How to use Node.js to implement web-based real-time chat function
With the rapid development of the Internet, real-time communication functions have become a must-have feature for many websites and applications. As a lightweight, efficient, event-driven asynchronous I/O JavaScript running environment, Node.js can quickly build high-performance real-time applications, so it has become the best choice for developing real-time communication functions. This article will introduce in detail how to use Node.js to implement web-based real-time chat function and provide specific code examples.
First of all, we need to build a basic web server, which can be implemented using the http module that comes with Node.js. The specific code is as follows:
const http = require('http'); const server = http.createServer(); server.listen(3000, () => { console.log('服务器已启动,监听端口:3000'); });
Next, we need to use the socket.io module to implement the real-time communication function. Socket.io is a real-time communication library based on the WebSocket protocol and compatible with multiple transmission methods. It supports two-way communication between the client and the server, and is very suitable for real-time chat scenarios. The command to install the socket.io module is as follows:
npm install socket.io
After the installation is completed, we can use the socket.io module on the server side to enable real-time communication functionality. The specific code is as follows:
const http = require('http'); const server = http.createServer(); const io = require('socket.io')(server); io.on('connection', (socket) => { console.log('有一个用户已连接'); socket.on('disconnect', () => { console.log('有一个用户已断开连接'); }); socket.on('chat message', (msg) => { console.log('收到一条新消息:' + msg); io.emit('chat message', msg); }); }); server.listen(3000, () => { console.log('服务器已启动,监听端口:3000'); });
In the above code, we listen to the connection event of socket.io, which means it will be triggered when a user connects. When a user connects, we will output a prompt message on the console. Next, we listened to the disconnect event of socket.io, which means it will be triggered when the user disconnects. When a user disconnects, we will also output a prompt message on the console. Finally, we listened to the custom chat message event, which indicates the receipt of new messages from users. When new messages arrive, we broadcast them to all online users.
On the client side, we need to introduce the socket.io-client module to connect to the server and realize the display and sending of real-time chat. The specific code is as follows:
<!DOCTYPE html> <html> <head> <title>实时聊天</title> <script src="https://cdn.socket.io/socket.io-3.0.1.min.js"></script> <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> <script> $(function() { var socket = io(); $('form').submit(function(e) { e.preventDefault(); // 阻止表单提交 var msg = $('#m').val(); socket.emit('chat message', msg); // 发送消息到服务器 $('#m').val(''); return false; }); socket.on('chat message', function(msg) { // 收到新消息 $('#messages').append($('<li>').text(msg)); }); }); </script> </head> <body> <ul id="messages"></ul> <form> <input type="text" id="m" autocomplete="off" /> <button>发送</button> </form> </body> </html>
In the above code, we introduced the socket.io-client and jQuery modules. After the page is loaded, we create a connection with the server and listen to the chat message event, which means it will be triggered when a new message is received. When a new message arrives, we add it to the list of messages on the page. At the same time, when the user enters text in the input box and clicks the send button, we send the message to the server.
In summary, we have implemented the real-time chat function based on the WebSocket protocol by using Node.js and socket.io modules. In actual projects, we can also extend data such as persistent storage, identity authentication, and message push to provide richer real-time communication services.
The above is the detailed content of How to use Node.js to implement web-based real-time chat function. For more information, please follow other related articles on the PHP Chinese website!

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
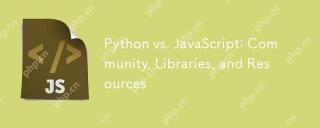
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
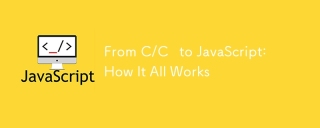
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
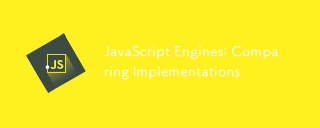
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
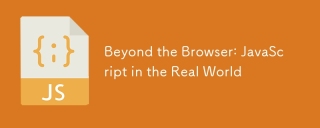
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
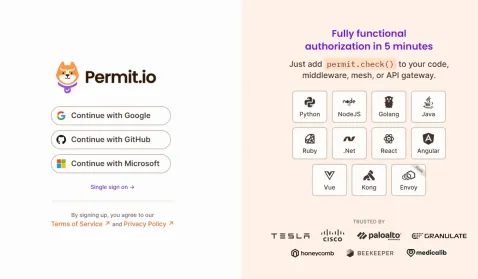
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
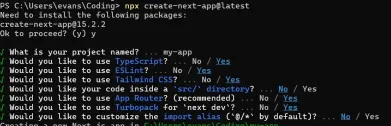
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
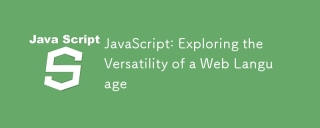
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
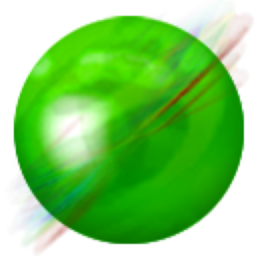
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor