How to implement custom error handling in Workerman documents
How to implement custom error handling in Workerman documents requires specific code examples
Workerman is a high-performance PHP asynchronous network communication framework, widely used in real-time In scenarios such as push and real-time interaction. In the process of using Workerman, we sometimes need to customize errors to improve the robustness and fault tolerance of the code. This article will detail how to implement custom error handling in Workerman and provide specific code examples.
1. The Importance of Error Handling
Error handling is an important part of ensuring the stable operation of the system. Normally, we use try...catch statements to catch and handle exceptions; but in the Workerman framework, we cannot use try...catch to catch exceptions. Therefore, we need to customize the error handling mechanism to handle abnormal situations and ensure the normal operation of the system.
2. Custom error handling method
Workerman provides a global error handling function register_shutdown_function, which can capture errors that occur during execution after the PHP parser parses the current script. We can customize error handling logic in this function.
The specific steps are as follows:
- Before the Worker starts, register the global error handling function register_shutdown_function.
require_once __DIR__ . '/vendor/autoload.php'; use WorkermanWorker; // 创建Worker对象 $worker = new Worker('tcp://0.0.0.0:2345'); // 设置错误处理函数 register_shutdown_function('customErrorHandler'); // Worker启动逻辑 $worker->onWorkerStart = function($worker) { // do something }; // 运行Worker Worker::runAll(); // 自定义错误处理函数 function customErrorHandler() { // 自定义错误处理逻辑 }
- In the custom error handling function, write the error handling logic. Operations such as logging and alarm notifications can be performed based on actual needs.
function customErrorHandler() { // 获取错误信息 $error = error_get_last(); // 判断是否存在错误信息 if ($error && ($error['type'] & (E_ERROR | E_PARSE | E_CORE_ERROR | E_COMPILE_ERROR))) { // 记录错误日志 error_log(date('Y-m-d H:i:s') . ' ' . $error['message'] . ' in ' . $error['file'] . ' on line ' . $error['line'] . PHP_EOL, 3, '/path/to/error.log'); // 发送告警通知 // sendAlert('Workerman Error', $error['message']); } }
In the above code, we use the error_get_last function to get the last error information. Then, we determine the error level based on the error type. If the error level is one of E_ERROR, E_PARSE, E_CORE_ERROR, and E_COMPILE_ERROR, it is considered a fatal error and needs to be processed. We can record the error information into a log file to facilitate future troubleshooting and analysis; at the same time, we can also send alarm notifications to promptly notify relevant personnel for processing.
3. Code example description
In the above code example, we used the Worker class, register_shutdown_function function and error_get_last function.
- The Worker class is the core of the Workerman framework, used to create Worker objects, set Worker startup logic, and run Worker.
- The register_shutdown_function function is a global error handling function provided by PHP, which is used to capture errors after the PHP parser parses the current script. We customize error handling logic in this function.
- The error_get_last function is used to get the last error information.
4. Summary
Custom error handling is an important part of ensuring stable operation of the system. In Workerman, we can use the register_shutdown_function function to customize error handling logic. By properly handling error messages, we can improve the robustness and fault tolerance of the code and ensure the normal operation of the system.
The above is a detailed introduction on how to implement custom error handling in Workerman documents, as well as corresponding code examples. I hope it will help you with error handling when using Workerman. Happy coding!
The above is the detailed content of How to implement custom error handling in Workerman documents. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
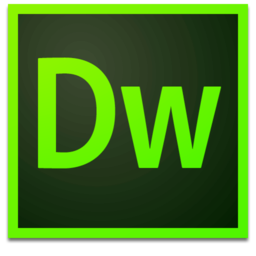
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Notepad++7.3.1
Easy-to-use and free code editor
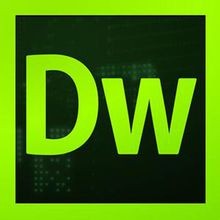
Dreamweaver CS6
Visual web development tools
