


Using JavaScript and Tencent Maps to implement map path planning function
Use JavaScript and Tencent Maps to implement map route planning function
In recent years, with the rapid development of the Internet, map navigation functions have become an essential tool for people to travel. In our daily lives, we often encounter situations where we need to plan the best route, such as travel, driving, delivery, etc. This article will introduce how to use JavaScript and Tencent Map API to implement the map route planning function, and provide relevant code examples.
First of all, we need to introduce the API of Tencent Maps and implement it through the following code:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>地图路径规划</title> <style type="text/css"> #map-container { width: 100%; height: 400px; } </style> <script src="http://map.qq.com/api/js?v=2.exp&key=YOUR_KEY"></script> </head> <body> <div id="map-container"></div> <script> // 在这里编写 JavaScript 代码 </script> </body> </html>
Among them, YOUR_KEY
needs to be replaced with your own Tencent Maps API key.
Next, we need to implement the map display and path planning functions in JavaScript code. The specific code example is as follows:
// 初始化地图 var map = new qq.maps.Map(document.getElementById('map-container'), { center: new qq.maps.LatLng(39.916527, 116.397128), // 设置地图中心点坐标 zoom: 13 // 设置地图缩放级别 }); // 调用腾讯地图的路径规划服务 var service = new qq.maps.DirectionService({ complete: function(result) { var path = result.detail.path[0]; var polyline = new qq.maps.Polyline({ path: path, strokeColor: '#3388ff', strokeWeight: 5, map: map }); map.fitBounds(polyline.getBounds()); // 调整地图视野以显示整条路径 } }); // 设置起点和终点坐标 var start = new qq.maps.LatLng(39.915, 116.400); var end = new qq.maps.LatLng(39.948, 116.415); // 发起路径规划请求 service.search(start, end); // 在地图上标注起点和终点 new qq.maps.Marker({ position: start, map: map }); new qq.maps.Marker({ position: end, map: map });
In the above code, we first create a map instance and set the display position and zoom level of the map. Then, initiate a path planning request by calling the search method of the qq.maps.DirectionService object. Finally, use the qq.maps.Polyline object to draw the path and mark the start and end points on the map.
Of course, in addition to the starting point and end point, you can also set more way points. Just add the coordinates sequentially to the array between the start and end points and modify your code accordingly.
It should be noted that when using Tencent Map API for route planning function, you need to use a valid API key and follow the usage specifications of Tencent Map API. Detailed usage methods and API documentation can be found on the Tencent Map Open Platform.
To sum up, using JavaScript and Tencent Maps, we can easily implement the map path planning function. By writing relevant code and introducing Tencent Map API into the page, we can obtain the best path and display it on the map. This is one of the very practical and important functions for map navigation applications.
Reference link:
Tencent Map Open Platform: http://lbs.qq.com/
The above is the detailed content of Using JavaScript and Tencent Maps to implement map path planning function. For more information, please follow other related articles on the PHP Chinese website!
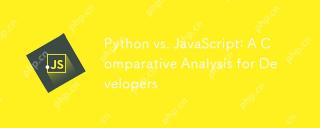
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
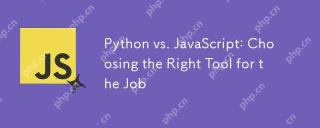
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
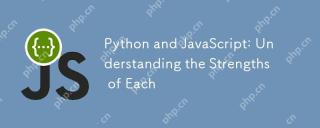
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
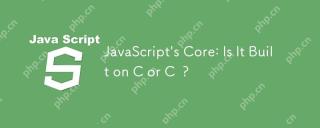
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
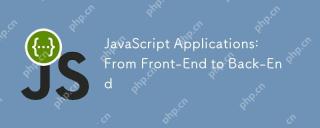
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
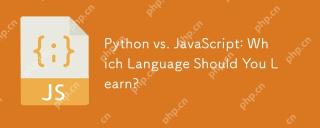
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
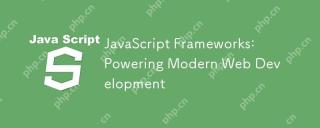
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
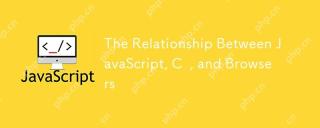
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
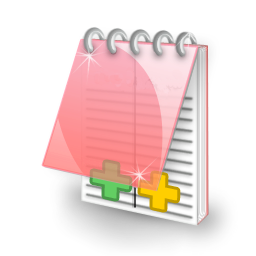
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
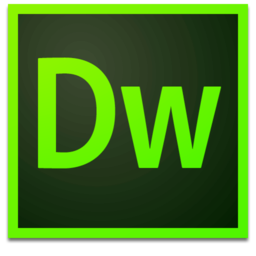
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
