Python's min() function: Get the minimum value in the list, specific code examples are required
The Python programming language is a simple, easy-to-learn and powerful language. It provides many built-in functions to handle lists and other data structures. One of the very useful built-in functions is the min() function, which can be used to get the minimum value in a list.
In Python, we often need to find the smallest element in a list. This task can be accomplished by looping through the list and recording the minimum value, but this approach is tedious and error-prone. The min() function can accomplish this task in a more concise way.
The syntax of the min() function is very simple. It accepts an iterable object as a parameter and returns the minimum value in the object. Here is an example:
numbers = [5, 2, 9, 1, 7] min_number = min(numbers) print(min_number) # 输出结果为1
In the above example, we defined a list of numbers containing 5 integers. We then use the min() function to get the minimum value in the list and assign the result to the variable min_number. Finally, we print the minimum value using the print() function.
In addition to lists, the min() function can also be used for other types of iterable objects, such as tuples and sets. Here is an example of using tuples:
animals = ('cat', 'dog', 'elephant', 'lion') min_animal = min(animals) print(min_animal) # 输出结果为cat
In the above example, we define a tuple animals containing four strings. We then use the min() function to get the minimum value in the tuple and assign the result to the variable min_animal. Finally, we print the minimum value using the print() function.
In addition to the default comparison method, the min() function can also accept an optional key parameter to specify the method of comparing elements. For example, if we want to get the shortest string in a list of strings based on the length of letters, we can use the len() function as the key parameter. Here is an example:
words = ['apple', 'banana', 'orange', 'kiwi'] min_word = min(words, key=len) print(min_word) # 输出结果为kiwi
In the above example, we define a list of four strings words. We then use the min() function to get the shortest string in the list and assign the result to the variable min_word. Finally, we print the shortest string using the print() function.
To summarize, the min() function is a very useful function in Python and can be used to obtain the minimum value in lists and other iterable objects. Its use is very simple, just pass the object to be compared as a parameter to the function. In addition, you can specify the comparison method through the optional key parameter. In actual programming, the min() function is often used to find the minimum value, as well as in some sorting and search algorithms. I hope the above code examples can help you better understand and use the min() function.
The above is the detailed content of Python's min() function: Get the minimum value in a list. For more information, please follow other related articles on the PHP Chinese website!
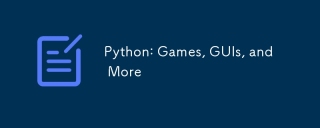
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
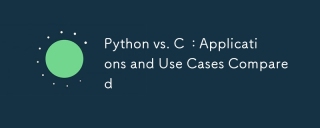
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
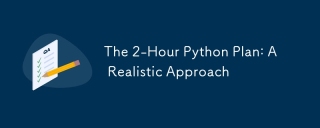
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
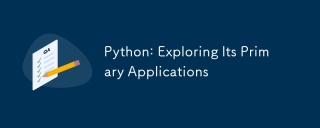
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
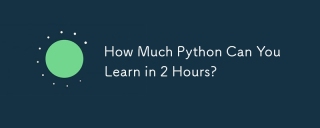
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
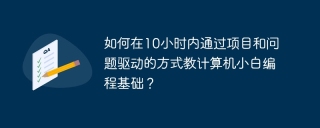
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
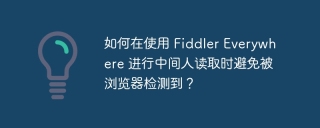
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
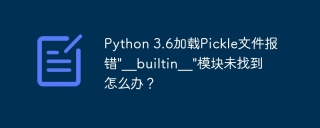
Error loading Pickle file in Python 3.6 environment: ModuleNotFoundError:Nomodulenamed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
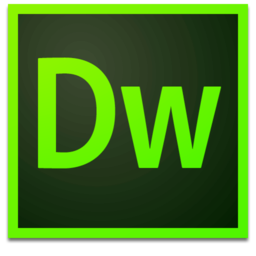
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.