PHP8 can use mysqli and PDO to connect to the database. Detailed introduction: 1. Use mysqli to connect to the database by passing in the database server name, user name, password and database name to connect. Then, use the `connect_error` attribute to check whether the connection is successful and output an error message if the connection fails. Finally, close the connection by calling the `close()` method; 2. Use PDO to connect to the database, and connect by passing in the database server name, password and database name, etc.
The operating system of this tutorial: windows10 system, php8.1.3 version, DELL G3 computer.
PHP8 can use mysqli and PDO to connect to the database.
1. Use mysqli to connect to the database:
First, you need to ensure that the mysqli extension has been installed. Then, you can connect to the database by following the steps below:
$servername = "localhost"; // 数据库服务器名称或IP地址 $username = "username"; // 数据库用户名 $password = "password"; // 数据库密码 $dbname = "database"; // 数据库名称 // 创建连接 $conn = new mysqli($servername, $username, $password, $dbname); // 检查连接是否成功 if ($conn->connect_error) { die("连接失败: " . $conn->connect_error); } else { echo "连接成功!"; } // 关闭连接 $conn->close(); ?>
This code first creates a mysqli object by passing in the database server name, user name, password and database name. Make a connection. Then, use the `connect_error` attribute to check whether the connection is successful and output an error message if the connection fails. Finally, the connection is closed by calling the `close()` method.
2. Use PDO to connect to the database:
First, you need to ensure that the PDO extension and corresponding database driver have been installed. Then, you can connect to the database by following the steps below:
$servername = "localhost"; // 数据库服务器名称或IP地址 $username = "username"; // 数据库用户名 $password = "password"; // 数据库密码 $dbname = "database"; // 数据库名称 // 创建连接 $dsn = "mysql:host=$servername;dbname=$dbname;charset=utf8mb4"; $options = [ PDO::ATTR_ERRMODE => PDO::ERRMODE_EXCEPTION, PDO::ATTR_DEFAULT_FETCH_MODE => PDO::FETCH_ASSOC, PDO::ATTR_EMULATE_PREPARES => false, ]; try { $conn = new PDO($dsn, $username, $password, $options); echo "连接成功!"; } catch (PDOException $e) { die("连接失败: " . $e->getMessage()); } // 关闭连接 $conn = null; ?>
This code first creates a PDO object by passing in the database server name, user name, password and database name. Make a connection. Then, use a `try-catch` block to catch exceptions that may occur during the connection process and output error information. Finally, close the connection by assigning null to the connection object.
The above is the method of using mysqli and PDO to connect to the database respectively. According to the specific situation, choose the connection method that suits you, and perform corresponding configuration and operation according to actual needs.
The above is the detailed content of How to connect to the database in php8. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
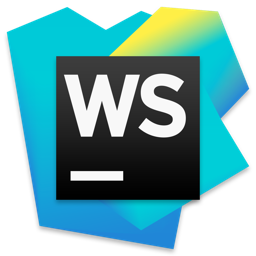
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor