


How to implement the thread scheduling and locking mechanism of Java's underlying technology
In Java development, thread scheduling and locking mechanisms are very important underlying technologies. Thread scheduling refers to how the operating system allocates time slices and execution sequences to different threads, while the lock mechanism is to ensure data synchronization and mutually exclusive access among multiple threads. This article will detail how to implement these two underlying technologies and provide specific code examples.
1. Thread Scheduling
Thread scheduling is the operating system's time slice allocation and execution sequence arrangement for multi-threads. In Java, we can implement thread scheduling by using some methods provided by the Thread class.
- Set priority
In Java, each thread has its own priority, and the priority of the thread can be set through the setPriority() method. The priority range is 1-10, where 1 is the lowest priority and 10 is the highest priority.
The sample code is as follows:
Thread thread1 = new Thread(); thread1.setPriority(Thread.MIN_PRIORITY); Thread thread2 = new Thread(); thread2.setPriority(Thread.NORM_PRIORITY); Thread thread3 = new Thread(); thread3.setPriority(Thread.MAX_PRIORITY);
- Thread sleep
Thread sleep refers to suspending the execution of the thread for a period of time, which can be achieved through the sleep() method of the Thread class . This method accepts a number of milliseconds as a parameter, indicating how long the thread should pause execution.
The sample code is as follows:
try { Thread.sleep(1000); // 线程暂停执行1秒 } catch (InterruptedException e) { e.printStackTrace(); }
- Thread waiting
Thread waiting means to let one thread wait for another thread to finish executing before continuing. This can be achieved using the join() method of the Thread class.
The sample code is as follows:
Thread thread1 = new Thread(); Thread thread2 = new Thread(); // 启动线程1 thread1.start(); // 在主线程中等待线程1执行完毕 try { thread1.join(); } catch (InterruptedException e) { e.printStackTrace(); } // 启动线程2 thread2.start();
2. Locking mechanism
In multi-threaded programming, the locking mechanism is used to protect resources shared by multiple threads to avoid concurrent access. brought about problems. Java provides the synchronized keyword and Lock interface to implement the lock mechanism.
- synchronized keyword
The synchronized keyword can be used to modify a method or a code block to protect access to shared resources. When a thread enters a synchronized method or code block, it acquires the lock on this object, and other threads must wait for the thread to release the lock before they can continue execution.
The sample code is as follows:
public synchronized void method() { // 同步代码块 synchronized (this) { // 访问共享资源 } }
- Lock interface
Lock interface is another way to implement the lock mechanism provided by Java. It is more flexible than the synchronized keyword . The Lock interface provides methods such as lock(), unlock(), tryLock(), etc., which can control thread synchronization access in a more fine-grained manner.
The sample code is as follows:
Lock lock = new ReentrantLock(); lock.lock(); // 获取锁 try { // 访问共享资源 } finally { lock.unlock(); // 释放锁 }
The above is a detailed introduction on how to implement the thread scheduling and locking mechanism of Java's underlying technology. Through the above code examples, we can better understand and Use these two underlying technologies. In multi-threaded programming, reasonable use of thread scheduling and lock mechanisms can improve the efficiency and concurrency of the program. Hope this article is helpful to you.
The above is the detailed content of How to implement thread scheduling and locking mechanism of Java underlying technology. For more information, please follow other related articles on the PHP Chinese website!
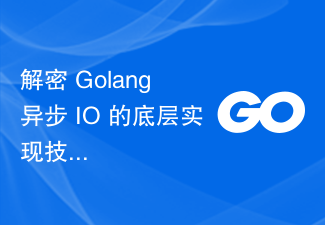
Golang作为一种强大而灵活的编程语言,其在异步IO方面有着独特的设计和实现。本文将深度解析Golang异步IO的底层实现技术,探讨其机制和原理,并提供具体的代码示例进行演示。1.异步IO概述在传统的同步IO模型中,一个IO操作会阻塞程序的执行,直到读写完成并返回结果。相比之下,异步IO模型允许程序在等待IO操作完成的同
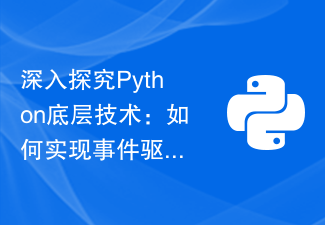
Python是一种高级编程语言,被广泛用于开发各种应用程序。在Python编程语言中,事件驱动编程被认为是一种非常高效的编程方式。它是一种编写事件处理程序的技术,其中程序代码按照事件的发生顺序执行。事件驱动编程的原理事件驱动编程是一种应用程序设计技术,该技术基于事件触发器。事件触发器由事件监视系统负责。当事件触发器被触发时,事件监视系统将调用应用程序的事件处
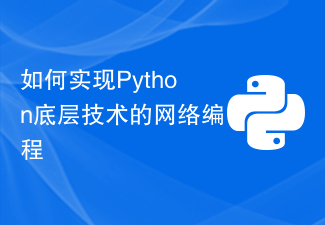
如何实现Python底层技术的网络编程网络编程是现代软件开发中的一个重要技术领域,通过网络编程,我们可以实现应用程序之间的通信,实现跨机器、跨平台的数据传输和交互。Python作为一种广泛使用的编程语言,提供了简洁而强大的底层技术来实现网络编程。本文将介绍如何使用Python的底层技术进行网络编程,并提供一些具体的代码示例。套接字(Socket):套接字是网
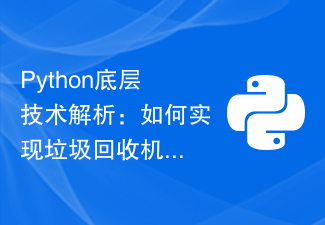
Python底层技术解析:如何实现垃圾回收机制,需要具体代码示例引言:Python作为一种高级编程语言在开发中极为方便和灵活,但是其底层实现却是相当复杂的。本文将重点探讨Python的垃圾回收机制,包括垃圾回收的原理、算法以及具体的实现代码示例。希望通过本文对Python垃圾回收机制的解析,读者能够更加深入地了解Python底层技术。一、垃圾回收原理首先,我
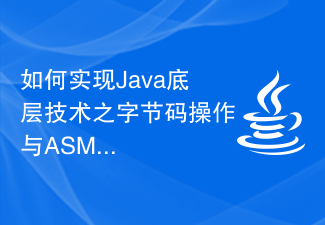
如何实现Java底层技术之字节码操作与ASM框架引言:Java作为一种高级编程语言,对于开发者来说,往往不需要关注底层的细节。然而,在某些特殊场景下,我们可能需要深入了解Java底层技术,例如字节码操作。本文将介绍如何通过ASM框架实现Java字节码操作,并提供具体的代码示例。一、什么是字节码操作?在Java的编译过程中,源代码将被编译为字节码,然后由JVM
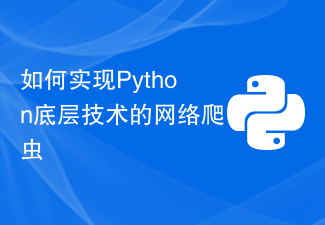
如何使用Python实现网络爬虫的底层技术网络爬虫是一种自动化的程序,用于在互联网上自动抓取和分析信息。Python作为一门功能强大且易于上手的编程语言,在网络爬虫开发中得到了广泛应用。本文将介绍如何使用Python的底层技术来实现一个简单的网络爬虫,并提供具体的代码示例。安装必要的库要实现网络爬虫,首先需要安装并导入一些Python库。在这里,我们将使用以
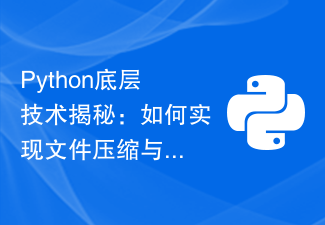
Python底层技术揭秘:如何实现文件压缩与解压缩文件压缩与解压缩是我们在日常开发中经常需要处理的任务之一。Python作为一种强大的编程语言,提供了丰富的库和模块来处理文件操作,其中包括文件压缩与解压缩的功能。本文将揭秘Python底层技术,讲解如何使用Python来实现文件的压缩与解压缩,并提供具体的代码示例。在Python中,我们可以使用标准库中的zi
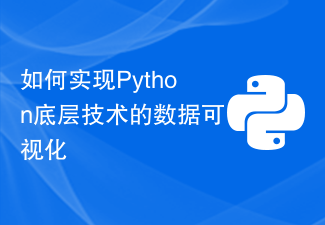
在当今人工智能和大数据时代,数据可视化成为了数据分析应用中的一个非常重要的环节。数据可视化能够帮助我们更加直观地理解数据,发现数据中的规律和异常,同时也能够帮助我们更加清晰地向他人传递自己的数据分析。Python是当前被广泛使用的编程语言之一,其在数据分析和数据挖掘领域表现非常出色。Python提供了丰富的数据可视化库,例如Matplotlib、Seab


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
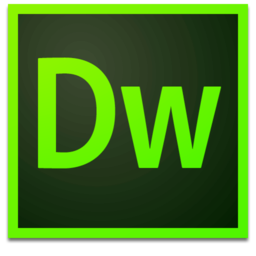
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
