Redis is an open source cache, key-value store and messaging system. It was invented by Salvatore Sanfilippo in 2009 and has gradually become one of the most commonly used caching and data storage solutions in web applications.
Redis provides a variety of data structures, including strings, hashes, lists, sets and ordered sets. These data structures have excellent features such as fast read/write performance, persistent storage, and cluster support. They can be used to cache response data in web applications, store session data, queue messages, etc.
The following will introduce how to use Redis to implement caching functions to improve application performance, and provide specific code examples.
- Initialize Redis connection
Before using Redis, you need to establish a connection with the corresponding driver library. Taking Python as an example, you can use the redis-py library:
import redis r = redis.Redis(host='localhost', port=6379, db=0)
In this example, we connect to a locally running Redis server, using the default port and the 0th database.
- Set cache data
Before writing data to the application's cache, the data needs to be serialized first. Redis supports multiple serialization methods, including string, JSON, pickle, etc.
The following is an example of writing the string "Hello, Redis Cache" to the cache:
import json data = 'Hello, Redis Cache' key = 'mykey' serialized_data = json.dumps(data) r.set(key, serialized_data)
This code converts the string data into JSON format and uses the Redis SET command to write it to In cache.
- Get cached data
Getting cached data from Redis is also a common operation. You can use the GET command to read the data in the cache and deserialize the data.
The following is an example of using the GET command to obtain cached data:
import json key = 'mykey' serialized_data = r.get(key) data = json.loads(serialized_data)
This code uses the Redis GET command to read the cached data with the key 'mykey'. Then, deserialize the data into a Python dictionary or other data type.
- Set the cache expiration time
When setting the cached data, you can also set the life cycle of the data. You can use the Redis EXPIRE command to set the cache expiration time. Once the cached data expires, Redis will automatically delete it.
The following is a sample code that sets the life cycle of the data to 60 seconds:
import json data = {'name': 'Tom', 'age': 30} key = 'user_001' serialized_data = json.dumps(data) r.set(key, serialized_data) r.expire(key, 60)
This code sets up a cached data named 'user_001' and sets the life cycle to 60 seconds. Afterwards, Redis will automatically delete this cached data.
- Use caching to improve application performance
Caching data can improve the performance of web applications, especially when the application needs to access the same data frequently. By writing data to the cache, applications can avoid querying the database multiple times, thereby reducing network latency and system load.
The following is an example of using caching to improve performance:
import time import json def get_user_data(user_id): key = 'user_' + str(user_id) serialized_data = r.get(key) if serialized_data is not None: # 缓存中有数据,直接读取并返回 data = json.loads(serialized_data) return data else: # 缓存中无数据,从数据库中读取并写入缓存 data = read_from_db(user_id) serialize_data = json.dumps(data) r.set(key, serialized_data) r.expire(key, 60) return data def read_from_db(user_id): # 从数据库读取用户数据 time.sleep(2) # 模拟真实数据库查询时间 data = {'name': 'Tom', 'age': 30} return data
This code simulates a function that reads user data. If there is user data in the cache, the function will read directly from the cache and return the data; otherwise, the function will read the user data from the database and write it to the Redis cache.
- Summary
The above introduces how Redis implements caching functions to improve the performance of web applications. It provides excellent features such as data storage, persistence, cluster support and multiple data structures, which can help developers easily build efficient applications.
When using Redis for caching, you need to pay attention to issues such as data serialization, cache expiration time, cache breakdown and cache avalanche. But these problems can be easily solved with some technical means and best practices.
We believe these tips and best practices will be helpful to you when using Redis caching to improve web application performance.
The above is the detailed content of How Redis implements caching function to improve application performance. For more information, please follow other related articles on the PHP Chinese website!
