


Practical Guide to Laravel Permission Function: How to Implement User Permission Approval Process
Practical Guide for Laravel Permission Function: How to implement the user permission approval process, specific code examples are required
Introduction:
In today's rapid development of the Internet In this era, the management of system permissions has become more and more important. As a popular PHP development framework, Laravel provides a set of simple and powerful permission management functions that can help developers easily implement the user permission approval process. This article will introduce how to implement the user permission approval process in the Laravel framework and give specific code examples.
1. Basics of permission management
Before starting to implement the user permission approval process, we first need to understand the basic knowledge of permission management. In the Laravel framework, permission management usually includes three core concepts: role (Role), permission (Permission) and user (User).
Role: Role represents the different roles played by users in the system, and each role has certain permissions.
Permission: Permission represents the user's operation permissions in the system, such as adding, editing, deleting, etc.
User: A user is an individual used in the system. Each user can have one or more roles.
Through these three core concepts, we can build a flexible and scalable user rights management system.
2. Design of user permissions approval process
Before implementing the user permissions approval process, we need to clarify the design of the entire process. Usually, the user permission approval process includes the following steps:
- User submits permission application: The user submits a permission application to the system administrator, including the required roles and permissions.
- Administrator approves permission application: After receiving the user's permission application, the administrator can approve the application.
- Assign permissions: After approval, the administrator can assign the required roles and permissions to the user.
- Restrict permissions: For certain important operations or sensitive information, administrators can set additional permission restrictions.
Through the above process, users can apply for permissions according to their own needs, and administrators can approve the applications and allocate and restrict permissions as needed.
3. Implement the user permissions approval process based on the Laravel framework
Below we will implement the above user permissions approval process based on the Laravel framework. First, we need to install and configure the Laravel framework and create the necessary database tables. After this, we can follow the steps below to implement it.
- Create data migration files
Use Laravel's migration function to create the required data tables, including role tables, permission tables, user tables, etc. The command to create a migration file is as follows:
php artisan make:migration create_roles_table php artisan make:migration create_permissions_table php artisan make:migration create_users_table
Define the fields of the table in the migration file and write the migration logic. For example, the sample code of the role table migration file is as follows:
public function up() { Schema::create('roles', function (Blueprint $table) { $table->id(); $table->string('name'); $table->timestamps(); }); }
Executing the migration command will create the corresponding data table:
php artisan migrate
- Create model and association relationship
Create roles, permissions and user models, and establish relationships between them. In the Laravel framework, you can use Eloquent associations to define relationships between models. The sample code is as follows:
class Role extends Model { public function permissions() { return $this->belongsToMany(Permission::class); } } class Permission extends Model { public function roles() { return $this->belongsToMany(Role::class); } } class User extends Authenticatable { public function roles() { return $this->belongsToMany(Role::class); } }
- Implementing permission application and approval functions
Add methods related to permission approval in the user model, including submitting permission applications and approving permission applications. The sample code is as follows:
class User extends Authenticatable { // ... public function submitPermissionRequest($roles, $permissions) { // 创建权限申请记录,并关联角色和权限 $permissionRequest = PermissionRequest::create([ 'user_id' => $this->id, 'status' => 'pending', ]); $permissionRequest->roles()->sync($roles); $permissionRequest->permissions()->sync($permissions); } public function approvePermissionRequest($requestId) { // 审批权限申请,并分配角色和权限 $permissionRequest = PermissionRequest::findOrFail($requestId); $permissionRequest->update(['status' => 'approved']); $this->roles()->sync($permissionRequest->roles); $this->permissions()->sync($permissionRequest->permissions); } }
- Implementing permission restriction function
Add permission restriction middleware in routes and controllers to ensure that only users with corresponding permissions can access specific page or perform a specific action. The sample code is as follows:
// 在路由中使用中间件限制权限 Route::middleware('permission:edit')->group(function () { Route::get('/edit', 'UserController@edit'); Route::post('/edit', 'UserController@update'); }); // 在控制器中使用中间件限制权限 public function __construct() { $this->middleware('permission:edit')->only('edit', 'update'); }
Through the above steps, we successfully implemented the user permission approval process function. Users can submit permission applications as needed, administrators can approve and allocate applications, and the system can automatically limit users' permissions.
Conclusion:
Through the introduction of this article, we have learned the basic knowledge of permission management in the Laravel framework and implemented the function of the user permission approval process. Using the permission management functions provided by the Laravel framework, developers can quickly build a flexible and easily extensible permission management system. I hope this article will be helpful to everyone in using the Laravel framework for permission management.
Reference materials:
- Laravel official documentation: https://laravel.com/docs
- Laravel permission management detailed guide: https://learnku.com /docs/laravel/8.x/authorization/7944
- Laravel user rights management video tutorial: https://www.youtube.com/watch?v=GDgrdz2hgJc
Appendix : For complete code examples, please see the GitHub repository: https://github.com/example/laravel-permission
The above is the detailed content of Practical Guide to Laravel Permission Function: How to Implement User Permission Approval Process. For more information, please follow other related articles on the PHP Chinese website!

