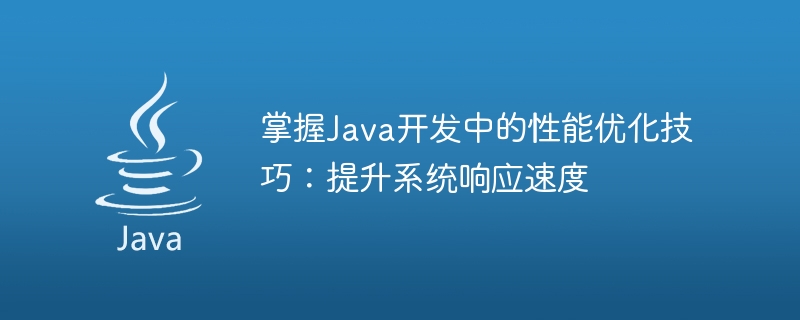
Master the performance optimization skills in Java development: Improve system response speed
With the popularization of the Internet and the advent of the information age, the performance of software systems has become a priority for developers One of the important issues that concern us. For Java development, performance optimization is a key task, which can greatly improve the system's response speed and user experience. This article will introduce some performance optimization techniques in Java development and discuss how to improve the response speed of the system.
1. Optimize Java code
The quality of Java code directly affects the performance of the system. When writing Java code, we should pay attention to the following aspects:
- Avoid over-reliance on string concatenation operations. String concatenation operations are time-consuming, especially when string concatenation operations are performed frequently in loops. You can use the StringBuilder class to replace string concatenation operations to improve efficiency.
- Avoid frequent creation and destruction of objects. The creation and destruction process of objects consumes a lot of memory and system resources. Creating a large number of temporary objects in a loop will increase the burden of garbage collection and reduce system performance. Objects can be reused, improving system performance.
- Use loops appropriately. For large loop operations, consider using parallel streams or concurrent programming to improve performance. In addition, you should avoid doing a large number of IO operations and database access in the loop. You can place these operations outside the loop to improve system performance.
2. Reasonable use of Java collections
In Java development, collections are one of our commonly used data structures. Using appropriate collection types and avoiding unnecessary traversals can improve system performance.
- For scenarios where elements need to be frequently added or deleted, LinkedList can be used instead of ArrayList. LinkedList has better performance in addition and deletion operations.
- In scenarios where search operations are required, HashSet or HashMap can be used instead of ArrayList or LinkedList. The search operation time complexity of HashSet and HashMap is O(1), which is highly efficient.
- When traversing collection elements, it is more efficient to use iterators than for loops. Because the iterator does not check the modification of the collection during the iteration process, unnecessary overhead is reduced.
3. Reasonable use of threads and thread pools
Java provides multi-threading support, which can make full use of the performance of multi-core processors. Proper use of threads and thread pools can improve the concurrency performance of the system.
- Use the thread pool to manage the creation and destruction of threads. The thread creation and destruction process consumes system resources. Using a thread pool can avoid frequent creation and destruction of threads and improve system performance.
- Reasonably allocate the number of threads. Too many threads will consume too many system resources, and too few threads will cause the system's response speed to drop. The best performance can be achieved by adjusting the number of threads in the thread pool.
- Use thread-safe data structures. In a multi-threaded environment, data consistency and security need to be ensured. You can use thread-safe data structures, such as ConcurrentHashMap, to ensure data security.
4. Database performance optimization
The database is an indispensable part of many systems. Optimizing the database can improve the response speed of the system.
- Use indexes to optimize query performance. By creating appropriate indexes, the speed of queries can be greatly improved. But be careful not to create too many indexes, otherwise it will reduce the update speed of the database.
- Use the database connection pool rationally. The creation and destruction process of database connections consumes resources. Using a connection pool can avoid frequent connection creation and destruction and improve system performance.
- Avoid excessive use of database connections. When querying data, the number of database connections should be minimized. You can query multiple pieces of data at one time to reduce the number of database connections and improve system performance.
Summary:
The above are some performance optimization techniques in Java development. By optimizing Java code, rational use of Java collections, rational use of threads and thread pools, and database performance optimization, you can Greatly improve the system's response speed. In actual development, we should optimize performance according to the needs and characteristics of the system and specific circumstances to improve system performance and user experience.
The above is the detailed content of Master performance optimization skills in Java development: improve system response speed. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn