


Array_walk() function in PHP: How to execute a callback function for each element in the array
array_walk() function in PHP: how to execute a callback function for each element in the array
In PHP, array is a commonly used data structure. It is often necessary to perform specific operations on each element in an array. The array_walk() function can help us achieve this goal. This article will introduce the usage of array_walk() function and give some specific code examples.
- array_walk() function introduction
The array_walk() function is a built-in array function in PHP, which is used to execute the specified callback function for each element in the array. The syntax is as follows:
array_walk(array &$array, callable $callback [, mixed $userdata = NULL])
Parameter description:
- array &$ array: required. The array to be operated on.
- callable $callback: required. Callback function used to perform processing operations on each element in the array.
- mixed $userdata: Optional. User data passed to the callback function.
The array_walk() function iterates through each element in the array and applies the callback function to each element.
- Application examples of array_walk() function
The following are some specific code examples to illustrate the usage of array_walk() function:
(1 ) Add the prefix "Hello, " to each element in the array:
<?php function addPrefix(&$value, $key) { $value = "Hello, " . $value; } $fruits = array("Apple", "Banana", "Cherry"); array_walk($fruits, 'addPrefix'); // 输出修改后的数组 print_r($fruits); ?>
Output result:
Array ( [0] => Hello, Apple [1] => Hello, Banana [2] => Hello, Cherry )
(2) Use the callback function to implement the sum of array elements:
<?php function sumElements(&$value, $key, $userdata) { $value += $userdata; } $numbers = array(1, 2, 3, 4, 5); $sum = 0; array_walk($numbers, 'sumElements', $sum); // 输出求和结果 echo $sum; ?>
Output results:
15
By passing different callback functions to the array_walk() function, we can perform different operations on each element in the array according to specific needs.
- Notes
When using the array_walk() function, you need to pay attention to the following points:
(1) Pay attention to the parameters of the callback function.
- If the callback function does not need to change the value of the array element, the first parameter of the callback function can be defined in the form of &$value to avoid the overhead of copying the array element.
- If you want the callback function to change the value of the array element, define the first parameter of the callback function in the form of &$value, and define the first parameter of the array_walk() function in the form of pass-in (& $array).
(2) The return value of the callback function will not affect the return value of the array_walk() function.
The return value of the callback function can exist, but it will not affect the return value of the array_walk() function. The return value of the array_walk() function is a Boolean type, indicating whether the execution was successful.
(3) The array_walk() function does not support associative arrays.
The array_walk() function is only valid for index arrays and cannot be used normally for associative arrays. If you need to process each value of an associative array, it is recommended to use the array_walk_assoc() function.
Summary
The array_walk() function is a convenient array operation function in PHP, which can be used to perform customized processing operations on each element in the array. By passing different callback functions, we can perform specific operations on the elements in the array according to our needs. I hope the introduction and examples in this article can help you become familiar with and use the array_walk() function.
The above is the detailed content of Array_walk() function in PHP: How to execute a callback function for each element in the array. For more information, please follow other related articles on the PHP Chinese website!
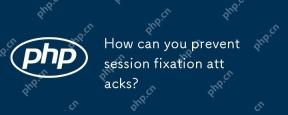
Effective methods to prevent session fixed attacks include: 1. Regenerate the session ID after the user logs in; 2. Use a secure session ID generation algorithm; 3. Implement the session timeout mechanism; 4. Encrypt session data using HTTPS. These measures can ensure that the application is indestructible when facing session fixed attacks.
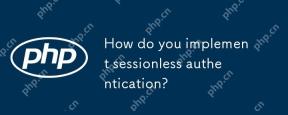
Implementing session-free authentication can be achieved by using JSONWebTokens (JWT), a token-based authentication system where all necessary information is stored in the token without server-side session storage. 1) Use JWT to generate and verify tokens, 2) Ensure that HTTPS is used to prevent tokens from being intercepted, 3) Securely store tokens on the client side, 4) Verify tokens on the server side to prevent tampering, 5) Implement token revocation mechanisms, such as using short-term access tokens and long-term refresh tokens.
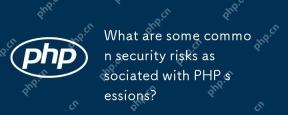
The security risks of PHP sessions mainly include session hijacking, session fixation, session prediction and session poisoning. 1. Session hijacking can be prevented by using HTTPS and protecting cookies. 2. Session fixation can be avoided by regenerating the session ID before the user logs in. 3. Session prediction needs to ensure the randomness and unpredictability of session IDs. 4. Session poisoning can be prevented by verifying and filtering session data.
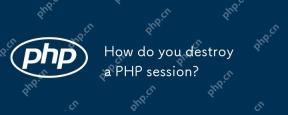
To destroy a PHP session, you need to start the session first, then clear the data and destroy the session file. 1. Use session_start() to start the session. 2. Use session_unset() to clear the session data. 3. Finally, use session_destroy() to destroy the session file to ensure data security and resource release.
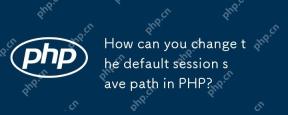
How to change the default session saving path of PHP? It can be achieved through the following steps: use session_save_path('/var/www/sessions');session_start(); in PHP scripts to set the session saving path. Set session.save_path="/var/www/sessions" in the php.ini file to change the session saving path globally. Use Memcached or Redis to store session data, such as ini_set('session.save_handler','memcached'); ini_set(
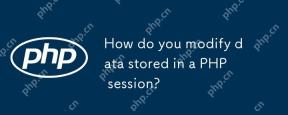
TomodifydatainaPHPsession,startthesessionwithsession_start(),thenuse$_SESSIONtoset,modify,orremovevariables.1)Startthesession.2)Setormodifysessionvariablesusing$_SESSION.3)Removevariableswithunset().4)Clearallvariableswithsession_unset().5)Destroythe
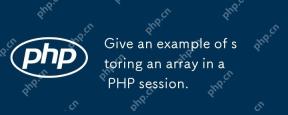
Arrays can be stored in PHP sessions. 1. Start the session and use session_start(). 2. Create an array and store it in $_SESSION. 3. Retrieve the array through $_SESSION. 4. Optimize session data to improve performance.
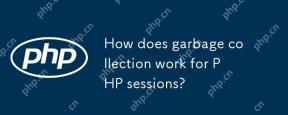
PHP session garbage collection is triggered through a probability mechanism to clean up expired session data. 1) Set the trigger probability and session life cycle in the configuration file; 2) You can use cron tasks to optimize high-load applications; 3) You need to balance the garbage collection frequency and performance to avoid data loss.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
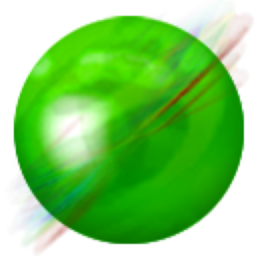
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
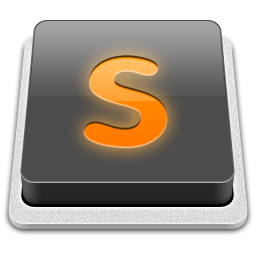
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version
