How to use Java to write a simple student leave approval result query system?
With the development of education, students asking for leave has become an indispensable part of every school management. In order to facilitate school administrators and students to understand the leave situation, this article will introduce how to use Java to write a simple student leave approval result query system.
First, we need to create a student class (Student), which contains attributes such as the student's name, student number, date of leave, reason for leave, and status of leave. You can use the following code to create a student class:
public class Student { private String name; // 学生姓名 private int id; // 学号 private String leaveDate; // 请假日期 private String leaveReason; // 请假事由 private String leaveStatus; // 请假状态 // 构造函数 public Student(String name, int id, String leaveDate, String leaveReason, String leaveStatus) { this.name = name; this.id = id; this.leaveDate = leaveDate; this.leaveReason = leaveReason; this.leaveStatus = leaveStatus; } // getter和setter方法 // ... }
Next, we need to create a leave management system class (LeaveManagementSystem) to manage students' leave information. You can use the following code to create a leave management system class:
import java.util.ArrayList; import java.util.List; public class LeaveManagementSystem { private List<Student> studentList; // 学生列表 public LeaveManagementSystem() { studentList = new ArrayList<>(); } // 添加学生请假信息 public void addStudentLeave(Student student) { studentList.add(student); } // 根据学号查询学生请假信息 public Student searchStudentLeave(int id) { for (Student student : studentList) { if (student.getId() == id) { return student; } } return null; } // 查询所有学生请假信息 public List<Student> getAllStudentLeave() { return studentList; } }
In the main function, we can create a LeaveManagementSystem object and perform corresponding operations. The following is a simple example:
public class Main { public static void main(String[] args) { LeaveManagementSystem system = new LeaveManagementSystem(); // 添加学生请假信息 system.addStudentLeave(new Student("张三", 1001, "2021-05-01", "生病", "已批准")); system.addStudentLeave(new Student("李四", 1002, "2021-05-02", "参加比赛", "已批准")); // 根据学号查询学生请假信息 Student student = system.searchStudentLeave(1001); if (student != null) { System.out.println("学生姓名:" + student.getName()); System.out.println("学号:" + student.getId()); System.out.println("请假日期:" + student.getLeaveDate()); System.out.println("请假事由:" + student.getLeaveReason()); System.out.println("请假状态:" + student.getLeaveStatus()); } // 查询所有学生请假信息 List<Student> studentList = system.getAllStudentLeave(); for (Student s : studentList) { System.out.println("学生姓名:" + s.getName()); System.out.println("学号:" + s.getId()); System.out.println("请假日期:" + s.getLeaveDate()); System.out.println("请假事由:" + s.getLeaveReason()); System.out.println("请假状态:" + s.getLeaveStatus()); } } }
Through the above code, we have implemented a simple student leave approval result query system. Users can add students' leave information, query the leave information of specific students based on their student numbers, or view the leave information of all students.
Of course, this is just a simple example, and the actual leave management system may require more functions and verifications. But through this simple example, we can understand how to use Java to write a student leave approval result query system, and it can be expanded and optimized according to the actual situation.
The above is the detailed content of How to use Java to write a simple student leave approval result query system?. For more information, please follow other related articles on the PHP Chinese website!
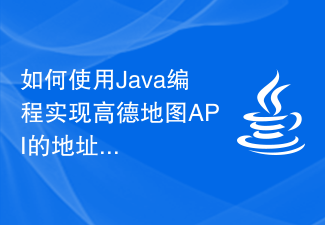
如何使用Java编程实现高德地图API的地址位置附近搜索引言:高德地图是一款颇为受欢迎的地图服务,广泛应用于各类应用程序中。其中,地址位置附近搜索功能提供了搜索附近POI(PointofInterest,兴趣点)的能力。本文将详细讲解如何使用Java编程实现高德地图API的地址位置附近搜索功能,通过代码示例帮助读者了解和掌握相关技术。一、申请高德地图开发
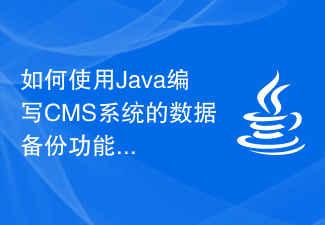
如何使用Java编写CMS系统的数据备份功能在一个内容管理系统(ContentManagementSystem,CMS)中,数据备份是一个非常重要且必不可少的功能。通过数据备份,我们可以保证系统中的数据在遭受损坏、丢失或错误操作等情况下能够及时恢复,从而确保系统的稳定性和可靠性。本文将介绍如何使用Java编写CMS系统的数据备份功能,并提供相关的代码示
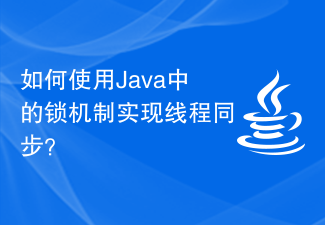
如何使用Java中的锁机制实现线程同步?在多线程编程中,线程同步是一个非常重要的概念。当多个线程同时访问和修改共享资源时,可能会导致数据不一致或竞态条件的问题。Java提供了锁机制来解决这些问题,并确保线程安全的访问共享资源。Java中的锁机制由synchronized关键字和Lock接口提供。接下来,我们将学习如何使用这两种机制来实现线程同步。使用sync
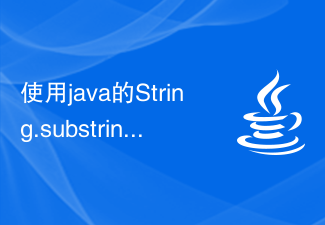
使用java的String.substring()函数截取字符串的子串在Java编程语言中,String类提供了用于操作字符串的丰富方法。其中,String.substring()函数是一个常用的方法,可以用于截取字符串的子串。本文将介绍如何使用String.substring()函数进行字符串截取,并提供一些实际应用场景的代码示例。String.subst
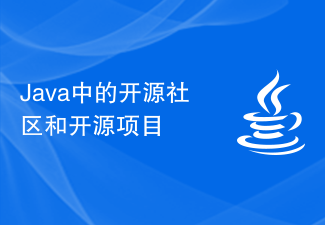
Java是一门广受欢迎的编程语言,其大量的开源社区和项目为Java编程提供了许多帮助。开源社区和项目的重要性越来越被人们所认识,本文将介绍Java开源社区和项目的概念、重要性以及一些流行的开源项目和社区。开源社区和项目是什么?简单地说,开源社区和项目是一群开发者利用开放源代码来共同开发软件的组织。这些项目通常基于一些开源软件许可证来授权,允许开发者
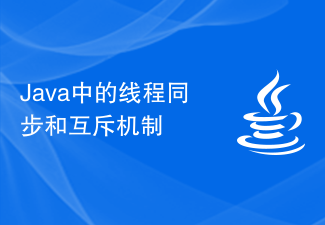
Java中的线程同步和互斥机制在Java中,多线程是一个重要的技术。要高效地并发执行多个任务,需要掌握线程之间的同步和协作机制。本文将介绍Java中的线程同步和互斥机制。线程同步线程同步指的是多个线程在执行过程中,通过合作来完成指定的任务。多个线程执行的代码段互斥地访问共享资源,在执行完一段代码后,只有一个线程能够访问共享资源,其他线程需要等待。线程同步遵循
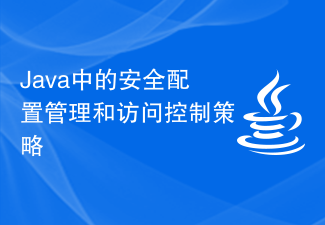
Java中的安全配置管理和访问控制策略在Java应用程序开发中,安全性是一个至关重要的方面。为了保护应用程序免受潜在的攻击,我们需要实施一系列的安全配置管理和访问控制策略。本文将探讨Java中的安全配置管理和访问控制策略,并提供一些相关的代码示例。安全配置管理安全配置管理是指在Java应用程序中设置和管理各种安全机制和策略,以确保应用程序的安全性。Java提
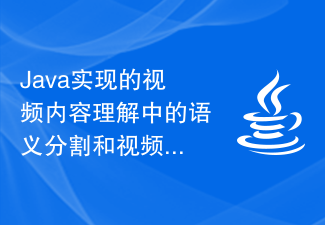
在现如今的数字视频时代,视频内容理解技术在各个领域中起着重要的作用,如视频推荐、视频搜索、视频自动标注等。其中,语义分割和视频概念检测技术是视频内容理解的两个主要方面。本文将从Java实现的角度出发,介绍语义分割和视频概念检测技术的基本概念及其在实际应用中的价值。一、语义分割技术语义分割技术是计算机视觉领域的一个重要研究方向,其目的是对图像或视频进行像素级别


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
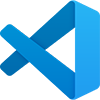
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
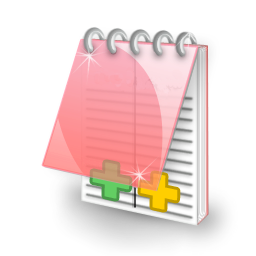
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Zend Studio 13.0.1
Powerful PHP integrated development environment
