Use JavaScript functions to autofill and validate forms
JavaScript function implementation for form automatic filling and validation
In web development, forms are a very common element, and we often need to fill and validate them. . Using JavaScript functions can easily realize the automatic filling and verification functions of the form, improving user experience and data accuracy. In this article, I will introduce how to use JavaScript functions to implement form autofill and validation, and provide specific code examples.
1. Auto-fill form
Auto-fill form can reduce the tedious manual input and improve efficiency when users fill in the form. Usually, we can store the user's information in advance, such as name, email, phone number, etc., and then automatically fill this information into the corresponding form when the user visits the web page.
The sample code is as follows:
// 假设用户信息存储在一个对象中 var userInfo = { name: "张三", email: "zhangsan@example.com", phone: "123456789" }; // 自动填充表单 function autoFillForm() { document.getElementById("name").value = userInfo.name; document.getElementById("email").value = userInfo.email; document.getElementById("phone").value = userInfo.phone; } // 在页面加载完成后调用自动填充函数 window.onload = function() { autoFillForm(); };
In the above code, we first define an object userInfo to store user information, which contains the values of name, email and phone number. Then through the autofill form function autoFillForm, we can assign these values to the value attribute of the corresponding form element to achieve the automatic filling effect. Finally, just call the autoFillForm function after the page is loaded.
2. Form verification
Form verification is to ensure that the information filled in by users conforms to certain rules or formats to prevent malicious submissions or data errors. Common form verification includes: required field verification, email format verification, mobile phone number format verification, etc.
The sample code is as follows:
// 表单验证 function formValidation() { var name = document.getElementById("name").value; var email = document.getElementById("email").value; var phone = document.getElementById("phone").value; // 验证姓名是否为空 if (name === "") { alert("请输入姓名!"); return false; } // 验证邮箱格式 var emailPattern = /^w+([-+.]w+)*@w+([-.]w+)*.w+([-.]w+)*$/; if (!emailPattern.test(email)) { alert("请输入有效的邮箱地址!"); return false; } // 验证手机号格式 var phonePattern = /^1[3456789]d{9}$/; if (!phonePattern.test(phone)) { alert("请输入有效的手机号码!"); return false; } // 验证通过,可提交表单 alert("提交成功!"); return true; }
In the above code, we obtain the value of each input box in the form and apply regular expressions for corresponding verification. If the verification fails, a prompt message will pop up and false will be returned, preventing form submission; if the verification passes, a prompt message indicating successful submission will pop up and return true, allowing the form to be submitted.
To use the form validation function, you can add an onclick event on the submit button of the form, as follows:
<form> <label for="name">姓名:</label> <input type="text" id="name" required> <label for="email">邮箱:</label> <input type="email" id="email" required> <label for="phone">电话:</label> <input type="tel" id="phone" required> <input type="submit" value="提交" onclick="return formValidation()"> </form>
In the above code, we called the formValidation function in the onclick event of the submit button , and use the return keyword to receive the return value of the function. If true is returned, form submission is allowed; if false is returned, form submission is prevented.
Summary:
By using JavaScript functions to realize automatic filling and verification of forms, we can make it more convenient and accurate for users to fill in forms. The above code provides a specific implementation of automatic form filling and form validation, which can be modified and expanded according to actual needs. Hope this article helps you!
The above is the detailed content of Use JavaScript functions to autofill and validate forms. For more information, please follow other related articles on the PHP Chinese website!
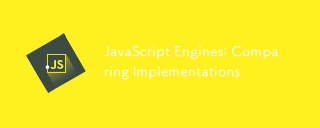
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
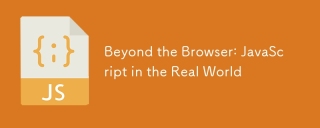
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
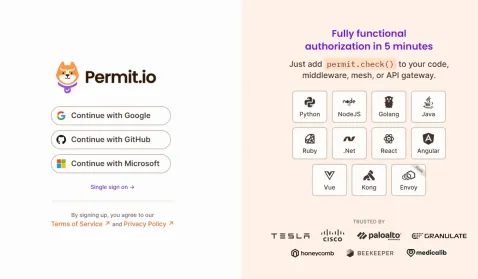
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
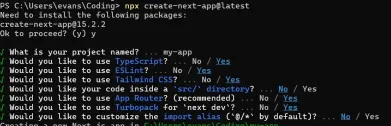
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
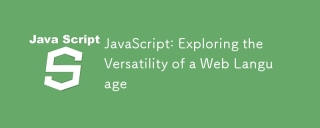
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
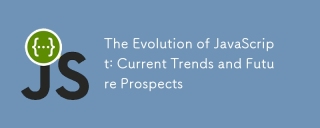
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
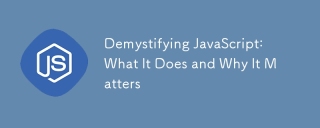
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
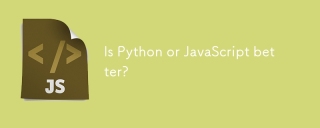
Python is more suitable for data science and machine learning, while JavaScript is more suitable for front-end and full-stack development. 1. Python is known for its concise syntax and rich library ecosystem, and is suitable for data analysis and web development. 2. JavaScript is the core of front-end development. Node.js supports server-side programming and is suitable for full-stack development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
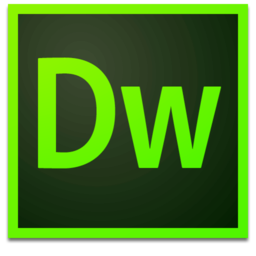
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.