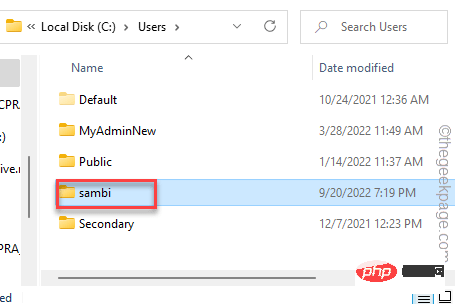
团队在Outlook中有一个非常有用的加载项,当您在使用Outlook2013或更高版本的应用程序时安装以前的应用程序时,它会自动安装。安装这两个应用程序后,只需打开Outlook,您就可以找到预装的加载项。但是,一些用户报告了在Outlook中找不到Team插件的异常情况。修复1–重新注册DLL文件有时需要重新注册特定的Teams加载项dll文件。第1步-找到MICROSOFT.TEAMS.ADDINLOADER.DLL文件1.首先,您必须确保
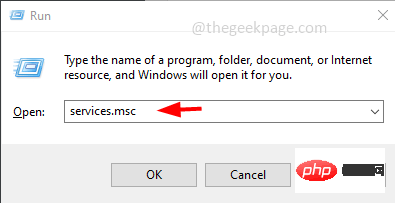
地址解析协议 (ARP) 用于将 MAC 地址映射到 IP 地址。网络上的所有主机都有自己的 IP 地址,但网络接口卡 (NIC) 将有 MAC 地址而不是 IP 地址。ARP 是用于将 IP 地址与 MAC 地址相关联的协议。所有这些条目都被收集并放置在 ARP 缓存中。映射的地址存储在缓存中,它们通常不会造成任何损害。但是,如果条目不正确或 ARP 缓存损坏,则会出现连接问题、加载问题或错误。因此,您需要清除 ARP 缓存并修复错误。在本文中,我们将研究如何清除 ARP 缓存的不同方法。方法

根据几位Windows10和Windows11用户的说法,他们在尝试安装Windows更新时遇到了错误0x80070246。此错误阻止他们升级PC并享受最新功能。值得庆幸的是,在本指南中,我们列出了一些最佳解决方案,可帮助您解决Windows0PC上80070246x11的Windows更新安装错误。我们还将首先讨论可能引发问题的原因。让我们直接进入它。为什么我会收到Windows更新安装错误0x80070246?您可能有多种原因导致您在PC上收到Windows11安装错误0x80070246。
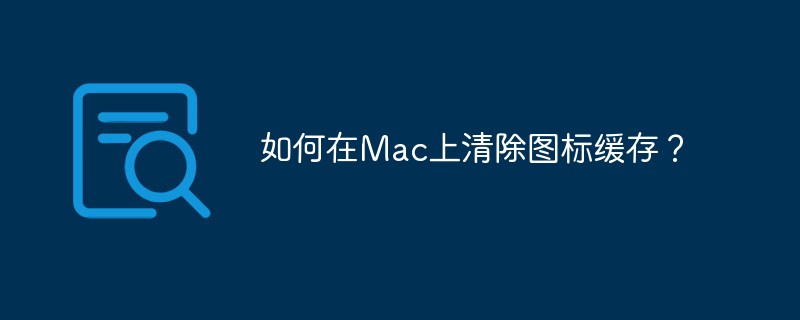
如何在Mac上清除和重置图标缓存警告:因为您将使用终端和rm命令,所以在继续执行任何操作之前,最好使用TimeMachine或您选择的备份方法备份您的Mac。输入错误的命令可能会导致永久性数据丢失,因此请务必使用准确的语法。如果您对命令行不满意,最好完全避免这种情况。启动终端并输入以下命令并按回车键:sudorm-rfv/Library/Caches/com.apple.iconservices.store接下来,输入以下命令并按回车键:sudofind/private/var
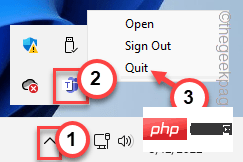
尝试在其设备上启动 Microsoft Teams 桌面客户端的用户在空白应用页面中报告了错误代码 caa70004。错误代码说:“我们很抱歉——我们遇到了问题。”以及重新启动 Microsoft Teams 以解决问题的选项。您可以尝试实施许多解决方案并再次加入会议。解决方法——1. 您应该尝试的第一件事是重新启动 Teams 应用程序。只需在错误页面上点击“重新启动”即可。
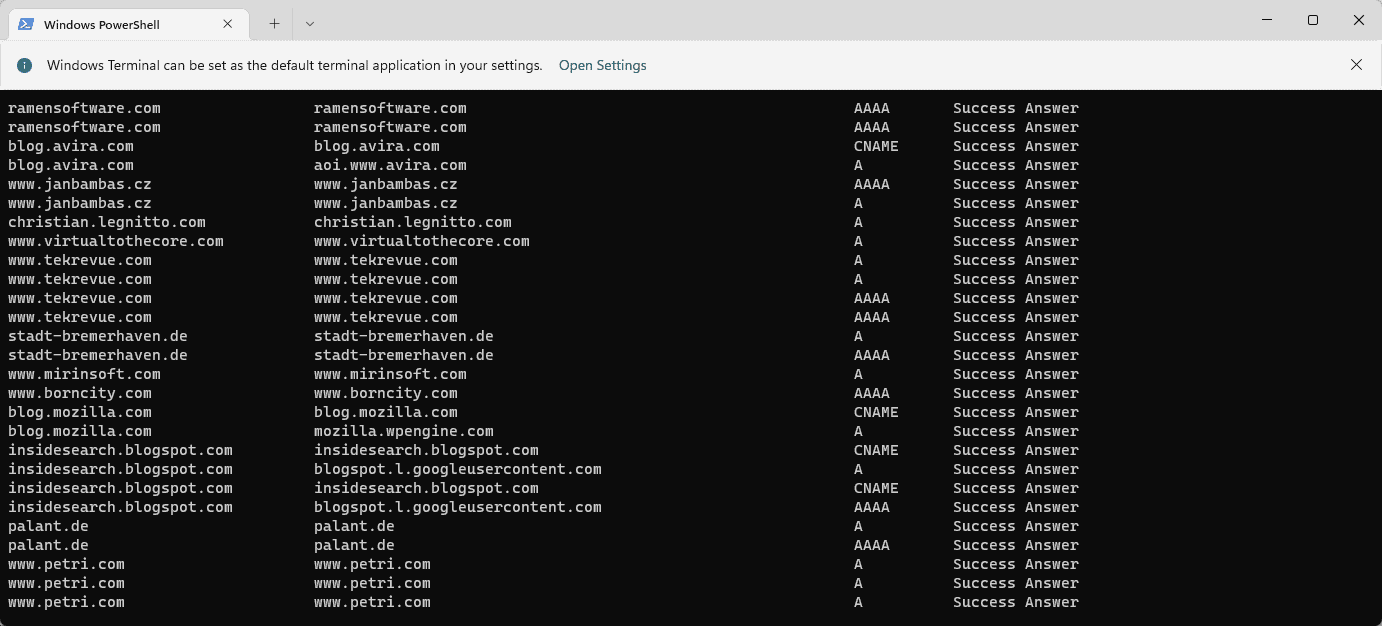
Windows操作系统使用缓存来存储DNS条目。DNS(域名系统)是用于通信的互联网核心技术。特别是用于查找域名的IP地址。当用户在浏览器中键入域名时,加载站点时执行的首要任务之一是查找其IP地址。该过程需要访问DNS服务器。通常,互联网服务提供商的DNS服务器会自动使用,但管理员可能会切换到其他DNS服务器,因为这些服务器可能更快或提供更好的隐私。如果DNS用于阻止对某些站点的访问,则切换DNS提供商也可能有助于绕过Internet审查。Windows使用DNS解
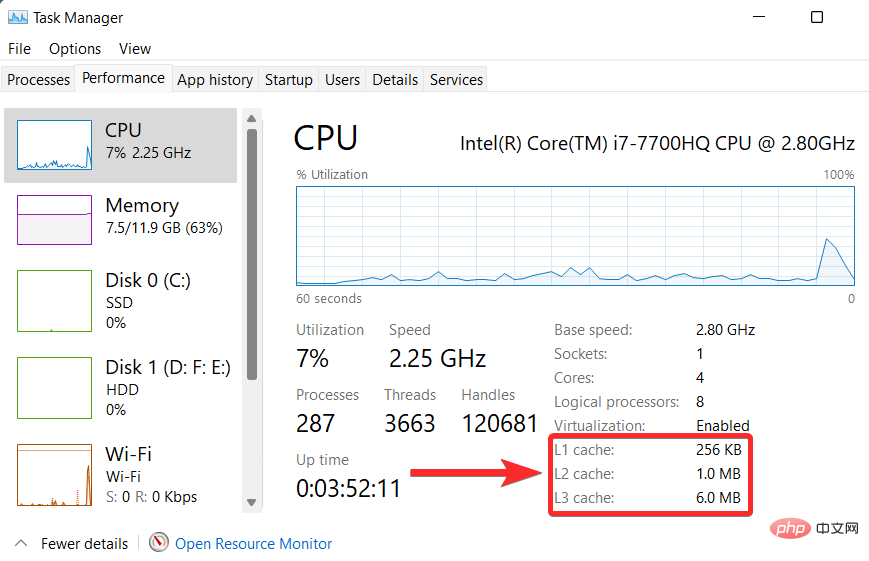
什么是缓存?缓存(发音为ka·shay)是一种专门的高速硬件或软件组件,用于存储经常请求的数据和指令,这些数据和指令又可用于更快地加载网站、应用程序、服务和系统的其他部分。缓存使最常访问的数据随时可用。缓存文件与缓存内存不同。缓存文件是指经常需要的文件,如PNG、图标、徽标、着色器等,多个程序可能需要这些文件。这些文件存储在您的物理驱动器空间中,通常是隐藏的。另一方面,高速缓存内存是一种比主内存和/或RAM更快的内存类型。它极大地减少了数据访问时间,因为与RAM相比,它更靠近CPU并且速度
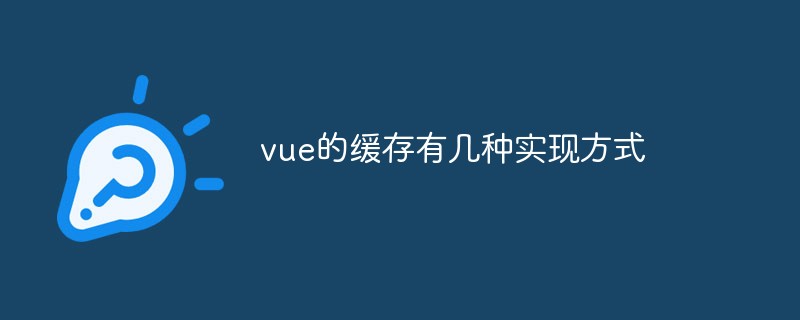
vue缓存数据有4种方式:1、利用localStorage,语法“localStorage.setItem(key,value)”;2、利用sessionStorage,语法“sessionStorage.setItem(key,value)”;3、安装并引用storage.js插件,利用该插件进行缓存;4、利用vuex,它是一个专为Vue.js应用程序开发的状态管理模式。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
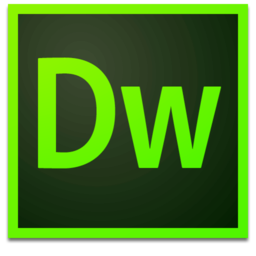
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
