How to use Laravel to implement data search and recommendation functions
How to use Laravel to implement data search and recommendation functions
Overview:
In modern applications, data search and recommendation functions are very important. Data search can help users quickly find the information they need in large amounts of data, while data recommendation can recommend relevant data based on users' interests and preferences. In this article, we will discuss how to implement these two functions using the Laravel framework and provide corresponding code examples.
- Implementation of data search function:
First, we need to create a database table containing a search field, such as a product table. This table can be created using Laravel's migration function, as shown below:
php artisan make:migration create_products_table --create=products
In the generated migration file, we can define the fields of the product table, such as name, description, price, etc. Implement it using the following code in the migration file:
public function up() { Schema::create('products', function (Blueprint $table) { $table->increments('id'); $table->string('name'); $table->text('description'); $table->decimal('price'); $table->timestamps(); }); }
Next, we need to create a controller to handle the logic of the search function. The controller can be generated using the following command:
php artisan make:controller ProductController
In the controller, we can implement a method called search to handle the search functionality. In this method, we will obtain the keywords entered by the user and query the data in the product table based on the keywords. The sample code is as follows:
public function search(Request $request) { $keyword = $request->input('keyword'); $products = Product::where('name', 'like', '%' . $keyword . '%') ->orWhere('description', 'like', '%' . $keyword . '%') ->get(); return view('products.search', ['products' => $products]); }
In the view file, we can display it based on the queried product data. For example, you can iterate through product data and display the name, description, and price of each product. The sample code is as follows:
@foreach($products as $product) <div> <h3 id="product-name">{{ $product->name }}</h3> <p>{{ $product->description }}</p> <p>Price: {{ $product->price }}</p> </div> @endforeach
- Implementation of data recommendation function:
The data recommendation function needs to recommend relevant data to users based on their interests and preferences. Before implementing this function, we need to create a database table that contains user interests and preferences, such as a user table. This table can be created using Laravel's migration functionality, similar to the previous steps.
When a user logs in or registers, we can collect the user's interest and preference data and store it in the user table. Next, we need to create a controller to handle the logic of the recommendation feature. The controller can be generated using the following command:
php artisan make:controller RecommendationController
In the controller, we can implement a method called recommend to handle the recommendation function. In this method, we will obtain the current user's interests and preferences, and query recommended products based on these data. The sample code is as follows:
public function recommend(Request $request) { $user = $request->user(); $products = Product::whereIn('category', $user->interests) ->orderBy('rating', 'desc') ->limit(5) ->get(); return view('products.recommend', ['products' => $products]); }
In the view file, we can display the recommended product data based on the query. The sample code is similar to the previous implementation.
Summary:
Through the Laravel framework, we can easily implement data search and recommendation functions. For data search, we need to create corresponding database tables and controllers, then query relevant data based on user input and display it in the view. For data recommendation, we need to collect user interest and preference data, and query and display recommended data based on this data. The above code examples hope to help readers better understand and use the Laravel framework to implement data search and recommendation functions.
The above is the detailed content of How to use Laravel to implement data search and recommendation functions. For more information, please follow other related articles on the PHP Chinese website!

Laravelcanbeeffectivelyusedinreal-worldapplicationsforbuildingscalablewebsolutions.1)ItsimplifiesCRUDoperationsinRESTfulAPIsusingEloquentORM.2)Laravel'secosystem,includingtoolslikeNova,enhancesdevelopment.3)Itaddressesperformancewithcachingsystems,en

Laravel's core functions in back-end development include routing system, EloquentORM, migration function, cache system and queue system. 1. The routing system simplifies URL mapping and improves code organization and maintenance. 2.EloquentORM provides object-oriented data operations to improve development efficiency. 3. The migration function manages the database structure through version control to ensure consistency. 4. The cache system reduces database queries and improves response speed. 5. The queue system effectively processes large-scale data, avoid blocking user requests, and improve overall performance.

Laravel performs strongly in back-end development, simplifying database operations through EloquentORM, controllers and service classes handle business logic, and providing queues, events and other functions. 1) EloquentORM maps database tables through the model to simplify query. 2) Business logic is processed in controllers and service classes to improve modularity and maintainability. 3) Other functions such as queue systems help to handle complex needs.

The Laravel development project was chosen because of its flexibility and power to suit the needs of different sizes and complexities. Laravel provides routing system, EloquentORM, Artisan command line and other functions, supporting the development of from simple blogs to complex enterprise-level systems.

The comparison between Laravel and Python in the development environment and ecosystem is as follows: 1. The development environment of Laravel is simple, only PHP and Composer are required. It provides a rich range of extension packages such as LaravelForge, but the extension package maintenance may not be timely. 2. The development environment of Python is also simple, only Python and pip are required. The ecosystem is huge and covers multiple fields, but version and dependency management may be complex.

How does Laravel play a role in backend logic? It simplifies and enhances backend development through routing systems, EloquentORM, authentication and authorization, event and listeners, and performance optimization. 1. The routing system allows the definition of URL structure and request processing logic. 2.EloquentORM simplifies database interaction. 3. The authentication and authorization system is convenient for user management. 4. The event and listener implement loosely coupled code structure. 5. Performance optimization improves application efficiency through caching and queueing.

Laravel's popularity includes its simplified development process, providing a pleasant development environment, and rich features. 1) It absorbs the design philosophy of RubyonRails, combining the flexibility of PHP. 2) Provide tools such as EloquentORM, Blade template engine, etc. to improve development efficiency. 3) Its MVC architecture and dependency injection mechanism make the code more modular and testable. 4) Provides powerful debugging tools and performance optimization methods such as caching systems and best practices.

Both Django and Laravel are full-stack frameworks. Django is suitable for Python developers and complex business logic, while Laravel is suitable for PHP developers and elegant syntax. 1.Django is based on Python and follows the "battery-complete" philosophy, suitable for rapid development and high concurrency. 2.Laravel is based on PHP, emphasizing the developer experience, and is suitable for small to medium-sized projects.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
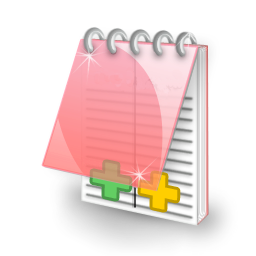
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
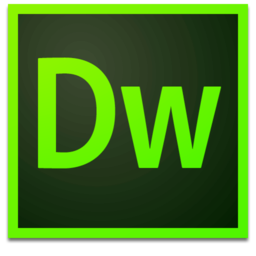
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor