How to use middleware for error handling in Laravel
How to use middleware for error handling in Laravel
Introduction:
Laravel is a popular PHP framework with a powerful error handling system. Among them, middleware is one of the core functions of Laravel. It can handle errors by executing a series of logic before or after the request enters routing processing. This article will detail how to use middleware for error handling in Laravel and provide specific code examples.
1. Create error handling middleware
First, we need to create a custom middleware to handle errors. In Laravel, you can create middleware through the following command:
php artisan make:middleware HandleErrors
This command will create a file named # in the app/Http/Middleware
directory. ##HandleErrors middleware. Next, we need to add our error handling logic in the
handle method of the middleware. The following is a simple sample code:
<?php namespace AppHttpMiddleware; use Closure; use Exception; use IlluminateHttpRequest; class HandleErrors { public function handle(Request $request, Closure $next) { try { return $next($request); } catch (Exception $exception) { // 在这里处理错误 } } }In the above code, we have used a
try-catch block to catch exceptions that may occur. Once an exception is caught, we can perform corresponding error handling operations in the
catch block.
In Laravel, the middleware needs to be registered in the global middleware stack of the application. This can be accomplished by following these steps:
- Open the
- app/Http/Kernel.php
file.
Add the following line of code in the - $middleware
array to register the middleware:
'error.handler' => AppHttpMiddlewareHandleErrors::class,
To apply error handling middleware to a specific route or routing group, you can use Laravel's
route method or
groupmethod. Here is an example:
Route::group(['middleware' => 'error.handler'], function () { // 在这里定义需要应用错误处理中间件的路由 });In the above code example, we used the
group method to create a routing group with error handling middleware. Routes defined within this group will automatically have error handling middleware applied.
Now that we have set up the error handling middleware, we will show how to handle errors in the middleware.
UserController, which contains a
create method for creating users. The following is a sample code:
namespace AppHttpControllers; use IlluminateHttpRequest; class UserController extends Controller { public function create(Request $request) { // 创建用户的逻辑 throw new Exception('创建用户失败'); } }In the above code, we deliberately threw an exception to simulate the occurrence of an error. Now, we need to catch the exception in the error handling middleware and handle it accordingly. The following is a simple sample code:
namespace AppHttpMiddleware; use Closure; use Exception; use IlluminateHttpRequest; use IlluminateSupportFacadesLog; class HandleErrors { public function handle(Request $request, Closure $next) { try { return $next($request); } catch (Exception $exception) { Log::error($exception->getMessage()); return response()->json(['error' => '发生了一个错误,请稍后再试'], 500); } } }In the above code, we use the
Log class to record error information to the log file and return a JSON response containing the error information .
Through the above steps, we successfully used middleware for error handling. First, we created a custom middleware to handle errors and registered it with the middleware stack. We then applied the middleware to a specific route or route group and implemented specific logic for error handling. In actual development, the logic and implementation of error handling can be customized as needed.
The above is the detailed content of How to use middleware for error handling in Laravel. For more information, please follow other related articles on the PHP Chinese website!

Tocombatisolationandlonelinessinremotework,companiesshouldimplementregular,meaningfulinteractions,provideequalgrowthopportunities,andusetechnologyeffectively.1)Fostergenuineconnectionsthroughvirtualcoffeebreaksandpersonalsharing.2)Ensureremoteworkers

Laravelispopularforfull-stackdevelopmentbecauseitoffersaseamlessblendofbackendpowerandfrontendflexibility.1)Itsbackendcapabilities,likeEloquentORM,simplifydatabaseinteractions.2)TheBladetemplatingengineallowsforclean,dynamicHTMLtemplates.3)LaravelMix

Key factors in choosing a video conferencing platform include user interface, security, and functionality. 1) The user interface should be intuitive, such as Zoom. 2) Security needs to be paid attention to, and Microsoft Teams provides end-to-end encryption. 3) Functions need to match requirements, GoogleMeet is suitable for short meetings, and CiscoWebex provides advanced collaboration tools.

The latest version of Laravel10 is compatible with MySQL 5.7 and above, PostgreSQL 9.6 and above, SQLite 3.8.8 and above, SQLServer 2017 and above. These versions are chosen because they support Laravel's ORM features, such as the JSON data type of MySQL5.7, which improves query and storage efficiency.

Laravelisanexcellentchoiceforfull-stackdevelopmentduetoitsrobustfeaturesandeaseofuse.1)ItsimplifiescomplextaskswithitsmodernPHPsyntaxandtoolslikeBladeforfront-endandEloquentORMforback-end.2)Laravel'secosystem,includingLaravelMixandArtisan,enhancespro

Laravel10,releasedonFebruary7,2023,isthelatestversion.Itfeatures:1)Improvederrorhandlingwithanewreportmethodintheexceptionhandler,2)EnhancedsupportforPHP8.1featureslikeenums,and3)AnewLaravel\Promptspackageforinteractivecommand-lineprompts.

ThelatestLaravelversionenhancesdevelopmentwith:1)Simplifiedroutingusingimplicitmodelbinding,2)EnhancedEloquentcapabilitieswithnewquerymethods,and3)ImprovedsupportformodernPHPfeatureslikenamedarguments,makingcodingmoreefficientandenjoyable.

You can find the release notes for the latest Laravel version at laravel.com/docs. 1) Release Notes provide detailed information on new features, bug fixes and improvements. 2) They contain examples and explanations to help understand the application of new features. 3) Pay attention to the potential complexity and backward compatibility issues of new features. 4) Regular review of release notes can keep it updated and inspire innovation.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
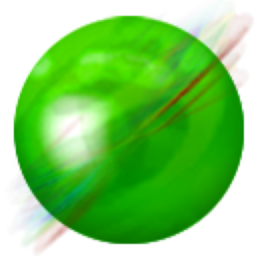
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
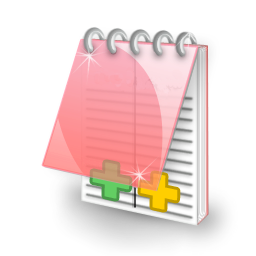
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Atom editor mac version download
The most popular open source editor