Laravel optimizes the web development process including: 1. Use the routing system to manage the URL structure; 2. Use the Blade template engine to simplify view development; 3. Handle time-consuming tasks through queues; 4. Use EloquentORM to simplify database operations; 5. Follow best practices to improve code quality and maintainability.

Laravel is a modern PHP framework that provides a powerful tool set, simplifies development processes and improves maintainability and scalability of code. 1) EloquentORM simplifies database operations; 2) Blade template engine makes front-end development intuitive; 3) Artisan command line tools improve development efficiency; 4) Performance optimization includes using EagerLoading, caching mechanism, following MVC architecture, queue processing and writing test cases.

Laravel's MVC architecture improves the structure and maintainability of the code through models, views, and controllers for separation of data logic, presentation and business processing. 1) The model processes data, 2) The view is responsible for display, 3) The controller processes user input and business logic. This architecture allows developers to focus on business logic and avoid falling into the quagmire of code.

Laravel is a PHP framework based on MVC architecture, with concise syntax, powerful command line tools, convenient data operation and flexible template engine. 1. Elegant syntax and easy-to-use API make development quick and easy to use. 2. Artisan command line tool simplifies code generation and database management. 3.EloquentORM makes data operation intuitive and simple. 4. The Blade template engine supports advanced view logic.

Laravel is suitable for building backend services because it provides elegant syntax, rich functionality and strong community support. 1) Laravel is based on the MVC architecture, simplifying the development process. 2) It contains EloquentORM, optimizes database operations. 3) Laravel's ecosystem provides tools such as Artisan, Blade and routing systems to improve development efficiency.
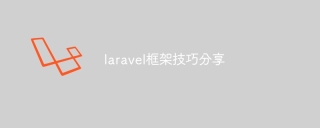
In this era of continuous technological advancement, mastering advanced frameworks is crucial for modern programmers. This article will help you improve your development skills by sharing little-known techniques in the Laravel framework. Known for its elegant syntax and a wide range of features, this article will dig into its powerful features and provide practical tips and tricks to help you create efficient and maintainable web applications.
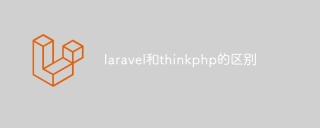
Laravel and ThinkPHP are both popular PHP frameworks and have their own advantages and disadvantages in development. This article will compare the two in depth, highlighting their architecture, features, and performance differences to help developers make informed choices based on their specific project needs.
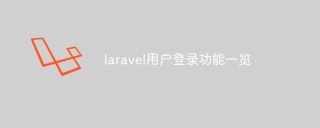
Building user login capabilities in Laravel is a crucial task and this article will provide a comprehensive overview covering every critical step from user registration to login verification. We will dive into the power of Laravel’s built-in verification capabilities and guide you through customizing and extending the login process to suit specific needs. By following these step-by-step instructions, you can create a secure and reliable login system that provides a seamless access experience for users of your Laravel application.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
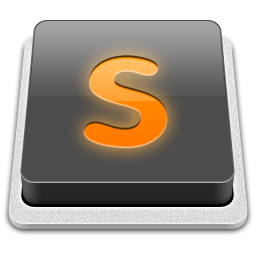
SublimeText3 Mac version
God-level code editing software (SublimeText3)
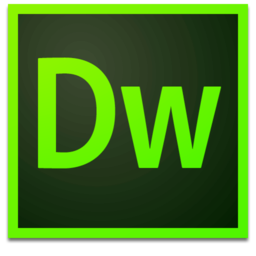
Dreamweaver Mac version
Visual web development tools
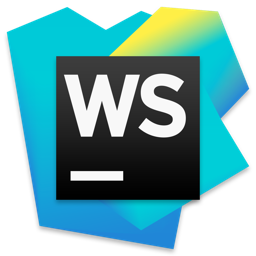
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment