


Interpretation of Java documentation: Detailed description of the equals() method of the Arrays class
Interpretation of Java documentation: Detailed description of the equals() method of the Arrays class
In Java, the Arrays class is a static method that provides a series of methods for operating arrays Utility class. One of them is the equals() method. This article will explain the equals() method of the Arrays class in detail and provide specific code examples.
The equals() method of the Arrays class is used to compare whether two arrays are equal. This method has the following signature:
public static boolean equals(datatype[] a, datatype[] b)
where datatype represents the data type stored in the array. This method compares the elements in the two arrays to see if they are equal, and returns a Boolean value indicating whether they are equal. If the lengths and values of each element of the two arrays are equal, the equals() method returns true; otherwise, it returns false.
The following is a specific usage example:
import java.util.Arrays; public class ArrayComparison { public static void main(String[] args) { int[] array1 = {1, 2, 3, 4, 5}; int[] array2 = {1, 2, 3, 4, 5}; int[] array3 = {1, 2, 3, 4, 6}; System.out.println(Arrays.equals(array1, array2)); // 输出 true System.out.println(Arrays.equals(array1, array3)); // 输出 false } }
In the above example, we defined three arrays array1, array2 and array3. Among them, the elements of array1 and array2 are exactly the same, but the last element of array3 is different. Then we call the Arrays.equals() method respectively to compare these arrays. The results output true and false, indicating that the equals() method can correctly compare whether the elements of the two arrays are equal.
It should be noted that the equals() method is not suitable for multi-dimensional arrays. If you need to compare multi-dimensional arrays for equality, you can use the Arrays.deepEquals() method.
The following is a specific usage example:
import java.util.Arrays; public class MultiDimensionalArrayComparison { public static void main(String[] args) { int[][] array1 = {{1, 2}, {3, 4}}; int[][] array2 = {{1, 2}, {3, 4}}; int[][] array3 = {{1, 2}, {3, 5}}; System.out.println(Arrays.deepEquals(array1, array2)); // 输出 true System.out.println(Arrays.deepEquals(array1, array3)); // 输出 false } }
In the above example, we defined three two-dimensional arrays array1, array2 and array3. Call the Arrays.deepEquals() method individually to compare these arrays. The results output true and false, indicating that the deepEquals() method can correctly compare whether the elements of multi-dimensional arrays are equal.
Summary:
Java's Arrays class provides the equals() method for comparing whether two arrays are equal. This method compares the lengths of two arrays and the values of each element to see if they are equal in one-to-one correspondence. It should be noted that the equals() method is not applicable to multi-dimensional arrays. You can use the deepEquals() method to compare the equality of multi-dimensional arrays. By using these methods, you can easily compare the contents of arrays.
The above is the Java documentation interpretation: detailed description and code examples of the equals() method of the Arrays class. I hope it will be helpful to readers in understanding and using the equals() method.
The above is the detailed content of Interpretation of Java documentation: Detailed description of the equals() method of the Arrays class. For more information, please follow other related articles on the PHP Chinese website!
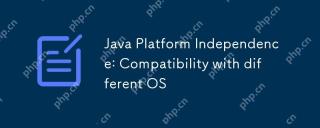
JavaachievesplatformindependencethroughtheJavaVirtualMachine(JVM),allowingcodetorunondifferentoperatingsystemswithoutmodification.TheJVMcompilesJavacodeintoplatform-independentbytecode,whichittheninterpretsandexecutesonthespecificOS,abstractingawayOS
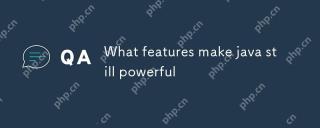
Javaispowerfulduetoitsplatformindependence,object-orientednature,richstandardlibrary,performancecapabilities,andstrongsecurityfeatures.1)PlatformindependenceallowsapplicationstorunonanydevicesupportingJava.2)Object-orientedprogrammingpromotesmodulara
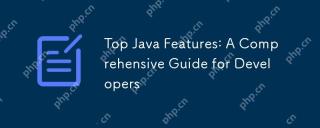
The top Java functions include: 1) object-oriented programming, supporting polymorphism, improving code flexibility and maintainability; 2) exception handling mechanism, improving code robustness through try-catch-finally blocks; 3) garbage collection, simplifying memory management; 4) generics, enhancing type safety; 5) ambda expressions and functional programming to make the code more concise and expressive; 6) rich standard libraries, providing optimized data structures and algorithms.
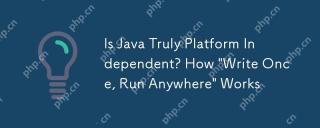
JavaisnotentirelyplatformindependentduetoJVMvariationsandnativecodeintegration,butitlargelyupholdsitsWORApromise.1)JavacompilestobytecoderunbytheJVM,allowingcross-platformexecution.2)However,eachplatformrequiresaspecificJVM,anddifferencesinJVMimpleme
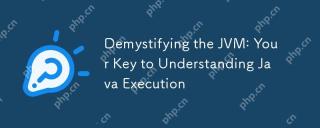
TheJavaVirtualMachine(JVM)isanabstractcomputingmachinecrucialforJavaexecutionasitrunsJavabytecode,enablingthe"writeonce,runanywhere"capability.TheJVM'skeycomponentsinclude:1)ClassLoader,whichloads,links,andinitializesclasses;2)RuntimeDataAr
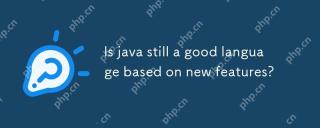
Javaremainsagoodlanguageduetoitscontinuousevolutionandrobustecosystem.1)Lambdaexpressionsenhancecodereadabilityandenablefunctionalprogramming.2)Streamsallowforefficientdataprocessing,particularlywithlargedatasets.3)ThemodularsystemintroducedinJava9im
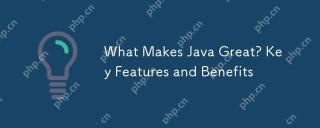
Javaisgreatduetoitsplatformindependence,robustOOPsupport,extensivelibraries,andstrongcommunity.1)PlatformindependenceviaJVMallowscodetorunonvariousplatforms.2)OOPfeatureslikeencapsulation,inheritance,andpolymorphismenablemodularandscalablecode.3)Rich
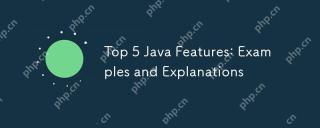
The five major features of Java are polymorphism, Lambda expressions, StreamsAPI, generics and exception handling. 1. Polymorphism allows objects of different classes to be used as objects of common base classes. 2. Lambda expressions make the code more concise, especially suitable for handling collections and streams. 3.StreamsAPI efficiently processes large data sets and supports declarative operations. 4. Generics provide type safety and reusability, and type errors are caught during compilation. 5. Exception handling helps handle errors elegantly and write reliable software.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
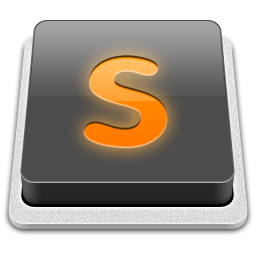
SublimeText3 Mac version
God-level code editing software (SublimeText3)
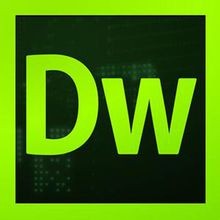
Dreamweaver CS6
Visual web development tools
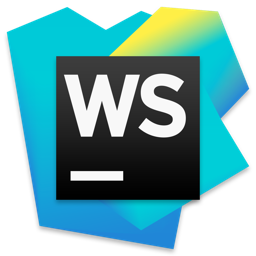
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
