How to use Laravel to implement data encryption and decryption functions
How to use Laravel to implement data encryption and decryption functions
Overview:
In modern Internet applications, protecting the security of user data is very important. One of the important security measures is the encrypted storage of sensitive data. Laravel, as a popular PHP framework, provides support for various encryption and decryption functions. This article will introduce how to use Laravel's encryption library to encrypt and decrypt data.
Step 1: Install Laravel
First, make sure Laravel is installed locally or on the server. If it is not installed yet, please follow the instructions in Laravel official documentation to install it. (https://laravel.com/docs)
Step 2: Configure the encryption key
Laravel uses the AES-256-CBC encryption algorithm by default. Before use, an encryption key needs to be generated and configured in the application's configuration file.
Open the config/app.php
file, find the 'key' => env('APP_KEY')
configuration item, and change env('APP_KEY')
Change it to a random string of length 32, such as: 'key' => 'YourRandomlyGeneratedKey'
, and save the configuration file.
Step 3: Use encryption and decryption functions
-
Encrypt data
Where the data needs to be encrypted, use theencrypt
function to encrypt the data encryption. For example:$encryptedData = encrypt('Sensitive Data');
encrypt
function will return the encrypted string. -
Decrypt data
For encrypted data, we can use thedecrypt
function to decrypt it. For example:$decryptedData = decrypt($encryptedData);
decrypt
function will return the decrypted original data.
Note:
- When using Laravel's encryption and decryption functions, you need to pay attention to the fact that the keys used for encryption and decryption must be consistent. If the application's key changes, the decryption operation will fail.
- It is not recommended to hardcode encryption keys in the code. The key can be stored in the application's environment variable and accessed using
env('APP_KEY')
.
Sample code:
The following example demonstrates how to use Laravel's encryption and decryption functions:
use IlluminateSupportFacadesCrypt; class UserController extends Controller { public function store(Request $request) { $encryptedData = Crypt::encrypt($request->input('sensitive_data')); // 存储加密后的数据到数据库或其他存储介质 return response()->json(['message' => 'Data encrypted successfully']); } public function show($id) { $encryptedData = DB::table('users')->select('encrypted_data')->where('id', $id)->first()->encrypted_data; $decryptedData = Crypt::decrypt($encryptedData); return response()->json(['data' => $decryptedData]); } }
The above example code demonstrates how to use encryption and decryption in a Laravel controller Decryption function:
- In the
store
method, receive the sensitive data in the request and use theCrypt::encrypt
function to encrypt the data, and then The encrypted data is stored in the database. - In the
show
method, obtain the encrypted data from the database and use theCrypt::decrypt
function to decrypt it, and finally the decrypted data Returned as a response.
Summary:
This article introduces how to use Laravel's encryption library to implement data encryption and decryption functions. By configuring the key and using the encrypt
and decrypt
functions, we can easily add data encryption functionality to our Laravel application to improve the security of user data.
The above is the detailed content of How to use Laravel to implement data encryption and decryption functions. For more information, please follow other related articles on the PHP Chinese website!

Laravel optimizes the web development process including: 1. Use the routing system to manage the URL structure; 2. Use the Blade template engine to simplify view development; 3. Handle time-consuming tasks through queues; 4. Use EloquentORM to simplify database operations; 5. Follow best practices to improve code quality and maintainability.

Laravel is a modern PHP framework that provides a powerful tool set, simplifies development processes and improves maintainability and scalability of code. 1) EloquentORM simplifies database operations; 2) Blade template engine makes front-end development intuitive; 3) Artisan command line tools improve development efficiency; 4) Performance optimization includes using EagerLoading, caching mechanism, following MVC architecture, queue processing and writing test cases.

Laravel's MVC architecture improves the structure and maintainability of the code through models, views, and controllers for separation of data logic, presentation and business processing. 1) The model processes data, 2) The view is responsible for display, 3) The controller processes user input and business logic. This architecture allows developers to focus on business logic and avoid falling into the quagmire of code.

Laravel is a PHP framework based on MVC architecture, with concise syntax, powerful command line tools, convenient data operation and flexible template engine. 1. Elegant syntax and easy-to-use API make development quick and easy to use. 2. Artisan command line tool simplifies code generation and database management. 3.EloquentORM makes data operation intuitive and simple. 4. The Blade template engine supports advanced view logic.

Laravel is suitable for building backend services because it provides elegant syntax, rich functionality and strong community support. 1) Laravel is based on the MVC architecture, simplifying the development process. 2) It contains EloquentORM, optimizes database operations. 3) Laravel's ecosystem provides tools such as Artisan, Blade and routing systems to improve development efficiency.
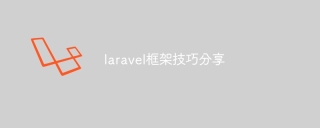
In this era of continuous technological advancement, mastering advanced frameworks is crucial for modern programmers. This article will help you improve your development skills by sharing little-known techniques in the Laravel framework. Known for its elegant syntax and a wide range of features, this article will dig into its powerful features and provide practical tips and tricks to help you create efficient and maintainable web applications.
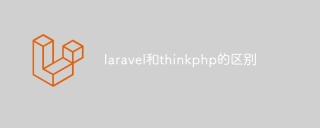
Laravel and ThinkPHP are both popular PHP frameworks and have their own advantages and disadvantages in development. This article will compare the two in depth, highlighting their architecture, features, and performance differences to help developers make informed choices based on their specific project needs.
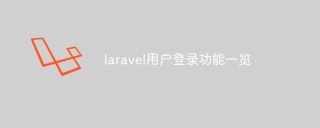
Building user login capabilities in Laravel is a crucial task and this article will provide a comprehensive overview covering every critical step from user registration to login verification. We will dive into the power of Laravel’s built-in verification capabilities and guide you through customizing and extending the login process to suit specific needs. By following these step-by-step instructions, you can create a secure and reliable login system that provides a seamless access experience for users of your Laravel application.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
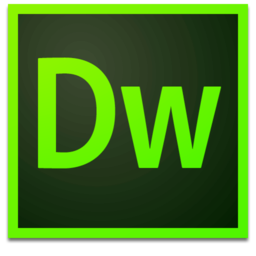
Dreamweaver Mac version
Visual web development tools
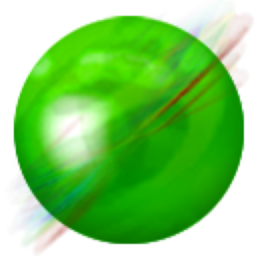
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
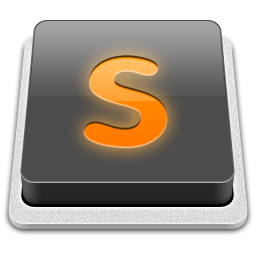
SublimeText3 Mac version
God-level code editing software (SublimeText3)