


How to design the inventory table structure of a warehouse management system in MySQL?
How to design the inventory table structure of the warehouse management system in MySQL?
In the warehouse management system, inventory management is a very important part. In order to effectively manage the inventory of items in the warehouse, we need to design a reasonable inventory table structure in the MySQL database. This article explains how to structure an inventory table and provides some concrete code examples.
- Create a table
First, we need to create an inventory table to store item information in the warehouse. Here we assume that the items in the warehouse have the following attributes: item ID, item name, and item quantity.
CREATE TABLE inventory (
id INT PRIMARY KEY AUTO_INCREMENT, name VARCHAR(50) NOT NULL, quantity INT NOT NULL
);
- Insert data
Next, we can insert some data into the inventory table Test data for verification.
INSERT INTO inventory (name, quantity)
VALUES ('item A', 100),
('物品B', 200), ('物品C', 150);
- Query inventory
We can Use a simple SELECT statement to query item information in the inventory table.
SELECT * FROM inventory;
This will return all data in the inventory table, including item ID, item name and item quantity.
- Update Inventory
When the quantity of items in the warehouse changes, we need to update the data in the inventory table.
-- Increase the quantity of item A
UPDATE inventory SET quantity = quantity 50 WHERE name = 'Item A';
-- Reduce the quantity of item B
UPDATE inventory SET quantity = quantity - 20 WHERE name = 'item B';
- Delete item
If an item is no longer stored in the warehouse, we can use the DELETE statement Delete it from the inventory table.
DELETE FROM inventory WHERE name = 'item C';
- Increase index
In order to improve query performance, we can add the primary key and Add an index to the item name.
ALTER TABLE inventory ADD INDEX idx_id (id);
ALTER TABLE inventory ADD INDEX idx_name (name);
In this way, when we query based on the item ID or item name, the database The query speed will be greatly improved.
- Add trigger
In the warehouse management system, we may need to perform some additional operations in the inventory table, for example, when the inventory quantity is lower than a certain threshold Automatically send notifications.
CREATE TRIGGER low_inventory_trigger
AFTER UPDATE ON inventory FOR EACH ROW
BEGIN
IF NEW.quantity < 10 THEN -- 发送库存不足通知 INSERT INTO notifications (message) VALUES ('库存不足,请及时补充'); END IF;
END;
This trigger will be checked every time the inventory quantity is updated is below the threshold and inserts a notification message into the notification table.
Summary:
Designing the inventory table structure is an important part of the warehouse management system. Through reasonable table structure design, we can efficiently manage the inventory of items in the warehouse. In MySQL, we can use the CREATE TABLE statement to create an inventory table, use the INSERT statement to insert data, use the SELECT statement to query the inventory, use the UPDATE statement to update the inventory, and use the DELETE statement to delete items. In addition, we can also use indexes to improve query performance and perform some additional operations through triggers. The above is a simple example. In actual situations, it may need to be appropriately adjusted and optimized according to specific needs.
Code examples are for reference only, and the specific implementation will be adjusted accordingly according to actual needs and project architecture.
The above is the detailed content of How to design the inventory table structure of a warehouse management system in MySQL?. For more information, please follow other related articles on the PHP Chinese website!
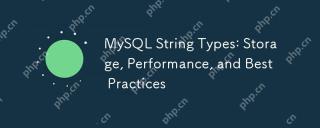
MySQLstringtypesimpactstorageandperformanceasfollows:1)CHARisfixed-length,alwaysusingthesamestoragespace,whichcanbefasterbutlessspace-efficient.2)VARCHARisvariable-length,morespace-efficientbutpotentiallyslower.3)TEXTisforlargetext,storedoutsiderows,
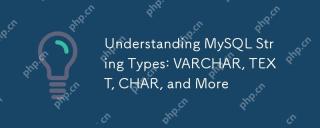
MySQLstringtypesincludeVARCHAR,TEXT,CHAR,ENUM,andSET.1)VARCHARisversatileforvariable-lengthstringsuptoaspecifiedlimit.2)TEXTisidealforlargetextstoragewithoutadefinedlength.3)CHARisfixed-length,suitableforconsistentdatalikecodes.4)ENUMenforcesdatainte
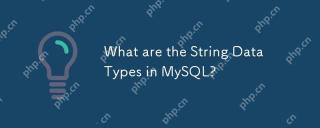
MySQLoffersvariousstringdatatypes:1)CHARforfixed-lengthstrings,2)VARCHARforvariable-lengthtext,3)BINARYandVARBINARYforbinarydata,4)BLOBandTEXTforlargedata,and5)ENUMandSETforcontrolledinput.Eachtypehasspecificusesandperformancecharacteristics,sochoose
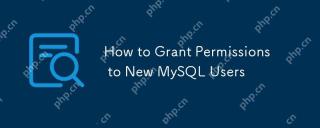
TograntpermissionstonewMySQLusers,followthesesteps:1)AccessMySQLasauserwithsufficientprivileges,2)CreateanewuserwiththeCREATEUSERcommand,3)UsetheGRANTcommandtospecifypermissionslikeSELECT,INSERT,UPDATE,orALLPRIVILEGESonspecificdatabasesortables,and4)
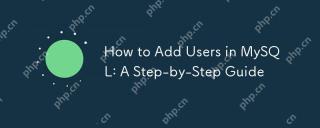
ToaddusersinMySQLeffectivelyandsecurely,followthesesteps:1)UsetheCREATEUSERstatementtoaddanewuser,specifyingthehostandastrongpassword.2)GrantnecessaryprivilegesusingtheGRANTstatement,adheringtotheprincipleofleastprivilege.3)Implementsecuritymeasuresl
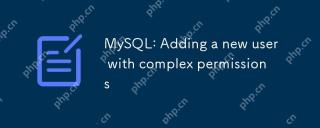
ToaddanewuserwithcomplexpermissionsinMySQL,followthesesteps:1)CreatetheuserwithCREATEUSER'newuser'@'localhost'IDENTIFIEDBY'password';.2)Grantreadaccesstoalltablesin'mydatabase'withGRANTSELECTONmydatabase.TO'newuser'@'localhost';.3)Grantwriteaccessto'
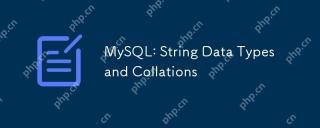
The string data types in MySQL include CHAR, VARCHAR, BINARY, VARBINARY, BLOB, and TEXT. The collations determine the comparison and sorting of strings. 1.CHAR is suitable for fixed-length strings, VARCHAR is suitable for variable-length strings. 2.BINARY and VARBINARY are used for binary data, and BLOB and TEXT are used for large object data. 3. Sorting rules such as utf8mb4_unicode_ci ignores upper and lower case and is suitable for user names; utf8mb4_bin is case sensitive and is suitable for fields that require precise comparison.
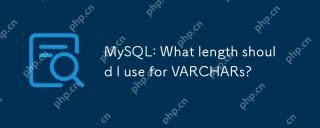
The best MySQLVARCHAR column length selection should be based on data analysis, consider future growth, evaluate performance impacts, and character set requirements. 1) Analyze the data to determine typical lengths; 2) Reserve future expansion space; 3) Pay attention to the impact of large lengths on performance; 4) Consider the impact of character sets on storage. Through these steps, the efficiency and scalability of the database can be optimized.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
