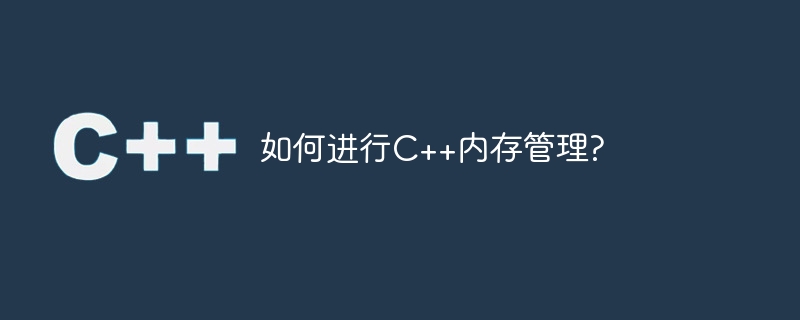
How to perform C memory management?
C is a powerful programming language, but it also requires developers to be responsible for memory management. In C, memory management is very important, because wrong memory usage can lead to memory leaks, wild pointers and a series of other problems. Therefore, it is crucial for C developers to master good memory management skills.
Memory in C is divided into two types: stack memory and heap memory. Stack memory is automatically managed by the compiler and is used to store local variables as well as function return addresses and other related information. The heap memory is manually managed by developers and is used to store dynamically allocated objects. The following are some basic principles and techniques of C memory management.
- Use new and delete operators
In C, use the new operator to dynamically allocate memory, and use the delete operator to release memory. For example:
int* p = new int; // Allocate an integer memory space
*p = 5; // Store 5 in memory
delete p; // Release Memory space
Note that when you use the new operator to allocate memory, you must use the delete operator to release the memory. Otherwise, memory leaks will result.
- Be careful when using arrays
When you need to dynamically allocate an array, you can use the new[] operator to allocate memory and the delete[] operator to release memory. For example:
#int* arr = new int[5]; // Allocate an array containing 5 integers
delete[] arr; // Release memory space
Likewise, when you use the new[] operator to allocate memory, you must use the delete[] operator to free the memory. Otherwise, memory leaks will also occur.
- Using smart pointers
C 11 introduced the concept of smart pointers, which are a tool for automatically managing memory. Smart pointers can ensure that the memory space occupied by an object is automatically released when the object is no longer used. There are two commonly used smart pointers in C: shared_ptr and unique_ptr. For example:
shared_ptr p(new int); // Allocate an integer memory space and use shared_ptr to manage it
*p = 5; // Store 5# in memory
##When using smart pointers, you do not need to explicitly call the delete operator to release memory. When the reference count of a smart pointer reaches 0, it will automatically release the memory space of the managed object.
Avoid memory leaks- A memory leak means that the allocated memory space is not released correctly during the execution of the program, causing this part of the memory to no longer be used by the program. In order to avoid memory leaks, you should develop a good habit of actively releasing memory. When an object is no longer needed, delete or a smart pointer should be called promptly to release the memory.
Also, avoid repeatedly allocating and freeing memory in a loop. If you need to frequently allocate and release memory in a loop, you can consider moving the memory allocation code outside the loop, which can improve the efficiency of the program.
Prevent wild pointers- Wild pointers refer to pointers pointing to released memory. When you release a certain memory space, the address of that space is no longer valid, but if you still hold a pointer to that address, it will cause a wild pointer problem. To avoid wild pointers, you should set the pointer to nullptr after freeing the memory, or assign the pointer to another valid address.
Summary
Memory management in C is an important task, which directly affects the performance and stability of the program. Memory in C can be better managed by using new and delete operators, smart pointers, and avoiding memory leaks and wild pointers. By mastering these techniques, you can write more efficient and stable C programs.
The above is the detailed content of How to perform C++ memory management?. For more information, please follow other related articles on the PHP Chinese website!