


How to send POST request and get response using http.Post function in golang
How to use the http.Post function in golang to send a POST request and get the response
When using golang for network programming, the http package is an important one we often use module. Among them, the http.Post function is a very practical function that can easily send POST requests and obtain response results. The following will introduce the specific steps and code examples on how to use the http.Post function to send a POST request and obtain a response.
Step 1: Import the http package
In the code, you first need to import the http package in order to use the related functions and types in the package. In golang, the syntax for importing packages is as follows:
import "net/http"
Step 2: Construct request parameters
To send a POST request, we need to prepare the request URL and request body. The request URL is a string, and the request body is a byte stream containing request parameters. You can use the url.Values type to construct request parameters, as shown below:
values := url.Values{} values.Set("key1", "value1") values.Set("key2", "value2")
Step 3: Send a POST request
Sending a POST request using the http.Post function is very simple, you only need to provide the requested Just the URL and request body. The code is as follows:
url := "http://example.com/api" // 要发送的POST请求的URL body := strings.NewReader(values.Encode()) // 将请求参数编码成字节流 response, err := http.Post(url, "application/x-www-form-urlencoded", body) // 发送POST请求 if err != nil { fmt.Println("发送POST请求失败:", err) return } defer response.Body.Close()
Step 4: Process the response result
After sending the POST request through the http.Post function, you can obtain the response result through the response object. The response result is a byte stream containing the data returned by the server. We can use the ioutil.ReadAll function to convert the byte stream into a string to facilitate processing of the response results. The code is as follows:
result, err := ioutil.ReadAll(response.Body) // 读取响应结果 if err != nil { fmt.Println("读取响应结果失败:", err) return } fmt.Println("响应结果:", string(result)) // 输出响应结果
Complete code example:
package main import ( "fmt" "io/ioutil" "net/http" "net/url" "strings" ) func main() { values := url.Values{} values.Set("key1", "value1") values.Set("key2", "value2") url := "http://example.com/api" body := strings.NewReader(values.Encode()) response, err := http.Post(url, "application/x-www-form-urlencoded", body) if err != nil { fmt.Println("发送POST请求失败:", err) return } defer response.Body.Close() result, err := ioutil.ReadAll(response.Body) if err != nil { fmt.Println("读取响应结果失败:", err) return } fmt.Println("响应结果:", string(result)) }
Through the above steps, we can use the http.Post function in golang to send a POST request and obtain the response result returned by the server. It should be noted that in the sample code, the request parameters are encoded and sent in query string format. If you need to send the request body in JSON format, you can use the json.Marshal function to convert the request parameters into a byte stream in JSON format and set the Content-Type. is "application/json".
I hope this article can be helpful to you when using the http.Post function in golang to send a POST request and get the response.
The above is the detailed content of How to send POST request and get response using http.Post function in golang. For more information, please follow other related articles on the PHP Chinese website!
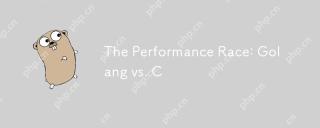
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
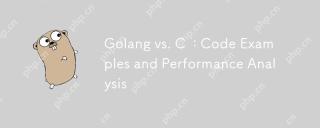
Golang is suitable for rapid development and concurrent programming, while C is more suitable for projects that require extreme performance and underlying control. 1) Golang's concurrency model simplifies concurrency programming through goroutine and channel. 2) C's template programming provides generic code and performance optimization. 3) Golang's garbage collection is convenient but may affect performance. C's memory management is complex but the control is fine.
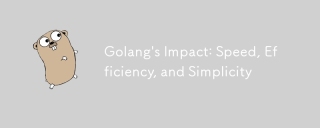
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
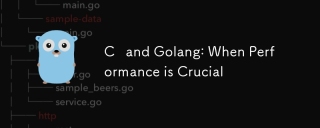
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
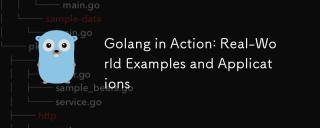
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
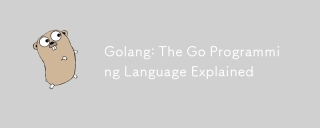
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
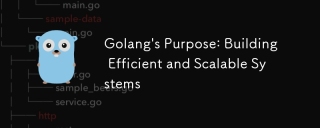
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
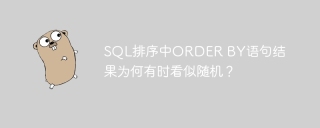
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
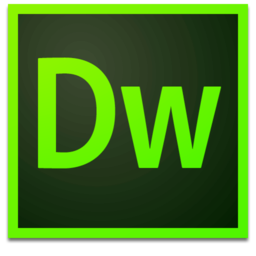
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool