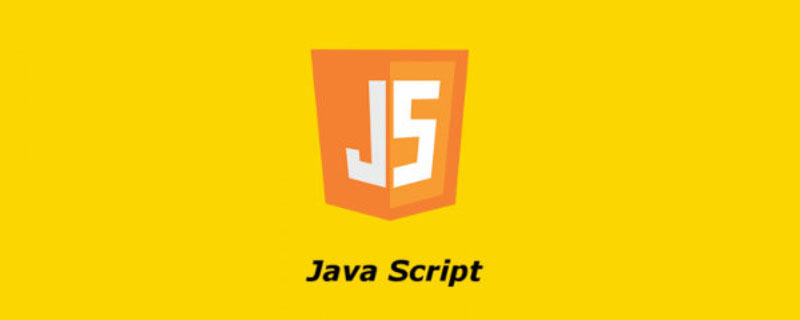
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
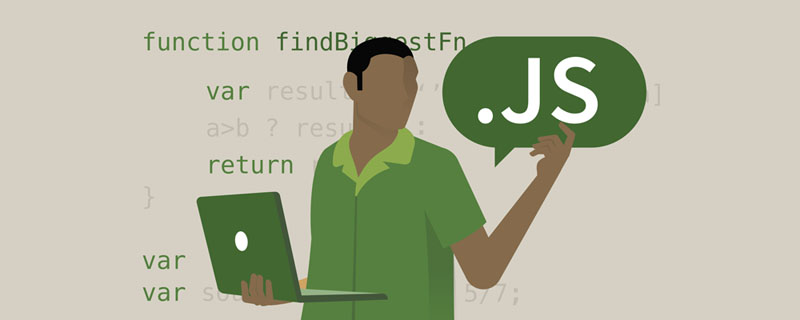
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
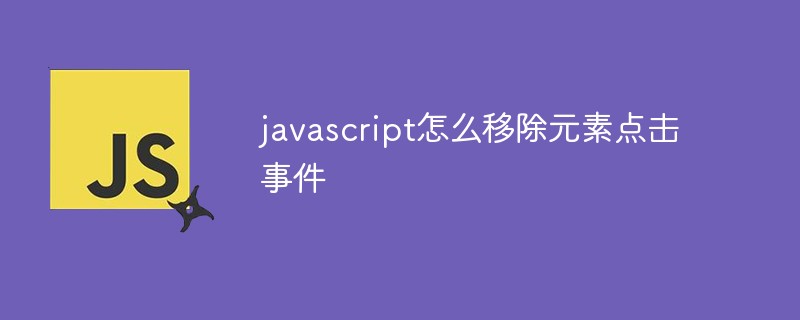
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
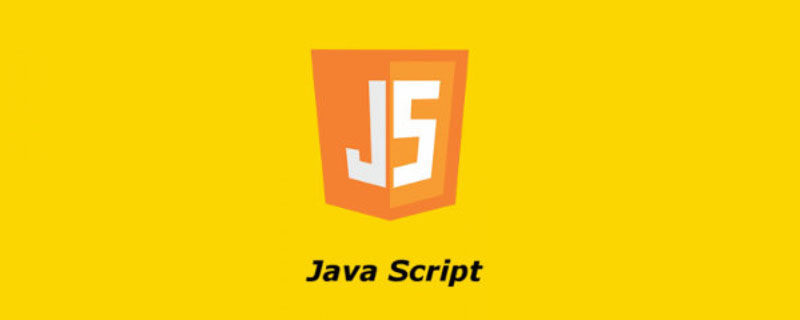
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
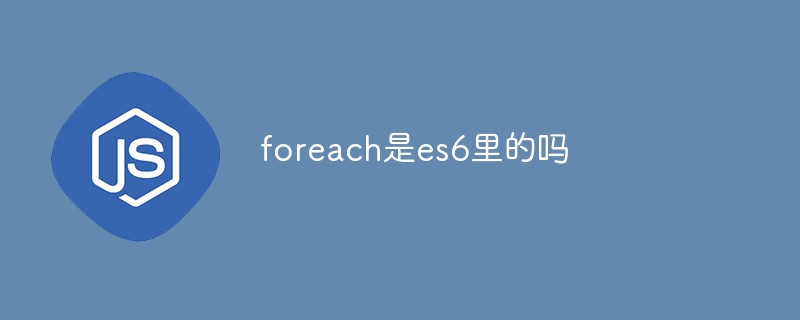
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
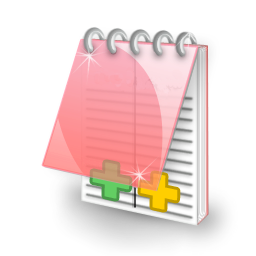
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
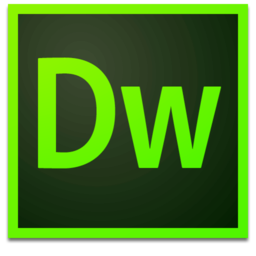
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
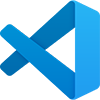
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
